The challenge
Many of today's applications require the capability of extracting certain files from a ZIP archive, either onto the hard disk or into memory. A lot of useful zip libraries for .NET applications abound on the Web (SharpZipLib for .NET Framework is a very known one), but I wanted to use the Info-ZIP library as it was always my favorite library for C++ projects.
Info-ZIP is an Open Source version of Phil Katz's "deflate" and "inflate" routines used in his popular file compression program, PKZIP. Info-ZIP code has been incorporated into a number of third-party products as well, both commercial and freeware. It offers two dynamic link libraries: one for zipping, and one for unzipping.
The Info-ZIP DLLs are free to use and distribute, but they are designed to be used in C/C++ projects, so they're not really .NET-friendly. Also, the Info-ZIP package contains almost no documentation showing how to use the Info-ZIP DLLs.
Therefore, I decided to write a small C# wrapper that provides all the required data types and functions in order to give the possibility to work with the Info-ZIP API.
The solution
I built the Info-ZIP .NET wrapper as a C# library named Karna Compression where all the Info-ZIP native types and methods are hidden behind the simple .NET facade. Karna Compression library has two main classes: KarnaZip
and KarnaUnzip
.
KarnaZip
is a class for dealing with zip archives that can add, freshen, or move files in an archive.
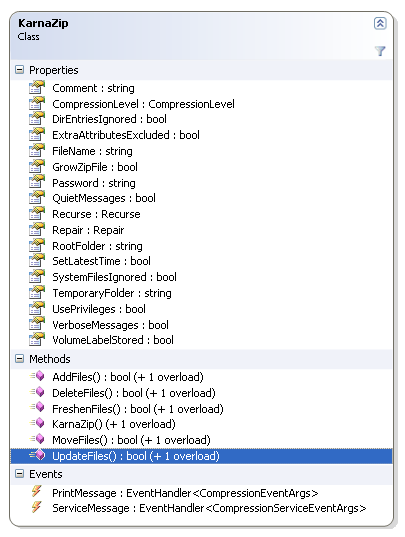
KarnaUnzip
can be used to extract files from a ZIP archive to the hard drive or memory.

Using the code
Creation of zip archive, including password protection and comments, requires just a few lines of code:
string[] content = { "*.txt" };
KarnaZip zip = new KarnaZip();
zip.FileName = "myziparchive.zip";
zip.Password = "password";
zip.Comment = "This is my documents archive";
zip.AddFiles(content);
Other operations with a zip archive can be performed in a similar way:
zip.UpdateFiles(content);
zip.MoveFiles(content);
zip.DeleteFiles(content);
Extracting files from the archive using the KarnaUnzip
class is even more simple:
KarnaUnzip unzip = new KarnaUnzip("myziparchive.zip");
unzip.Password = "password";
unzip.ExtractArchive();
The following code snippet shows how to extract a file from the archive to memory without saving the file to the hard drive:
byte[] rawBytes;
KarnaUnzip unzip = new KarnaUnzip("myziparchive.zip");
rawBytes = unzip.ExtractToMemory("readme.txt");
Additional information
Karna Compression is a small part of the Open Source Karna .NET library which can be downloaded from the SourceForge website: http://www.sourceforge.net/projects/karna.
More information about the Info-ZIP project can be found on Info-ZIP's home site: www.info-zip.org.
History
- 25.05.2009: Initial release.