Download JigsawPuzzleGameIn-Html5.zip (773.2 KB)
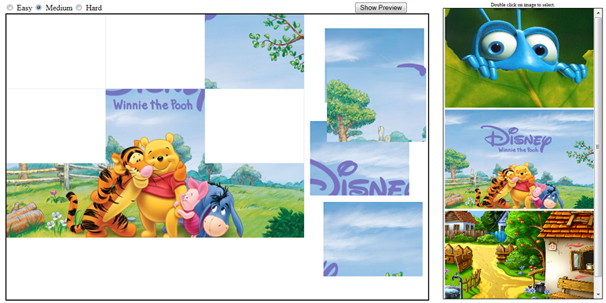
Introduction
HTML5 is getting famous day by day. Some fantastic and amazing games have been building
using HTML5. So I thought to create Jigsaw game using HTML 5. It is very famous
game to arrange a blocks to complete image. I used HTML5 canvas which is very handy
and efficient way to render image.
Create Image Blocks
For saving information of each block, create imageBlock
class, which will contain
the information of each block. For drawing the image block in the canvas, we will
simply get block information from array and draw it accordingly.
function imageBlock(no, x, y) {
this.no = no;
this.x = x;
this.y = y;
this.isSelected = false;
}
For breaking image into the parts, we need to push each imageBlock
into the array
and set the random x and y coordinates for each block. We also prepare final destination
array having coordinates for each block. This array helps us to get information
on drop of the image block and also checking how many image blocks has right position.
function SetImageBlock() {
var total = TOTAL_PIECES;
imageBlockList = new Array();
blockList = new Array();
var x1 = BLOCK_IMG_WIDTH + 20;
var x2 = canvas.width - 50;
var y2 = BLOCK_IMG_HEIGHT;
for (var i = 0; i < total; i++) {
var randomX = randomXtoY(x1, x2, 2);
var randomY = randomXtoY(0, y2, 2);
var imgBlock = new imageBlock(i, randomX, randomY);
imageBlockList.push(imgBlock);
var x = (i % TOTAL_COLUMNS) * BLOCK_WIDTH;
var y = Math.floor(i / TOTAL_COLUMNS) * BLOCK_HEIGHT;
var block = new imageBlock(i, x, y);
blockList.push(block);
}
}
Get all image Blocks information from array and if block is not selected then simply
draw it.
function drawAllImages() {
for (var i = 0; i < imageBlockList.length; i++) {
var imgBlock = imageBlockList[i];
if (imgBlock.isSelected == false) {
drawImageBlock(imgBlock);
}
}
}
Use drawImage
method of the canvas for drawing block. Calculate source x and y coordinates
based on block number and pass them to the ctx.drawImage(...)
method
to draw image block. This method read image based on provided information and draw
it into canvas on given destination coordinates.
function drawImageBlock(imgBlock) {
drawFinalImage(imgBlock.no, imgBlock.x, imgBlock.y, BLOCK_WIDTH, BLOCK_HEIGHT);
}
function drawFinalImage(index, destX, destY, destWidth, destHeight) {
ctx.save();
var srcX = (index % TOTAL_COLUMNS) * IMG_WIDTH;
var srcY = Math.floor(index / TOTAL_COLUMNS) * IMG_HEIGHT;
ctx.drawImage(image1, srcX, srcY, IMG_WIDTH, IMG_HEIGHT, destX, destY, destWidth, destHeight);
ctx.restore();
}
Drag Image Block
For drag image block, register mouse down, up and move event of canvas.
canvas.onmousedown = handleOnMouseDown;
canvas.onmouseup = handleOnMouseUp;
canvas.onmouseout = handleOnMouseOut;
canvas.onmousemove = handleOnMouseMove;
On mouse down simple find imageBlock in the collection lay between click positions
of mouse. If item found then assign it to the selectedBlock
object.
function handleOnMouseDown(e) {
if (selectedBlock != null) {
imageBlockList[selectedBlock.no].isSelected = false;
}
selectedBlock = GetImageBlock(imageBlockList, e.pageX, e.pageY);
if (selectedBlock) {
imageBlockList[selectedBlock.no].isSelected = true;
}
}
On mouse move if it has selectedBlock
then set new x and y position
of the selected block and redraw the canvas.
function handleOnMouseMove(e) {
if (selectedBlock) {
selectedBlock.x = e.pageX - 25;
selectedBlock.y = e.pageY - 25;
DrawGame();
}
}
On mouse up check if it is on the block and block does not has image then set source
image coordinates accordingly and redraw the canvas. If game is finished with this
move then make it finish.
function handleOnMouseUp(e) {
if (selectedBlock) {
var index = selectedBlock.no;
var block = GetImageBlock(blockList, e.pageX, e.pageY);
if (block) {
var blockOldImage = GetImageBlockOnEqual(imageBlockList, block.x, block.y);
if (blockOldImage == null) {
imageBlockList[index].x = block.x;
imageBlockList[index].y = block.y;
}
}
else {
imageBlockList[index].x = selectedBlock.x;
imageBlockList[index].y = selectedBlock.y;
}
imageBlockList[index].isSelected = false;
selectedBlock = null;
DrawGame();
if (isFinished()) {
OnFinished();
}
}
}
Play Audio on Finish
On the finish of the game for playing music simply creates audio object using javaScript
and set its source and play it.
function OnFinished() {
var audioElement = document.createElement('audio');
audioElement.setAttribute('src', 'Audio/finish.mp3');
audioElement.play();
.
.
.
}