Sometimes, it is a requirement to have separate validation groups to be triggered for an action. To meet the requirement, you can simply write some JavaScript code to perform validation according to the specific condition.
For example:
function IsSearchInputValidated() {
return (ClientValidate("Search") & ClientValidate("AdvancedSearch"));
}
or:
function IsSearchInputValidated() {
return (ClientValidate("Search") && ClientValidate("AdvancedSearch"));
}
If you were to write the code in this way, you will get only 1 of the validation groups message displayed. ^^
To overcome this, we can create a helper function to make sure that all validation groups are being validated at one shot.
function ClientValidate(groupName) {
var result = new Boolean(1);
var g = groupName.split(";");
var html = new String();
for (var i = 0; i < g.length; i++) {
if (!Page_ClientValidate(g[i])) {
result = false;
if (typeof (Page_ValidationSummaries) != "undefined") {
for (var j = 0; j < Page_ValidationSummaries.length; j++) {
if (Page_ValidationSummaries[j].validationGroup == g[i]) {
html += Page_ValidationSummaries[j].innerHTML;
break;
}
}
}
}
}
if (result == false) {
if (typeof (Page_ValidationSummaries) != "undefined") {
for (var i = 0; i < Page_ValidationSummaries.length; i++) {
for (var j = 0; j < g.length; j++) {
if (Page_ValidationSummaries[i].validationGroup == g[j]) {
Page_ValidationSummaries[i].innerHTML = html;
Page_ValidationSummaries[i].style.display = "inline";
}
else {
Page_ValidationSummaries[i].innerHTML = "";
}
}
}
}
}
return result;
};
And the code to consume it will look like this:
function IsSearchInputValidated() {
var option = document.getElementById("AdvancedSearch");
if (option.checked == true) {
return ClientValidate("Search;AdvancedSearch");
}
else {
return ClientValidate("Search");
}
}
See, now we will have both validation groups message displayed.
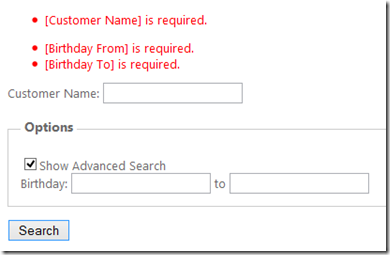