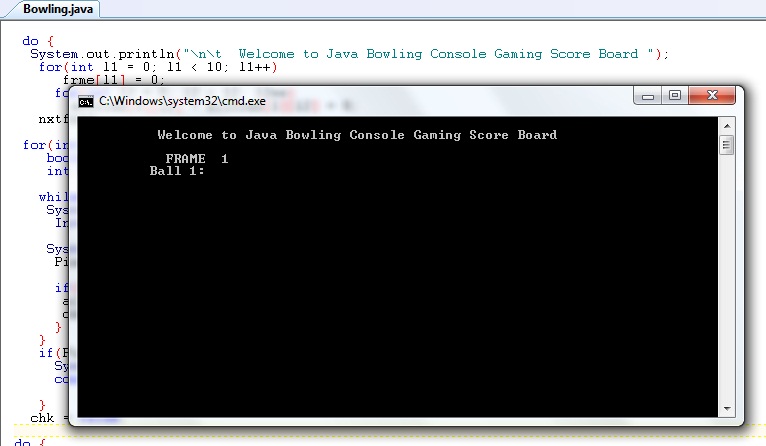
Introduction
The following Java console code is about viewing your scores after each sequence of Pins the player hits. The Java Console Bowling program is useful
for keeping track of all your score and displaying all the scenarios possible each time you enter your bowling scores.
Also, this java code is useful as a plugin to incorporate it within your java program because it can populate data within a array structure and calculating the variables stored.
Enjoy!
Background
The Java Game allows you to add your scores and keep track of the player's score as they hit each Pin in the Bowling game. Ball 1 score shows the number of pins knocked down with the first ball and Ball 2
score shows the number of pins knocked down with the second ball while assuming a second ball is used. The total score will display the total score up to and
including that possible 10th frame extra ball.

Using the code
To use the code, you will need a java editor such as TextPad
and the Java JDK on your computer. Using Java classes can run on the major Operating Systems on the market with the JDK installed.
On completing the installation requirements, running the java code should be simple. However, if you like to create a bat or exe file to run
your class file it can be done. Enter the following code with your text editor and save it as Bowling.bat
echo "Launching My Java Bowling game Score Board"
java -jar "location of java file (Bowling.java)"
Display the Console Menu Interface
The following java code below shows the menu of the Java game (refer to the comments):
int frme[] = new int[10];
int allfrme[][] = new int[2][12];
String s;
do {
System.out.println("\n\t Welcome to Java Bowling Console Gaming Score Board ");
for(int l1 = 0; l1 < 10; l1++)
frme[l1] = 0;
for(int l2 = 0; l2 < 12; l2++)
allfrme[0][l2] = allfrme[1][l2] = 0;
nxtfrme:
for(int i = 0; i < 10; i++) {
boolean chk = false;
int Pins = 0;
while(!chk) {
System.out.printf("\n\t FRAME %2d ", new Object[] {
Integer.valueOf(i + 1) });
System.out.printf("\n\t Ball 1: ");
Pins = keyIn.nextInt();
if(Pins <= 10 && Pins >= 0) {
allfrme[0][i] = Pins;
chk = true;
}
}
if(Pins == 10) {
System.out.println("\n\t\t\tExcelent, Strike!!");
continue;
}
chk = false;
Displaying your bowling score in two dimension
The code below display the Score after the user or player finish entering their Bowling scores. As the user enter each strings place it within its addresses,
convert the string as an object int value and calculate each interval value as int as place in each array address.
if(allfrme[0][0] + allfrme[0][1] == 10)
frme[0] = 10 + allfrme[0][1];
else
frme[0] = allfrme[0][0] + allfrme[1][0];
if(allfrme[0][0] == 10) {
if(allfrme[0][1] == 10)
frme[0] = 20 + allfrme[0][2];
else
frme[0] = 10 + allfrme[0][1] + allfrme[1][1];
}
for(int j = 1; j < 10; j++) {
if(allfrme[0][j] == 10){
if(allfrme[0][j + 1] == 10)
frme[j] = frme[j - 1] + 20 + allfrme[0][j + 2];
else
frme[j] = frme[j - 1] + 10 + allfrme[0][j + 1] + allfrme[1][j + 1];
continue;
}
if(allfrme[0][j] + allfrme[1][j] == 10)
frme[j] = frme[j - 1] + 10 + allfrme[0][j + 1];
else
frme[j] = frme[j - 1] + allfrme[0][j] + allfrme[1][j];
}
System.out.print("\n\n\t FRAME");
for(int k = 1; k < 11; k++)
System.out.printf("%4d", new Object[] {
Integer.valueOf(k) });
if(allfrme[0][9] == 10) {
if(allfrme[0][10] == 10)
System.out.print(" XTR1 XTR2");
else
System.out.print(" EXTRA");
}else if(allfrme[0][9] + allfrme[1][9] == 10)
System.out.print(" XTRA");
System.out.print("\n\n\tBall 1 ");
for(int l = 0; l < 10; l++)
System.out.printf("%4d", new Object[] {
Integer.valueOf(allfrme[0][l])
});
if(allfrme[0][9] == 10) {
if(allfrme[0][10] == 10)
System.out.printf("%4d%4d", new Object[] {
Integer.valueOf(allfrme[0][10]), Integer.valueOf(allfrme[0][11]) });
else
System.out.printf("%4d", new Object[] {
Integer.valueOf(allfrme[0][10]) });
} else
if(allfrme[0][9] + allfrme[1][9] == 10)
System.out.printf("%4d", new Object[] {
Integer.valueOf(allfrme[0][10])
});
System.out.print("\n\tBall 2 ");
for(int i1 = 0; i1 < 10; i1++)
System.out.printf("%4d", new Object[] {
Integer.valueOf(allfrme[1][i1])
});
if(allfrme[0][9] == 10 && allfrme[0][10] != 10)
System.out.printf("%4d", new Object[] {
Integer.valueOf(allfrme[1][10])
});
System.out.print("\n\n\t SCORE");
for(int j1 = 0; j1 < 10; j1++)
System.out.printf("%4d", new Object[] {
Integer.valueOf(frme[j1])
});
System.out.print("\n\n\t\t\tPlay more (Y/N)? ");
s = keyIn.next();
String s1 = keyIn.nextLine();
} while(s.toUpperCase().charAt(0) == 'Y');
}
Really simple, yet complicated java program because of getting the values to print in a two dimension pattern in Console. Run it yourself and discuss any issues,
suggestions, and improvements towards creating a bowling game.
Points of interest
Challenge yourself and see how you could use few set of for loops with if statements to achieve various programming issues.
History
Created: Nov. 30 2011.