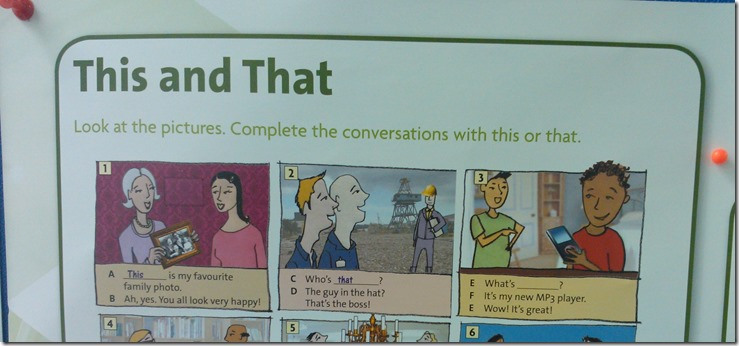
Recently, I saw this in a classroom and it reminded me of how I use it in JavaScript.
To overcome some of the requirements, we have to create client side scripting in an object oriented way. For an example, client side scripting for a custom server control. Anyways, I’m not going to show how to create a custom server control now. Perhaps, another day. 
I created a demo page for this topic. I design a JavaScript class for this page with 3 public
functions (Container
, Push
and Pop
) and 1 public
property (SequenceId
).
function Demo() {
var that = this;
this.SequenceId = 1;
Demo.prototype.Container = function () {
return $(".Container");
};
Demo.prototype.Push = function () {
this.Container().append("<div class='Item'>" + this.SequenceId + "</>");
this.SequenceId += 1;
};
Demo.prototype.Pop = function () {
if (this.Container().children().length > 0) {
this.Container().children().first().remove();
}
};
function AddHandler() {
$("#Add").click(function (s, e) {
that.Push();
});
$("#Remove").click(function (s, e) {
that.Pop();
});
}
AddHandler();
}
You can see I created a variable that
and assigned it with this
object. (In JavaScript, this
represent the current instant of the object.) This is because I’m going to re-use the functions of current instant at function body.
Let me explain this JavaScript class.
function AddHandler()
: I use this private
method to register all event handler of controls so that I can have no JavaScript in my page. In this function body, I’m going to use Push
and Pop
functions which were created as public
function. I cannot use this.Push
or this.Pop
because they will actually point to nothing since the this
object actually represents the button
control.

this.SequenceId
: This is a property of this class object. I use it to store the current running number. It will print on every item. Demo.prototype.Push
: This is a public
function so the this
object in this function body actually represents the instant of the class.

To know more about this, you can refer to Douglas Crockford’s Private Members in JavaScript.