Introduction
The jQuery Tab Menu is a small and nice looking menu plugin which you can add to your web site very easily. It has built-in selection animation effect, customizable open trigger and optional open and selection callbacks. By default, the drop down menus are opened when the mouse enters a top-level anchor element, but you can configure the open behavior and make the drop downs open after a click.
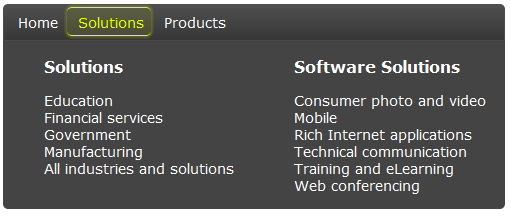
Using the Code
To show the jQuery Tab Menu, you need to add two .JS files. The first .JS file is the latest version of jQuery. The second file is the jqwidgetstabmenu.js which is the implementation of the plugin. After that, you need to add the style.css file which includes classes for styling the jQuery Tab Menu. The final step is to initialize the menu by using the jqwidgetstabmenu
function and pass a DIV
container element's id as parameter.
<script type="text/javascript"
src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js"></script>
<link rel="stylesheet" href="style.css" type="text/css" />
<script type="text/javascript" src="jqwidgetstabmenu.js"></script>
<script type="text/javascript">
$(document).ready(function () {
jqwidgetstabmenu("tabmenuid");
});
</script>
You can also pass an optional second parameter to the jqwidgetstabmenu
function which represents the open trigger, i.e., when the dropdown menu should be opened (after click, double-click or mouse-enter).
Below is a sample HTML markup for the Tab Menu:
<div id='tabmenuid'>
<ul>
<li><a href="#1">Home</a></li>
<li><a href="#2">Solutions</a></li>
<li><a href="#3">Products</a></li>
</ul>
<div class="container">
Home Container</div>
<div class="container">
<div style="float: left; width: 50%;">
<ul>
<li>
<h3>
Solutions</h3>
</li>
<li><a href="#">Education</a></li>
<li><a href="#">Financial services</a></li>
<li><a href="#">Government</a></li>
<li><a href="#">Manufacturing</a></li>
<li><a href="#">All industries and solutions</a></li>
</ul>
</div>
<div style="float: left; width: 50%;">
<ul>
<li>
<h3>
Software Solutions</h3>
</li>
<li><a href="#">Consumer photo and video</a></li>
<li><a href="#">Mobile</a></li>
</ul>
</div>
</div>
<div class="container">
<ul>
<li>
<h3>
Products</h3>
</li>
<li><a href="#">PC products</a></li>
<li><a href="#">Mobile products</a></li>
<li><a href="#">All products</a></li>
</ul>
</div>
</div>
In order to create the menu, you need to pass the DIV
container's id to the jqwidgetstabmenu
function and you need to set the class of the drop downs to "container
".
Working with the API:
trigger
The trigger member specifies when a drop down should be opened.
The following code configures the sub menus to be opened after click:
var tab = jqwidgetstabmenu("tabmenuid", "click");
opencallback
The opencallback
is called when a drop down is opened.
var tab = jqwidgetstabmenu("tabmenuid", "click");
selectioncallback
The selectioncallback
is called when the selected tab is changed.
var tab = jqwidgetstabmenu("tabmenuid");tab.selectioncallback = function (args)
{ var index = args.selected; var selectedanchor = args.anchor;}
closeall
closeall
function closes all drop downs.
var tab = jqwidgetstabmenu("tabmenuid");tab.closeall();
openmenu
openmenu
function opens a drop down by index.
var tab = jqwidgetstabmenu("tabmenuid");tab.openmenu(0);
Implementation
When we pass the id to the jqwidgetstabmenu
constructor, it tries to find the DIV
container by that id.
var menu = $("#" + tabmenu.menuid);
Then we find the top-level anchor tags and the DIV
elements with class 'container
'. Each top-level anchor tag should have an associated DIV
element with 'container
' class.
var anchors = $(menu).find('ul:first a');var submenus = $(menu).find('.container');
After that, we loop through the anchor
tags. Inside the loop, we need to create a wrapper around each container DIV
element. This wrapper is added to the document
's body
with absolute positioning. Inside the loop body, you will also see a function called 'move
'. The purpose of this function is to move a semi-transparent selection background when the user selects a tab and position it over the tab with smooth animation effect.
anchors.each(function (index) {
var $anchor = $(this)
var $submenu = $(submenus[index]);
$submenu.wrap('<div class="wrapper" style="z-index: 9999;
position:absolute;top:0;left:0;visibility:hidden">
<div style="position:absolute;overflow:hidden;left:0;top:0;width:100%;
height:100%;"></div></div>')
.css({ visibility: 'inherit', top: -$submenu.outerHeight() })
.data('timer', {})
var $wrapper = $submenu.closest('div.wrapper').css
({ width: $submenu.outerWidth() + offset[0], height: $submenu.outerHeight() +
offset[1] })
$wrapper.appendTo(document.body);
var move = function ($el) {
selection.show();
var dims = {
'left': $el.offset().left,
'top': $el.offset().top,
'width': $el.outerWidth(),
'height': $el.outerHeight()
};
$el.addClass('menu-item-selected');
selection.stop().animate(dims, 250, function () {
});
if (tabmenu.selectioncallback) {
tabmenu.selectioncallback({ selected: index, anchor: $anchor[0] });
}
};
tabmenu.submenus[index] = function () {
tabmenu.closeall();
var offset = menu.offset();
move($anchor.parents('li'));
$wrapper.css({ visibility: 'visible', left: offset.left,
top: offset.top + menu.outerHeight(), width: menu.outerWidth() })
$submenu.css('top', 0);
menu.removeClass('jqx-rc-all');
menu.addClass('jqx-rc-t');
if (tabmenu.opencallback) {
tabmenu.opencallback({ opened: index });
}
}
$anchor.bind(tabmenu.trigger, function (e) {
tabmenu.submenus[index]();
return false;
})
})
In the above code, we bind each anchor
tag to a trigger specified by the tabmenu.trigger
member. The value of this member is passed as second parameter to jqwidgetstabmenu
function. If you omit it, the default value is equal to 'mouseenter
', i.e., a drop down will be opened when the mouse cursor enters into a tab's bounds.
$anchor.bind(tabmenu.trigger, function (e) {
tabmenu.submenus[index]();
return false;
})
The following code shows a drop down menu below the Tab Menu. It shows the wrapper element and positions it below the menu's root container element.
tabmenu.submenus[index] = function () {
tabmenu.closeall();
var offset = menu.offset();
move($anchor.parents('li'));
$wrapper.css({ visibility: 'visible', left: offset.left, top:
offset.top + menu.outerHeight(), width: menu.outerWidth() })
$submenu.css('top', 0);
menu.removeClass('jqx-rc-all');
menu.addClass('jqx-rc-t');
if (tabmenu.opencallback) {
tabmenu.opencallback({ opened: index });
}
}
History
- 24th December, 2011: Initial post