The same-origin policy prevents a script loaded from one domain from getting or manipulating properties of a document from another domain. That is, the domain of the requested URL must be the same as the domain of the current Web page. This basically means that the browser isolates content from different origins to guard them against manipulation. JSONP (JSON with Padding) represents a JSON data wrapped in a function call. JSONP is an effective cross-domain communication technique, by-passing the same-origin policy limitations. In this post, we will show you how to bind the jQuery Grid to JSONP data. We will use the Geonames JSONP Service to populate our Grid with data.
- Add references to the JavaScript and CSS files:
<link rel="stylesheet" href="styles/jqx.base.css" type="text/css" />
<link rel="stylesheet" href="styles/jqx.classic.css" type="text/css" />
<script type="text/javascript" src="jquery-1.7.1.min.js"></script>
<script type="text/javascript" src="jqxcore.js"></script>
<script type="text/javascript" src="jqxbuttons.js"></script>
<script type="text/javascript" src="jqxscrollbar.js"></script>
<script type="text/javascript" src="jqxmenu.js"></script>
<script type="text/javascript" src="jqxgrid.js"></script>
- Add a
DIV
tag to the document’s body
. We will later select this DIV
tag to render the Grid
inside.
<div id="jqxgrid"></div>
- Create the source object. In the source object, set the
url
to point to the JSONP service. The processdata
callback function is called before the Ajax call to the remote data. You can use this callback function to process the data to be sent to the server. The datatype member should be set to ‘jsonp
’ and we also specify the datafields
array. Each datafield
name is a name of a member in the data source.
var source =
{
datatype: "jsonp",
datafields: [
{ name: 'countryName' },
{ name: 'name' },
{ name: 'population' },
{ name: 'continentCode' }
],
url: "http://ws.geonames.org/searchJSON",
processdata: function(data)
{
data.featureClass = "P";
data.style = "full";
data.maxRows = 50;
}
};
- The final step is to initialize the
Grid
. We achieve this by selecting the jqxgrid DIV
tag and calling the jqxGrid
constructor. In the constructor, we set the source
property to point to the source
object. We also initialize the Grid
columns by setting the displayed text, datafield
which points to a datafield
in the source
object and the column’s width.
$("#jqxgrid").jqxGrid(
{
source: source,
theme: 'classic',
columns: [
{ text: 'Country Name', datafield: 'countryName', width: 200},
{ text: 'City', datafield: 'name', width: 170 },
{ text: 'Population', datafield: 'population', width: 170 },
{ text: 'Continent Code', datafield: 'continentCode' }
]
});
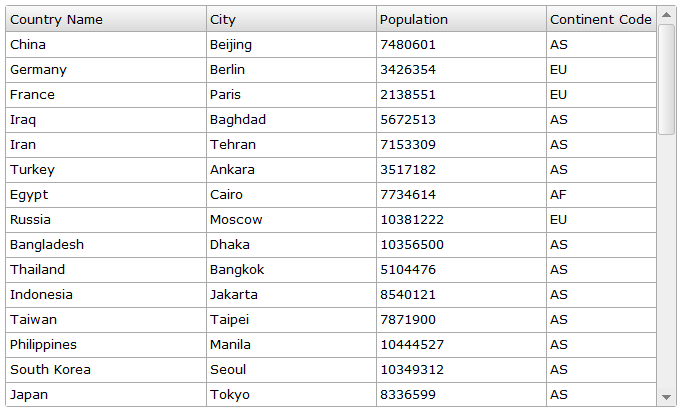
CodeProject