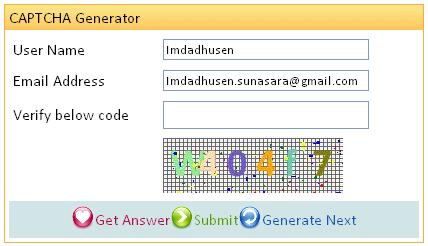
Introduction
What is CAPTCHA?
CAPTCHA is an abbreviation for Completely Automated Public Turing test to tell Computers and Humans Apart.
This method uses images of words or numbers that are, in theory, distorted and jumbled enough so that an optical character recognition program can’t read them but a human should be able to do so easily.



Background
This class is a simple one that builds:
- Six characters (using numerics 0-9, capital letters A-Z, and small letters a-z).
- Is case sensitive.
- Has dynamic font color and position.
- Provides help (can be seen clearly in the message box).
Using the Constructor
Create a CAPTCHA image using the Captcha
class. It has two constructors, with argument (defined by the user) and without (set by the system).
The following example describes how to create a captcha image with/without an argument:
Private Sub DisplayCaptcha()
Dim strFile As String = "CAPTCHA.jpg"
Dim fnt As Font = New System.Drawing.Font("Calibri", 30.0!, _
System.Drawing.FontStyle.Bold, _
System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Dim captcha = New Captcha(180, 55, fnt)
captcha.GenerateCaptcha()
captcha.CaptchaImage.Save(Server.MapPath("CAPTCHAImage/") & _
strFile, Drawing.Imaging.ImageFormat.Jpeg)
imgCaptcha.ImageUrl = "CAPTCHAImage/" & strFile
imgCaptcha.ToolTip = "Add this code into Verify textbox"
Session("CAPTCHA") = captcha.CaptchaValue
End Sub
Using the Class
- Display the captcha and verify the user input against the value stored in the class.
- Save the generated bitmap to disk in order to display it to a web user in an ASP.NET application.
- You can also regenerate the value if the user requests it.
#Region "Captcha Generation Code"
Public Sub GenerateCaptcha()
If _captchaFont Is Nothing OrElse _captchaHeight = 0 _
OrElse _captchaWidth = 0 Then
Exit Sub
End If
Dim strValue As String = ""
Dim captchaDigit As String = ""
Dim strAlphaDisplay As String = "0100110100"
captchaDigit = RandomValue.Next(10000, 1000000).ToString
_captchaImage = New Bitmap(_captchaWidth, _captchaHeight)
Using CaptchaGraphics As Graphics = Graphics.FromImage(CaptchaImage)
Using BackgroundBrush As New Drawing2D.HatchBrush(_
Drawing2D.HatchStyle.SmallGrid, Color.DimGray, Color.WhiteSmoke)
CaptchaGraphics.FillRectangle(BackgroundBrush, 0, 0, _
_captchaWidth, _captchaHeight)
End Using
Dim CharacterSpacing As Integer = (_captchaWidth \ captchaDigit.Length) - 1
Dim HorizontalPosition As Integer
Dim MaxVerticalPosition As Integer
Dim rndbBrush As New Random
Dim rndAlphabet As New Random
Dim rndAlphaDisplay As New Random
For Each CharValue As Char In captchaDigit.ToCharArray
Dim intAlphaDisplay As Integer = rndAlphaDisplay.Next(0, 9)
If strAlphaDisplay.ToCharArray(intAlphaDisplay, 1) = "1" Then
CharValue = alphabets(rndAlphabet.Next(0, 51))
End If
strValue = strValue & CharValue
Dim intRand As Integer = rndbBrush.Next(0, 139)
Dim brush As Brush = _
New SolidBrush(ColorTranslator.FromHtml(colors(intRand)))
MaxVerticalPosition = _captchaHeight - Convert.ToInt32(_
CaptchaGraphics.MeasureString(CharValue, _captchaFont).Height)
CaptchaGraphics.DrawString(CharValue, _captchaFont, brush, _
HorizontalPosition, RandomValue.Next(0, MaxVerticalPosition))
HorizontalPosition += CharacterSpacing + RandomValue.Next(-1, 1)
brush.Dispose()
Next
Dim RndmValue As New Random
Dim start As Integer = RndmValue.Next(1, 4)
For Counter As Integer = 0 To 24
CaptchaGraphics.FillEllipse(Brushes.Red, _
RandomValue.Next(start, _captchaWidth), _
RandomValue.Next(1, _captchaHeight), _
RandomValue.Next(1, 4), RandomValue.Next(2, 5))
start = RndmValue.Next(1, 4)
CaptchaGraphics.FillEllipse(Brushes.Blue, _
RandomValue.Next(start, _captchaWidth), _
RandomValue.Next(1, _captchaHeight), _
RandomValue.Next(1, 6), RandomValue.Next(2, 7))
start = RndmValue.Next(1, 4)
CaptchaGraphics.FillEllipse(Brushes.Green, _
RandomValue.Next(start, _captchaWidth), _
RandomValue.Next(1, _captchaHeight), _
RandomValue.Next(1, 4), RandomValue.Next(2, 6))
start = RndmValue.Next(1, 4)
CaptchaGraphics.FillEllipse(Brushes.Yellow, _
RandomValue.Next(start, _captchaWidth), _
RandomValue.Next(1, _captchaHeight), _
RandomValue.Next(1, 5), RandomValue.Next(2, 6))
Next
CaptchaGraphics.Flush()
_captchaFont.Dispose()
End Using
CaptchaValue = strValue
CaptchaImage = _captchaImage
End Sub
#End Region
Points of Interest
Let’s look at some of the details of this routine.
- The
HatchBrush
object is used to draw the grid background. - The characters from the randomly generated number are drawn onto the graphic one character at a time, and are randomly placed along the vertical axis and slightly off kilter on the horizontal one.
- The
FillEllipse
method of the Graphics
object is used to create noise at 50 different random points on the graphic.
Let me know if you have any questions or observations in this example by leaving me a comment.