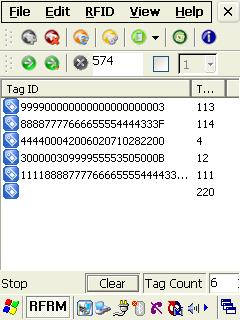
Introduction
This is a program for a 900 MHz UHF (Ultra High Frequency) RFID (Radio Frequency Identification). This program must have a Samsung Techwin UHF Handheld RFID Reader (VLAC-G1) or a UHF Fixed RFIDReader (VLAC-Alpha) based InpinJ R1000 chipset.
Background
Many people listen to RFID. This technology has numerous possibilities for the distribution industry, food hygiene, air cargo, public library, etc......
This technology is constructed using an RFID Tag, an RFID Reader, and a Service. The RFID Tag is a very low cost product. Several months ago, Hynix Semiconductors said, "We will make 1 cent RFID tag chips." The RFID Reader is more expensive than RFID tags.
However, this might be perfect for any distribution vendor considering applications for low cost of transport and reliability of transport. And, many food companies might consider its application for food safety. For example, an industry vendor might consider changing from 2D barcode technology to RFID technology.
This program will be very useful for RF tag identification.
RFID Tags Structure
The 900MHz RFID Tags is composed of a tag chip and an antenna. The tag chip is based on the ISO 18000-4 spec for automatic data identification specification and ISO-18000-6C specialization for item level management tags. The latest trend is 18000-6C. We call these Gen2 tags.
The detail structure searches the EPC Global standard. These tags are composed of BANK 00 (Reserved Memory), BANK 01 (EPC Memory), BANK 10 (TID), and BANK 11 (User Memory).
- The BANK 00 (Reserved memory) is composed of a kill password area and an access password area for secure functions.
- The BANK 01 (EPC memory) is composed of CRC-16 (tag used when protecting certain R=> T, T => R), PC (Protocol control for EPC memory area), and EPC (Electronic Product Code).
- The BANK 10 (TID memory) is the 8 bit ISO/IEC 15963 allocation class identifier; 11100010 for EPC Global.
- The BANK 11 (User memory) is the user specification data storage.
How to Read RFID Tags in this Program
This program is composed of RFID Radio (CRFIDRadio
) and RFID Radio Management (CRFIDRadioManager
) objects.
The CRFIDRadio
object is a real command operator. This object has some functions for the Inventory command, Tag Access (Read
/BlockRead
/Write
/BlockWrite
/Lock
/Kill
) commands, and the RFID setting function. And, this object calls the Samsung Techwin RFID Reader API.
The CRFIDRadioManager
object is to manage each RFID Radio object. And, this object controls CRFIDRadio
objects for command transport.
First, you must create a CRFIDRadioManager
:
CRFIDRadioManager m_pRFIDRadioManager;
m_pRFIDRadioManager = new CRFIDRadioManager();
and connect to RFID Reader device like this:
m_pRFIDRadioManager->SetParnet(CWnd* Object);
if(m_pRFIDRadioManager->Connect() != RFID_STATUS_OK)
{
}
else
{
}
This function makes a connection to a CRFIDRadio
object. In this state, the CRFIDRadioManager
object searches and attaches an RFID Reader device on your accessible RFID Reader using USB, Serial, and TCP/IP interfaces. And, the SetParent
function sets the CWnd
window for Win32 message returning. This state is a complete reader connection.
Next, you will want identify the RFID tag. Look at the RunInventory
function in the CRFIDRadioManager
. This function sends tags for the read command to the RFID reader. It creates a thread for the read command.
void CMainFrame::OnRfidRunInventory()
{
pRFIDRadioManager->RunInventory(0, -1);
}
RFID_STATUS CRFIDRadioManager::RunInventory(int nModule, INT32 nCount)
{
RFID_STATUS status = RFID_STATUS_OK;
if(m_listRadio.GetCount() > 0)
{
POSITION pos = m_listRadio.FindIndex(nModule); CRFIDRadio* pRadio = (CRFIDRadio*)m_listRadio.GetAt(pos);
pRadio->m_nStopCountDown = nCount; pRadio->m_runStatus = RFID_INVENTORY;
_oleStartDateTime = COleDateTime::GetCurrentTime();
Radio->Create(); }
return status;
}
The packet parameter is the result information from the reader. The base structure is RFID_PACKET_COMMON
. For detailed structure information, see the Interface Specification white paper in the Samsung Techwin homepage.
The CRFIDRadioManager
returns the parent CTagInfo
object using the SetParent
function.
case RFID_PACKET_TYPE_18K6C_INVENTORY:
{
RFID_PACKET_18K6C_INVENTORY *inv =
(RFID_PACKET_18K6C_INVENTORY *)packet;
int length =
(MacToHost16(common->pkt_len)-1) * BYTES_PER_LEN_UNIT -
(common->flags >> 6);
if(common->flags & 0x01)
break;
int padding_byte = (int)(common->flags & 0xC0);
POSITION pos = m_listRadio.FindIndex(0);
CRFIDRadio* pRadio = (CRFIDRadio*)m_listRadio.GetAt(pos);
if(pRadio->m_runStatus != RFID_INVENTORY)
{
CString strTemp = TEXT("Tag ID : ");
CTagInfo lpTagInfo; lpTagInfo.SetTagMemory(inv->inv_data);
strTemp += lpTagInfo.GetTagString();
theApp.m_pMainWnd->SendMessage(WM_TAG_ACCESS, (WPARAM)(LPCTSTR)strTemp, 0);
#ifdef _WIN32_WCE
if(m_radioBeepMode == RFID_RADIO_BEEP_MODE_ENABLE)
m_waveBox->Play(0);
#endif
break;
}
CTagInfo* lpTagInfo = new CTagInfo();
lpTagInfo->SetTagMemory(inv->inv_data);
lpTagInfo->SetTagReadCount(inv->count);
lpTagInfo->SetTagTrialCount(inv->trial_count);
lpTagInfo->SetTagAntenna(m_nAntenna);
lpTagInfo->SetTagChannel(m_nChannel);
lpTagInfo->SetTagRSSI(MacToHost16(inv->rssi));
lpTagInfo->SetTagLNAGain(MacToHost16(inv->ana_ctrl1));
lpTagInfo->SetTagReveiveTime(COleDateTime::GetCurrentTime());
ifdef _WIN32_WCE
if(m_radioBeepMode == RFID_RADIO_BEEP_MODE_ENABLE)
_waveBox->Play(0);
#endif
m_pParent->SendMessage(WM_PROCESS_TAG, (WPARAM)lpTagInfo);
break;
}
The CTagInfo
object copies tag information from the RFID Reader. And, the CTagInfo
object reconstructs PC, EPC, and other information using the ISO 18000-6C tag type.
I do type cast from the packet parameter to the RFID_PACKET_18K6C_INVENTORY
struct. And, I define the inv variance. I check the packet length and the flag for the right packet type. If the packet is right, I make a CTagInfo
object for tag information. Finally, I return a CTagInfo
object to the parent object using the SendMessage
function. The complete source code is available from the download link above.