Introduction
The article gives an overview of the steps and code required to create, fill, and upload an InfoPath form using code.
Design Infopath Form
Create an InfoPath form using the Microsoft InfoPath designer. This sample form will store the name and surname of the client and a table of products and amounts.
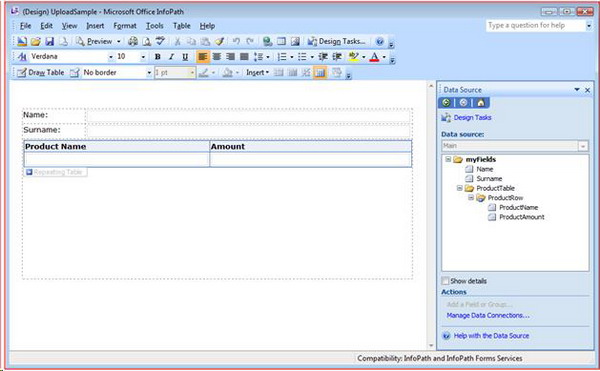
Create Class Representation of the Infopath Form
- Save the InfoPath form:

Figure 2 - Save as Source File
- Load the Visual Studio Command Prompt:

- Locate form the source files:

- Execute the command xsd.exe /c /l:CS myschema.xsd.
The XSD tool forms part of the .NET framework tools. We will use it to generate a common language class from the XSD file representation of the InfoPath form.

Figure 5 – Generated myschema class
[System.CodeDom.Compiler.GeneratedCodeAttribute("xsd", "2.0.50727.1432")]
[System.SerializableAttribute()]
[System.Diagnostics.DebuggerStepThroughAttribute()]
[System.ComponentModel.DesignerCategoryAttribute("code")]
[System.Xml.Serialization.XmlTypeAttribute(AnonymousType=true,
Namespace="http://schemas.microsoft.com/office/infopath/2003/myXSD/2009-02-04T09:20:42")]
[System.Xml.Serialization.XmlRootAttribute(
Namespace="http://schemas.microsoft.com/office/infopath/2003/myXSD/2009-02-04T09:20:42",
IsNullable=false)]
public partial class myFields {
private string nameField;
private string surnameField;
private ProductRow[] productTableField;
private System.Xml.XmlAttribute[] anyAttrField;.....
.....
.....
Once the file is available in the project, you have the capability to bind the data source to the .NET class and access the data in a standardized format. If you ever make changes to your InfoPath data source, you’ll need to rerun the xsd.exe command against the new “schema.xsd” file.
Upload Form Template to a SharePoint Form Site

Figure 6 – Publish

Figure 7

Figure 8 – Select SharePoint Server

Figure 9

Figure 10

Figure 11

Figure 12

Figure 13
Populate and Upload Form Data
Populate the myFields
class with dummy data. The class was created using the XSD application.
myFields fields = new myFields();
fields.Name = "Joe";
fields.Surname = "Blogs";
ProductRow[] rows = new ProductRow[2];
rows[0] = new ProductRow();
rows[0].ProductAmount = "1";
rows[0].ProductName = "Peach Jam";
rows[1] = new ProductRow();
rows[1].ProductAmount = "2";
rows[1].ProductName = "Strawberry Jam";
fields.ProductTable = rows;
MemoryStream myInStream = new MemoryStream();
string rFormSite = @"http://sp/Sample Form";
string rTemplateLocation = @"http://sp/Sample Form/Forms/template.xsn";
using (myInStream)
{
XmlSerializer serializer = new XmlSerializer(typeof(myFields));
XmlTextWriter writer = new XmlTextWriter(myInStream, Encoding.UTF8);
string rInstruction =
"name=\"urn:schemas-microsoft-com:office:infopath:" +
"UploadSample:-myXSD-2009-02-04T09-20-42\"";
rInstruction +=
"solutionVersion=\"1.0.0.2\" productVersion=\"12.0.0.0\"
PIVersion=\"1.0.0.0\" href=\"" + rTemplateLocation + "\"";
writer.WriteProcessingInstruction("mso-infoPathSolution", rInstruction);
writer.WriteProcessingInstruction("mso-application",
"progid=\"InfoPath.Document\" versionProgid=\"InfoPath.Document.2\"");
serializer.Serialize(writer, fields);
using (SPSite site = new SPSite(rFormSite))
{
using (SPWeb spweb = site.OpenWeb())
{
spweb.Files.Add(rFormSite + "\\" + Path.GetRandomFileName() + ".xml",
myInStream.GetBuffer());
}
}
}
Result

Figure 14 – New Item in Form Library

Figure 15 – Form Loaded with Data