PanView is a custom control that allows you to pan a canvas or any other content from within your MainWindow
or from within any other user interface element.
Posts in this series:
A user interface element that supports panning should allow a user to swipe the content left/right with one finger, zoom in/out using two fingers, and rotate using two fingers.
Examples
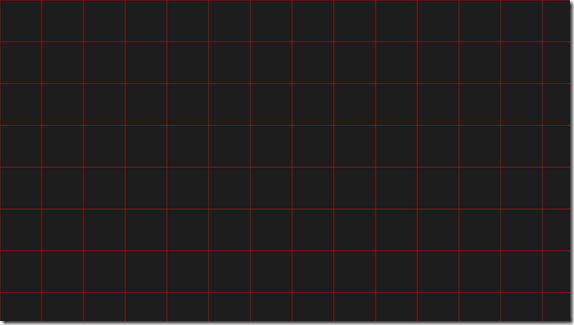
Move left/right up/down.
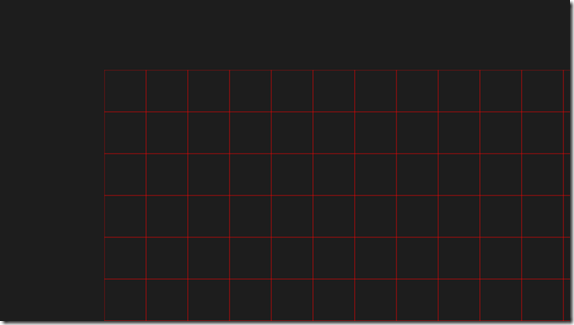
Rotate clockwise/counterclockwise.
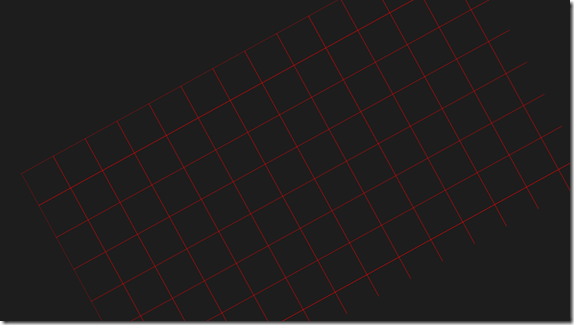
Zoom in/out.
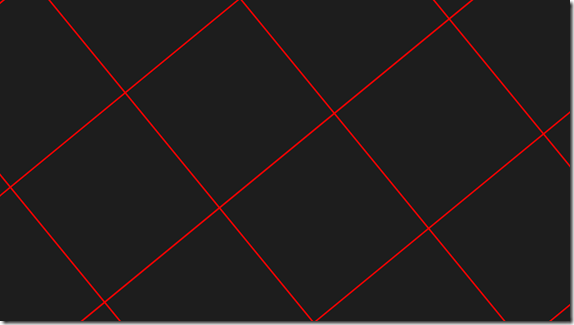
See Touch interaction design (Metro style apps) and Guidelines for Panning (Metro style apps) for more information.
My goal is to make adding panning to a C# Metro application as easy as possible. To that end, I created a custom control called PanView.
Install PanView from NuGet.org and add to your existing Metro application:
- Install the NuGet Package Manager if you have not already.
- Open your Metro application in Visual Studio.
- Select Manage NuGet Packages from the Project menu.
- Click Online. Search for PanView. Click Install.
Edit MainPage.xaml to:
- Add the
using:PanViewLibrary
. - Add the PanView custom control.
- Add content to the PanView. In the example below, the content is a
TextBlock
.
Example MainPage.xaml:
<Page
x:Class="PanViewDemoApp.MainPage"
IsTabStop="false"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:PanViewDemoApp"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:pvl="using:PanViewLibrary"
mc:Ignorable="d">
<Grid
Background="{StaticResource ApplicationPageBackgroundThemeBrush}">
<pvl:PanView>
<TextBlock
Text="This is the pannable content" />
</pvl:PanView>
</Grid>
</Page>
Limiting Manipulation Modes
You may choose to limit the types of manipulations the user may perform by setting the PanView
dependency property “ManipulationMode
”. The example below limits the panning to simple x/y translations with inertia.
MainPage.xaml
<pvl:PanView
ManipulationMode="TranslateX TranslateY TranslateInertia"
Reset
You may programmatically reset the transformations by calling PanView.Reset()
. In the XAML and C# code below, clicking the reset button resets the panning.
MainPage.xaml
<Grid Background="{StaticResource ApplicationPageBackgroundThemeBrush}">
<pvl:PanView x:Name="MyPanView">
<Grid x:Name="_grid"
Width="2000"
Height="2000" />
</pvl:PanView>
<Button
Content="Reset"
Click="OnResetClicked" />
</Grid>
MainPage.xaml.cs
private void OnResetClicked(object sender, RoutedEventArgs e)
{
MyPanView.Reset();
}
Constraining Translations
To limit the amount of translation distance, set the dependency properties MinTranslateX
, MaxTranslateX
, MinTranslateY
, and MaxTranslateY
as shown here:
MainPage.xaml
<pvl:PanView
MinTranslateX="-200"
MaxTranslateX="100"
MinTranslateY="-300"
MaxTranslateY="600"
Binaries and Source