Introduction
In this article, I will explore the Entity Framework with ASP.NET MVC. We will modify the form validation sample that will access the data layer using the Entity Framework.
We will create the following table in a local database using SQL Express:
CREATE TABLE [dbo].[tblComment](
[CommentID] [int] IDENTITY(1,1) NOT NULL,
[Name] [nvarchar](50) NULL,
[Email] [nvarchar](100) NULL,
[Comment] [nvarchar](100) NULL)
Now, right click on the Models directory and select Add New Item. In the dialog, select ADO.NET Entity Data Model, as shown below:
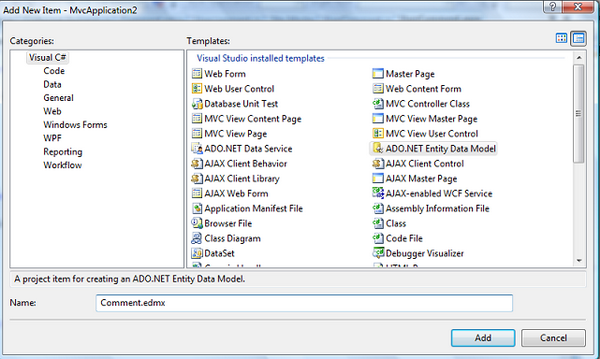
In the wizard, select "Generate from Database", and then select your database connection string. Then, select the tblComment table and the click Finish. Visual Studio will create a set of .NET classes that are custom built for accessing the database, as shown below:

Now, we will modify our controller as shown below:
[HandleError]
public class UserCommentController : Controller
{
[AcceptVerbs("GET")]
public ActionResult UserComment()
{
return View();
}
[AcceptVerbs("POST")]
public ActionResult UserComment(string name, string email, string comment)
{
ViewData["name"] = name;
ViewData["email"] = email;
ViewData["comment"] = comment;
if (string.IsNullOrEmpty(name))
ModelState.AddModelError("name", "Please enter your name!");
if (!string.IsNullOrEmpty(email) && !email.Contains("@"))
ModelState.AddModelError("email", "Please enter a valid e-mail!");
if (string.IsNullOrEmpty(comment))
ModelState.AddModelError("comment", "Please enter a comment!");
if (ViewData.ModelState.IsValid)
{
try
{
CommentEntities _db = new CommentEntities ();
tblComment commentToCreate = new tblComment();
commentToCreate.Name = name;
commentToCreate.Email = email;
commentToCreate.comment= comment;
_db.AddTotblComment(commentToCreate);
_db.SaveChanges();
}
catch
{
return View();
}
}
return View();
}
}
Summary
In this article, we explored the Entity Framework with ASP.NET MVC. In the next article, I will explore a custom IErrorDataInfo
interface.