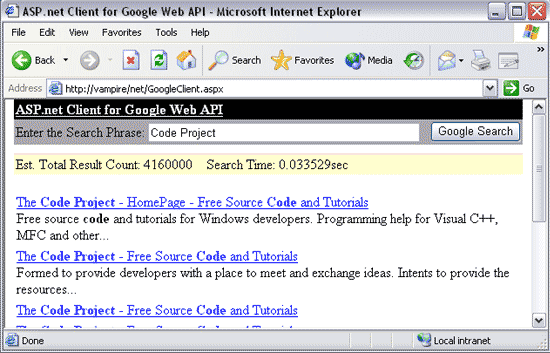
Introduction
Recently I got an opportunity to explore the Google Web API which can be downloaded from the
Google site. So I decided to code the web-client which is
very easy and interesting too! The article focuses on developing the
Web-Client with which you can search items from your site itself.
Installation & Setup
You must download the google web api first! Then follow
the instructions below.
- Create the folder like
"Net" within "wwwroot" folder and make that folder web application.
- Create "bin" sub-folder within the "Net"
folder.
- Make
GoogleProxy.cs
using the WSDL tool and compile GoogleProxy.cs
into the
.net assembly using csc
command to generate the
proxy.
-
>wsdl
/l:cs /o:GoogleProxy.cs <a href="http://localhost/Net/GoogleSearch.wsdl">http:
/n:GoogleWebService
This
generates the GoogleProxy.cs
. "GoogleSearch.wsdl
" is
found in the API you have downloaded. -
>csc /out:GoogleProxy.dll /t:library /r:system.dll, system.web.dll,
<br>
system.xml.dll, system.web.services.dll
GoogleProxy.cs
This generates
the .net assembly, GoogleProxy.dll. Copy the dll file into "bin" folder.
Your ASP.NET page will ultimately call the web-callable methods and properties
exposed by this dll.
- Write
GoogleClient.aspx
file to create the UI and to consume the
services exposed by google web api, to be precise GoogleProxy.dll in our
case. - Browse
http://localhost/Net/GoogleClient.aspx
. That's it!
Why proxy?
A proxy resides on the consumer's machine and acts as a
rely between the consumer and the web service. When we build the proxy, we use
WSDL file to create a map that tells the consumer what methods are available and
how to call them. The consumer then calls the web method that is mapped in the
proxy, which in turn, makes calls to the actual web service over the Internet.
The proxy handles all of the network-related work and sending of data, as well
as managing the underlying WSDL so the consumer doesn't have to. When we
reference the web service in the consumer application, it looks as if it's part of the consumer application itself.
Using the code
The code is pretty straight forward.
<%@ Page Language="C#" %>
<@ import Namespace="GoogleWebService" >
//Remember,GoogleWebService is the namespace you named while creating the proxy!
<script runat="server">
string key="licence key you got from google";
void Page_Load()
{
lblSpellSug.Text="";
lblResultCount.Text="";
lblSearchTime.Text="";
}
void btnSearch_Click(Object sender, EventArgs e) {
GoogleSearchService obj=new GoogleSearchService();
string suggestion=obj.doSpellingSuggestion(key,Request.Form["txtPhrase"]);
if (suggestion!=null)
{
lblSpellSug.Text="Suggestion: "+ suggestion;
}
GoogleSearchResult res=obj.doGoogleSearch(key, Request.Form["txtPhrase"],
0, 10, false,"",false,"","","");
lblResultCount.Text="Est. Total Result Count: " +
Convert.ToString(res.estimatedTotalResultsCount);
lblSearchTime.Text= "Search Time: " + Convert.ToString(res.searchTime) +
"sec";
ResultElement[] result=res.resultElements;
foreach(ResultElement r in result)
{
ResultTable.CellSpacing=1;
ResultTable.CellPadding=2;
TableRowCollection trCol=ResultTable.Rows;
TableRow tr=new TableRow();
trCol.Add(tr);
TableCellCollection tcCol=tr.Cells;
TableCell tc=new TableCell();
tc.Text="<a href="+ r.URL +">"+ r.title + "</a>" + "<BR>" + r.summary;
tcCol.Add(tc);
}
}
</script>
Conclusion
Let's share the solution.
Milan has been developing commercial applications over a decade primarily on Windows platform. When not programming, he loves to test his physical endurance - running far away to unreachable distance or hiking up in the mountains to play with Yaks and wanting to teach 'Yeti' programming!