Introduction
In VB6, you could use various API calls to get the FileDropList
of files on the Clipboard as well as a couple of more calls to get the DropEffect
that tells whether the files where copied or cut from the Windows File Explorer.
In VB.NET, it’s even easier to get the FileDropList
from the clipboard using the specialized string collection. But I could not find how to get the DropEffect
. By looking at what the old method did, and using a C# example I found, I was able to get at the DropEffect
.
To get the DropEffect
, set an Object
to My.Computer.Clipboard.GetData("Preferred DropEffect")
, and then read the first 4 bytes into a byte array. The first byte is the DropEffect
. I am not using the other 3 bytes, but the old code was grabbing them due to variable formats, so I did too.
Get FileDropList and DropEffect in VB6
In VB6, you can use calls to the Clipboard functions to retrieve the file list, and then another call to get the data with the drop effect. A call to MoveMemory
puts the data in a format we can use. (Full examples of how to do this in VB6 can be found elsewhere).
Private Declare Function GetClipboardData Lib "user32" (ByVal wFormat As Long) As Long
Private Declare Function IsClipboardFormatAvailable _
Lib "user32" (ByVal wFormat As Long) As Long
Private Declare Function RegisterClipboardFormat Lib "user32" +
Alias "RegisterClipboardFormatA" (ByVal lpString As String) As Long
Private Declare Sub MoveMemory Lib "kernel32" Alias "RtlMoveMemory" _
(ByRef Destination As Any, ByRef Source As Any, ByVal Length As Long)
Dim hDrop As Long
Dim nFiles As Long
Dim i As Long
Dim desc As String
Dim Filename As String
Dim pt As POINTAPI
Dim lngEffect As Long
Dim lngFormat As Long
Dim hGlobal As Long
Const MAX_PATH As Long = 260
If IsClipboardFormatAvailable(CF_HDROP) Then
If OpenClipboard(0&) Then
hDrop = GetClipboardData(CF_HDROP)
nFiles = DragQueryFile(hDrop, -1&, "", 0)
ReDim Files(0 To nFiles - 1) As String
Filename = Space(MAX_PATH)
For i = 0 To nFiles - 1
Call DragQueryFile(hDrop, i, Filename, Len(Filename))
Files(i) = TrimNull(Filename)
Next
lngFormat = RegisterClipboardFormat(CFSTR_PREFERREDDROPEFFECT)
hGlobal = GetClipboardData(lngFormat)
If (hGlobal) Then
MoveMemory lngEffect, ByVal hGlobal, 4
DropEffect = lngEffect
End If
End If
End If
Get FileDropList and DropEffect in VB.NET
Getting the DropEffect
in VB.NET requires getting the “Preferred DropEffect” data off the clipboard and reading it with a memory stream.
If My.Computer.Clipboard.ContainsFileDropList() Then
Dim DropEffectData(3) As Byte
Dim DropEffectCheck As Object = _
My.Computer.Clipboard.GetData("Preferred DropEffect")
DropEffectCheck.Read(DropEffectData, 0, DropEffectData.Length)
Select Case DropEffectData(0)
Case 2
DropEff = DragDropEffects.Move
DropEffType = "Move"
Case 5
DropEff = DragDropEffects.Copy
DropEffType = "Copy"
Case Else
DropEffType = "???"
End Select
DropEffNum = DropEffectData(0).ToString
TextBox1.Text = String.Concat(DropEffNum, " - ", DropEffType)
Dim FileNameCollection As Collections.Specialized.StringCollection = _
My.Computer.Clipboard.GetFileDropList()
For Each FileName As String In FileNameCollection
RichTextBox1.Text = String.Concat(RichTextBox1.Text, FileName, vbCrLf)
Next
End If
Sample App
The sample application shows the DropEffect
and the list of files you put on the Clipboard. Switch between cutting and copying files from the File Explorer to see the DropEffect
change.
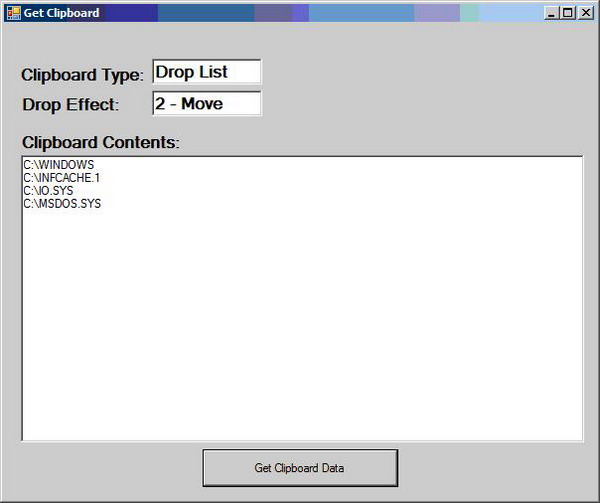