Introduction
A scalable web application uses paging techniques to load large data. Paging enables a web-application to efficiently retrieve only the specific rows it needs from the database, and avoid having to pull back dozens, hundreds, or even thousands of results to the web-server. Today I am going to demonstrate how a custom paging can be implemented in asp.net application. It is a very essential approach to use paging technique in applications where lot of data to be loaded from database. Using a good paging technique can lead to significant performance wins within your application (both in terms of latency and throughput), and reduce the load on your database.
Background
GridView has built-in support for paging but it is very heavy and costly when it comes to deal large amount of data. The idea to develop a custom paging comes from stackoverflow website where lot of data are being divided into many page using custom paging technique very smoothly.
Using the code
First of all the big magic here is the SQL Query which makes it easy to handle the amount of data to be fetch from database. I used the NorthWind
database's Orders
table which has over 800 records. Here Index
and Size
are two integer
variables which are comes from querystring
.
- Index is the current page number
- Size is the no of records to be shown on a page
select * from (SELECT ROW_NUMBER() OVER (ORDER BY CustomerID asc) as row,* FROM Orders)
tblTemp WHERE row between (" + index + " - 1) * " + size +
" + 1 and " + index + "*" + size + " ";
SQL += " select COUNT(*) from Orders
First time when the page loads it has no querystring
values so the default Index
is 1 and Size
is 10. You can latter change the page size, there are options for 10 records per page and 15 records per page. I tried to explain all necessary points within the code.
void BindGrid(int size, int index)
{
string url = ConfigurationManager.AppSettings["URL"].ToString();
string link = "<a href='" + url +
"?Index=##Index##&Size=##Size##'><span class='page-numbers'>##Text##</span></a>";
string link_pre = "<a href='" + url +
"?Index=##Index##&Size=##Size##'><span class='page-numbers prev'>##Text##</span></a>";
string link_next = "<a href='" + url +
"?Index=##Index##&Size=##Size##'><span class='page-numbers next'>##Text##</span></a>";
try
{
String ConnStr = ConfigurationManager.ConnectionStrings["myConnection"].ToString();
String SQL = @"select * from (SELECT ROW_NUMBER() OVER (ORDER BY CustomerID asc) as row,* FROM Orders) tblTemp
WHERE row between (" + index + " - 1) * " + size +
" + 1 and " + index + "*" + size + " ";
SQL += " select COUNT(*) from Orders";
SqlDataAdapter ad = new SqlDataAdapter(SQL, ConnStr);
DataSet ds = new DataSet();
ad.Fill(ds);
this.gvPaging.DataSource = ds.Tables[0];
this.gvPaging.DataBind();
Double n = Convert.ToDouble(Convert.ToInt32(ds.Tables[1].Rows[0][0]) / size);
if (index != 1)
lblpre.Text = link_pre.Replace("##Size##", size.ToString()).Replace(
"##Index##", (index - 1).ToString()).Replace("##Text##", "prev");
else
lblpre.Text = "<span class='page-numbers prev'>prev</span>";
if (index != Convert.ToInt32(n))
lblnext.Text = link_next.Replace("##Size##", size.ToString()).Replace(
"##Index##", (index + 1).ToString()).Replace("##Text##", "next");
else
lblnext.Text = "<span class='page-numbers next'>next</span>";
int start;
if (index <= 5) start = 1;
else start = index - 4;
for (int i = start; i < start + 7; i++)
{
if (i > n) continue;
HyperLink lnk = new HyperLink();
lnk.ID = "lnk_" + i.ToString();
if (i == index)
{
lnk.CssClass = "page-numbers current";
lnk.Text = i.ToString();
}
else
{
lnk.Text = i.ToString();
lnk.CssClass = "page-numbers";
lnk.NavigateUrl = url + "?Index=" + i + "&Size=" + size + "";
}
this.pl.Controls.Add(lnk);
}
if (n > 7)
{
if (index <= Convert.ToInt32(n / 2))
{
lblLast.Visible = true;
lblIst.Visible = false;
lblLast.Text = link.Replace("##Index##", n.ToString()).Replace(
"##Size##", size.ToString()).Replace("##Text##", n.ToString());
spDot2.Visible = true;
spDot1.Visible = false;
}
else
{
lblLast.Visible = false;
lblIst.Visible = true;
lblIst.Text = link.Replace("##Index##", (n - n + 1).ToString()).Replace(
"##Size##", size.ToString()).Replace("##Text##", (n - n + 1).ToString());
spDot2.Visible = false;
spDot1.Visible = true;
}
}
}
catch (Exception ee)
{
}
}
In the above method you have to change the Config settings like the Connectionstring
and page url
which can be edited in the web.config file.
On the aspx page there are six labels
:
- previous page link
- ist page link
- dot before ist page link
- next page link
- last page link
- dot before ist page link
and a PlaceHolder
for other page numbers
<div class="pager fl">
<asp:Label runat="server" ID="lblpre"></asp:Label>
<asp:Label runat="server" ID="lblIst" Visible="false"></asp:Label>
<asp:Label runat="server" ID="spDot1" CssClass="page-numbers prev" Visible="false"
Text="..."></asp:Label>
<asp:PlaceHolder ID="pl" runat="server"></asp:PlaceHolder>
<asp:Label runat="server" ID="spDot2" Visible="false" CssClass="page-numbers prev"
Text="..."></asp:Label>
<asp:Label runat="server" ID="lblLast" Visible="false"></asp:Label>
<asp:Label runat="server" ID="lblnext"></asp:Label>
</div>
<div class="pager f2">
<a id="A1" href="javascript:void(0);" runat="server" class="page-numbers">10</a>
<a href="javascript:void(0);" id="A2" runat="server" class="page-numbers">15</a><span
class="page-numbers desc">per page</span>
</div>
HyperLinks
are dynamically created and set their Links and css
and add in the placeholder at page load.
Another important point is to replace the Table name in the sql Query and the column name in the order by
statement.
To change the page size there are two options(html anchor tags) on the right side of the page. You can set 10 records per page or 15 records per page. Using JQuery
I capture the click events of these two html anchors and set the currently set page size in a HiddenField
and reload the current page by passing Index
and Size
in querystring.
<input type="hidden" runat="server" id="hdSize" />
jQuery Code:
<script type="text/javascript">
$(document).ready(function () {
$('#A1').click(function () {
$('#hdSize').val('10');
Loadpage();
});
$('#A2').click(function () {
$('#hdSize').val('15');
Loadpage();
});
});
function Loadpage() {
try {
var url = $(location).attr('href');
var index = "Index=1";
if (url.indexOf('Index') > 0) {
index = url.split('?')[1];
index = index.split('&')[0];
}
url = url.split('?')[0] + "?" + index + "&Size=" + $('#hdSize').val() + "";
window.location.href = url;
} catch (e) {
alert(e);
}
}
</script>
CSS: CSS plays an important role in this paging. Although all works done but these all looks very poor untill a good css effect is given.
td
{
color: #55ff00;
}
.text
{
color: #74aacc;
}
.fl
{
float: left;
}
.f2
{
float: right;
padding-right: 200px;
}
.pager
{
margin-top: 20px;
margin-bottom: 20px;
}
.page-numbers
{
font-family: 'Helvetica Neue' ,Helvetica,Arial,sans-serif;
border: 1px solid #CCC;
color: #808185;
display: block;
float: left;
font-size: 130%;
margin-right: 3px;
padding: 4px 4px 3px;
text-decoration: none;
}
.page-numbers.current
{
background-color: #808185;
border: 1px solid #808185;
color: white;
font-weight: bold;
}
.page-numbers.next, .page-numbers.prev
{
border: 1px solid white;
}
.page-numbers.desc
{
border: none;
margin-bottom: 10px;
}
Screenshot
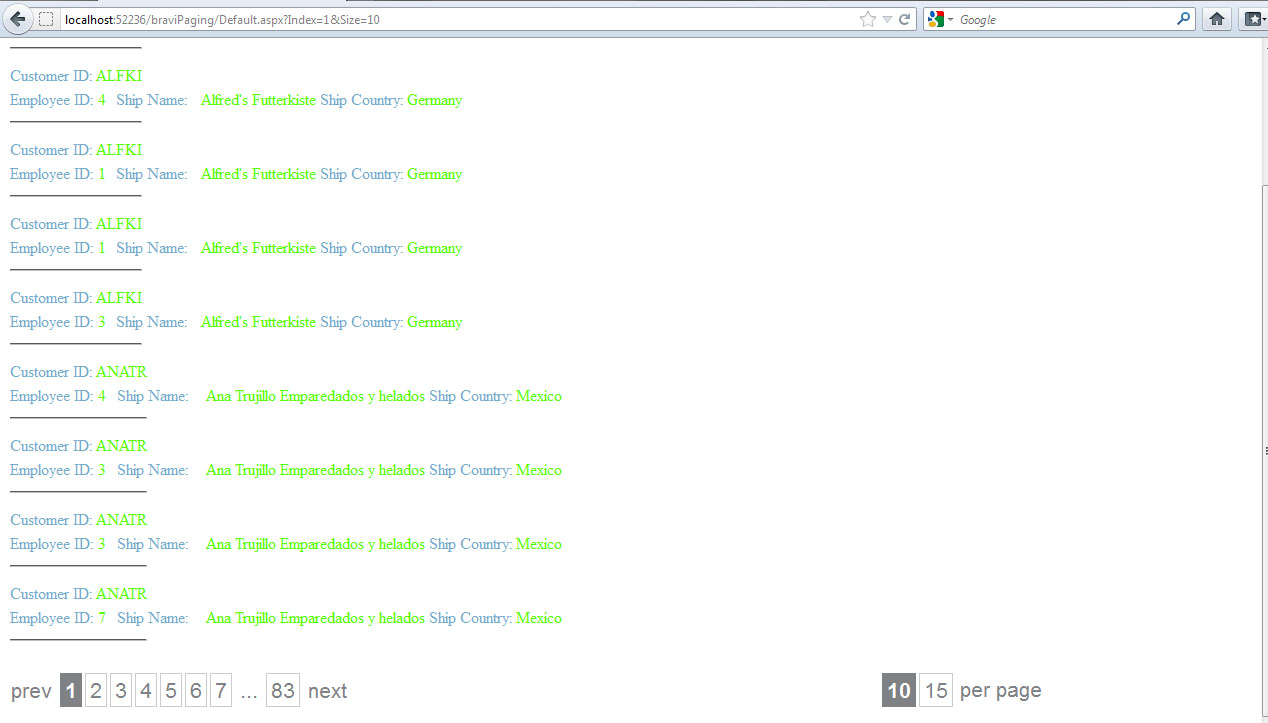
History
It took a whole week to develop this article and ist release date is 26th June 2012