Introduction
It's been a while since I have been working with Selenium but once I've started the main problem have risen: "in order to debug a selenium script one needs to rerun the script from the first step", well, that's borring. So I was checking for a solution to overcome this issue. RMI has come into my mind from the first of the beginning.
Background
My aim is to get the WebDriver from a different application so that every time script crashes I am able to start the debug from the last executed function. Also I want to have the posibility to develop a small script to check the page in order to find the problems in it.
I am a QTP developer and from my experience I have found that "find elements in the page" is the most frequent problem. But QTP besides Selenium has a big advantage: it can start run from any point of your script. Taking this into account I've realised that I need this QTP feature into Selenium. So starting with RMI I have made a two applications: a Server with WebDriver and a Client with Selenium scripts. The Server will hold the webdriver during the development of the testing script.
The code is written in Eclipse Juno with JRE 7 installed. I am using Maven to load the required libraries. If you are not familiar with RMI please consider the following resource before going further: http://yama-linux.cc.kagoshima-u.ac.jp/~yamanoue/researches/java/rmi-ex2/
Before starting to run the sollution, install maven plugin, here is a good tuorial: http://www.metadatatechnology.com/getting-started-with-the-fusion-components/installing-and-configuring-maven/
To run in debug mode, start the server: WebDriverWrapper has the main function impemented so you can run it as a java application.
The Server.
RMI interface:
public interface ReceiveMessageInterface extends Remote
{
void startup(String x) throws RemoteException;
public void close() throws RemoteException;
public WebElement findElement(By arg0) throws RemoteException;
public List<WebElement> findElements(By arg0) throws RemoteException;
public void get(String arg0) throws RemoteException;
public String getCurrentUrl() throws RemoteException;
public String getPageSource() throws RemoteException;
public String getTitle() throws RemoteException;
public String getWindowHandle() throws RemoteException;
public Set<String> getWindowHandles() throws RemoteException;
public Options manage() throws RemoteException;
public Navigation navigate() throws RemoteException;
public void quit() throws RemoteException;
public TargetLocator switchTo() throws RemoteException;
}
Here is the WebDriverWrapper constructor:
private WebDriverWrapper () throws RemoteException
{
try{
thisAddress= (InetAddress.getLocalHost()).toString();
}
catch(Exception e){
throw new RemoteException("can't get inet address.");
}
thisPort=3232;
System.out.println("this address="+thisAddress+",port="+thisPort);
try{
registry = LocateRegistry.createRegistry( thisPort );
registry.rebind("rmiServer", this);
}
catch(RemoteException e){
throw e;
}
}
...this script does nothing more than putting the WebDriverWrapper via ReceiveMessageInterface into JVM registry.
The Client.
In "real life testing" you can use WebDriver interface for testing:
WebDriver driver = FirefoxDriver();
In debug mode change the above line to this:
ReceiveMessageInterface driver = DriverFactory.getDriver();
On the client side we obtain the loaded driver from the server and use it via the ReceiveMessageInterface.
DriverFactory.getDriver() method is a pseudo factory fuction in this example.
Diagram
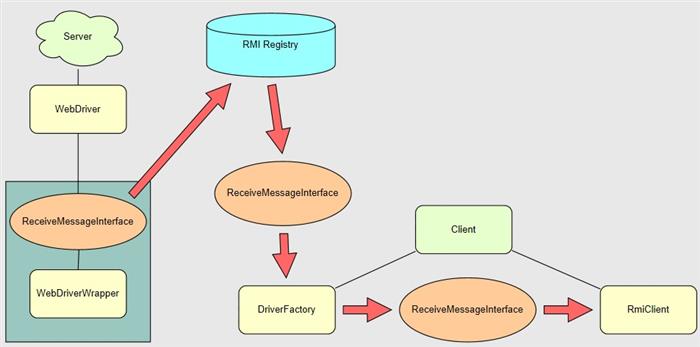
Additional comments
For each WebDriver functionality you'l need to update the RemoteMessageInterface and implement the method in the Wrapper class, in order to use it in the script.
History
Version 2