Knockoutjs is a type safe client side library which provides declarative bindings of DOM with model data, Automatic UI refresh when view model changes, etc. You can read more about KnockoutJS on its website. You can read more about AspNet MVC3 on ASP.NET MVC website.
Model
Create Model
class on server side MarketPriceModel
, its collection class MarketPriceCollectionModel
.
public class MarketPriceCollectionModel
{
public List Prices { get; private set; }
public void Add(MarketPriceModel model)
{
if (Prices == null)
Prices = new List();
Prices.Add(model);
}
}
public class MarketPriceModel
{
public string Symbol { get; set; }
public double Open { get; set; }
public double Close { get; set; }
public double Low { get; set; }
public double High { get; set; }
public DateTime TimeStamp { get; set; }
}
Controller: MarketWatchController
This controller class has action method “MarketPriceUI
” which returns MarketPriceUI
view and injects MarketPriceCollectionModel
class as model.
public class MarketWatchController : Controller
{
public ActionResult MarketPriceUI()
{
MarketPriceCollectionModel collection = new MarketPriceCollectionModel();
collection.Add(new MarketPriceModel() { Symbol = "MSFT",
Open = 34.5d, Close = 35.5d, Low = 33.4d, High = 36.5d });
collection.Add(new MarketPriceModel() { Symbol = "Goog",
Open = 54.5d, Close = 58.65d, Low = 52.78d, High = 59.23d });
return View(collection);
}
}
View MarketPriceUI.cshtml
@model
: contains object returns from controller. We can use this object to show data in view, but here I want to convert @model
to knockoutJS viewmodel
.
var initialData = @(Html.Raw(Json.Encode(Model.Prices)));
viewModel = {prices: ko.observableArray(initialData)
};
ko.applyBindings(viewModel);
@(Html.Raw(Json.Encode(Model.Prices)))
converts model data into json and this data is set as observablearray for knockoutjs viewmodel
. “data-bind="foreach: viewModel.prices"
statement can loop in this viewmodel
to show data on page.
@model MarketWatchWebSample.Models.MarketPriceCollectionModel
@{
ViewBag.Title = "MarketWatch";
Layout = "~/Views/Shared/_Layout.cshtml";
}
var viewModel;
$(document).ready(function () {
var initialData = @(Html.Raw(Json.Encode(Model.Prices)));
viewModel = {prices: ko.observableArray(initialData)
};
ko.applyBindings(viewModel);
});
}
<table>
<tr>
<td>
Symbol
</td>
<td>
High
</td>
<td>
Low
</td>
</tr>
<tbody data-bind="foreach: viewModel.prices">
<tr>
<td>
<span data-bind="text:Symbol"></span>
</td>
<td>
<span data-bind="text:High"></span>
</td>
<td>
<span data-bind="text:Low"></span>
</td>
</tr>
</tbody>
</table>
Output
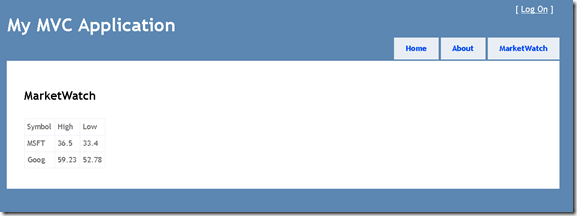
