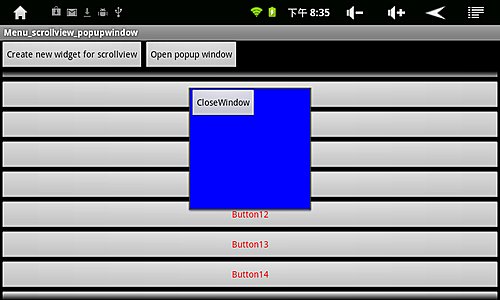
Introduction
The examples in this article will create menu, scroll view and popup window. For menu widget, programmer should override Android functions of activity, which are “onCreateOptionsMenu
”, “onPrepareOptionsMenu
”, “onOptionsItemSelected
”. Using Python, the same functions should be defined and assigned to activity object. Popup window is often used to show information or perform some interactions with customer. Python permits define functions in another function, which is convenient to define widgets in popup window.
Scroll View
Creating scroll view is more directly and simple.
Create Scroll View Object
MyScroll = Service.ScrollViewClass._New(MyLayout);
MyScroll.setLinearLayoutParams(Service.FILL_PARENT,Service.WRAP_CONTENT);
Create Root Layout in the Scroll View
MyScrollLayout = Service.LinearLayoutClass._New(MyScroll);
MyScrollLayout.setOrientation("VERTICAL");
MyScrollLayout.setFrameLayoutParams(Service.FILL_PARENT,Service.WRAP_CONTENT);
Create a Button, When Clicked, We Create A New Button in Scroll View
MyButtonIndex = 1;
//
def MyButton_onClick(self,Ev) :
global MyButtonIndex;
//
b = Service.ButtonClass._New(MyScrollLayout);
//
b.setText("Button" + str(MyButtonIndex));
b.setTextColor(0xFFFF0000);
b.setLinearLayoutParams(Service.FILL_PARENT,Service.WRAP_CONTENT);
MyButtonIndex = MyButtonIndex + 1;
return;
//
MyButton.onClick = MyButton_onClick;
Menu
For menu, we should override functions of the Activity
object which are onCreateOptionsMenu
, onPrepareOptionsMenu
, and onOptionsItemSelected
. The activity
object is obtained using code “StarActivity = Service.ActivityClass.getCurrent();
”.
Override Function “onCreateOptionsMenu”
In this function, we create some menu items:
def StarActivity_onCreateOptionsMenu(self,menu) :
menu.add1(0, 1, 1, "open");
menu.add1(0, 2, 2, "edit");
menu.add1(0, 3, 3, "update");
menu.add1(0, 4, 4, "clode");
return True;
StarActivity.onCreateOptionsMenu = StarActivity_onCreateOptionsMenu;
Override Function “onPrepareOptionsMenu”
The function simply returns true
to enable menu to show:
def StarActivity_onPrepareOptionsMenu(self,menu) :
return True;
StarActivity.onPrepareOptionsMenu = StarActivity_onPrepareOptionsMenu;
Override Function “onOptionsItemSelected”
def StarActivity_onOptionsItemSelected(self,menuitem) :
id = menuitem.getItemId();
if( id == 1 ) :
self.setTitle("Open Text!");
if( id == 2 ) :
self.setTitle("Edit Text!");
if( id == 3 ) :
self.setTitle("Update Text!");
if( id == 4 ) :
self.setTitle("Close Text!");
return True;
StarActivity.onOptionsItemSelected = StarActivity_onOptionsItemSelected;
Popup Window
Popup window is often used to show information or perform some interactions with customer.
Create a Button, When Clicked, We Create a Popup Window
OpenPopWindowButton = Service.ButtonClass._New(ButtonLayout);
OpenPopWindowButton.setText("Open popup window");
OpenPopWindowButton.setLinearLayoutParams(Service.WRAP_CONTENT,Service.WRAP_CONTENT);
def OpenPopWindowButton_onClick(self, Ev):
…
return;
OpenPopWindowButton.onClick = OpenPopWindowButton_onClick
In onClick Event Function, A Popup Window is Created
Because Python permits to define function in another function, we can create popup window and create functions for it.
def OpenPopWindowButton_onClick(self, Ev):
//
MyPopupWindow = Service.PopupWindowClass._New()
//
def MyPopupWindow_onDismiss(self, Ev):
Service.ToastClass._New().makeText("PopupWindow is dismiss",0).show();
return;
MyPopupWindow.onDismiss = MyPopupWindow_onDismiss;
//
PopupLayout = Service.LinearLayoutClass._New();
PopupLayout.setBackgroundColor(0xFF0000FF)
//
MyButton = Service.ButtonClass._New(PopupLayout);
def MyButton_onClick(self, Ev) :
//
Service.ToastClass._New().makeText("Button is click",0).show();
MyPopupWindow.dismiss();
return;
MyButton.onClick = MyButton_onClick;
MyButton.setText("CloseWindow");
MyButton.setLinearLayoutParams(Service.WRAP_CONTENT,Service.WRAP_CONTENT);
//
MyPopupWindow.setContentView(PopupLayout);
MyPopupWindow.setWidth(200);
MyPopupWindow.setHeight(200);
MyPopupWindow.setFocusable(True);
MyPopupWindow.showAtLocation(self,17,0,0);
//
MyPopupWindow._LockGC();
return;