Introduction
This is my first post on the CodeProject.
The main idea that pushed me to write this article is that I thought it might be useful to you as it was for me! First of all I searched on the net for something like this component, but unfortunately I
didn't find anything like a readymade control or component to use in a project of mine when I was in need
for it. So, I decided to stop relying on others work :p and started to make my own. The component works as the following figure shows:
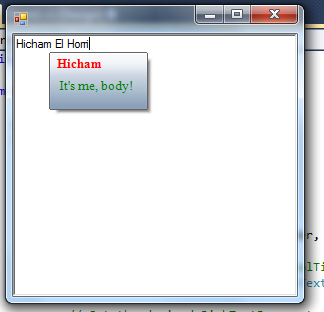
The component dependencies
The component inherits from the standard ToolTip
and relies on the following .NET methods of the
RichTextBox
control:
GetCharIndexFromPosition(Point)
GetLineFromCharIndex(int)
The component is style-able:
- You can specify your own color for the text of both; ToolTip title / ToolTip description text.
- You can specify your own font for the text for each of both; ToolTip title / ToolTip description text.
- You can set your own background bitmap.
How to use the component
Here's a step-by-step:
- On your project add a reference to the class library file "RTB_ToolTip.dll".
- On the VS designer add a new
RichTextBox
control and let's name it "richTextBox1
" - On the VS editor add the following
using
-directive:
using RTB_ToolTip;
- After the '
InitializeComponent
' or on the 'Load' event (or wherever you want) do the following:
RichTextBoxToolTip rtb = new RichTextBoxToolTip();
rtb.RichTextBox = richTextBox1;
eDictionary dict = new eDictionary();
dict.Add("Title", "Description of the ToolTip here");
dict.Add("abc", "Alphabets :)");
dict.Add("123", "Numbers ;)");
rtb.Dictionary = dict;
- Now push F5 button or click on Debug button to see your new
RichTextBox
behavior. When the window is shown, type on the richTextBox1
control something like this "Title" / "abc" / "123" (quotes are not necessary).
Now put the mouse over a word you typed and the ToolTip will show up automatically like this:

Additional info
- If you want to change ToolTip Title/Description color, do the following:
rtb.TitleBrush = Brushes.Blue;
Or:
rtb.DescriptionBrush = new SolidBrush(Color.Red);
You can also set your own pre and after text of the ToolTip title. For example:
rtb.TitlePrefix = "Definition for '";
rtb.TitleSuffix = "':";
The result would look like this:

You can access the other properties (TitleFont
/ DescriptionFont
/
BackgroundImage
...) by the same way.
For customizing the way the 'SyntaxCheking
' class determines words' start and end boundaries you can reset that in the
RichTextBoxToolTip.Chars
property. Example:
rtb.Chars = new List<Char>() {' ', '(', ')', '?'};
How does it work?
Sorry if the source code looks a little bit complicated to understand, I'll try to tell you how it works. See the following figure please:

He is a 21 year-old student studying at Mohammed I University (in Oujda - Morocco). Born in Aklim - Berkane, Morocco.
Hates discrimination with all its kinds.
He is interested in programming on Visual Studio since 2009.
Current programming languages:
+ VBScript (for both: Windows & web).
+ JScript (for both: Windows & web).
+ Visual Basic 6.0 and higher.
+ C# 2003 and higher.
+ RealBasic.
+ Html.
+ Still learning C++.