Introduction
When your application needs to send a file to the server via FTP, you don't need a third party DLL, just a module, which allows you to send a file directly to the server on a command line. In this article, we will show how it is possible to upload a file via FTP and upload progress through a ProgressBar
and show status progress in ListBox
control.
The purpose of this article is to introduce developers to the basic routine of sending files via FTP, but it opens up numerous possibilities for the implementation of new routines, which may even create an FTP application.
Another important issue to be highlighted in this article refers to resources. The project uses five image (icons) in the resource files. These are made available in the source code file.
Requirements
- .NET Framework 4.5 or higher
System.Windows.Forms
System.Net
System.Drawing
Code
The code is divided between the Module and the Form. In the module, there are all necessary routines for simple file upload to the Server via FTP.
Module
The heart or core of the application is the mFTP
module, which contains the Routines and Functions needed for full operation. In this module, we have a Friend
Class (named Xrefs
), which allows the insertion of text, image (16x16 icon, but this dimension can be changed) and changes the color of the text, to give the user visual information and draw attention. The following colors were used in this article to inform the user of the status:
Blue
= Low Attention Status Black
= Neutral Status ForestGreen
= Good Status DeepSkyBlue
= Medium Attention Status DarkGreen
= Excellent Status Red
= Error performing operation
In addition to Friend
Class
, we have two Sub
s (pStatus
e UploadFile
) and one Function
ConvertBytes
.
1 Imports System.Net
2 Imports System.IO
3
4 Module mFTP
5 Friend Class Xrefs
6
7 Public Property Text As String
8 Public Property Image As Image
9 Public Property Color As System.Drawing.Brush
10 Public Sub New()
11
12 Me.New(Nothing, Nothing, Nothing)
13 End Sub
14 Public Sub New(ByVal Text As String)
15
16 Me.New(Text, Nothing, Nothing)
17 End Sub
18
19 Public Sub New(ByVal Text As String, _
20 ByVal Image As Image, ByVal Color As System.Drawing.Brush)
21 Me.Text = Text
22 Me.Image = Image
23 Me.Color = Color
24 End Sub
25 End Class
26
27 Public Sub UploadFile(ByVal sFileName As String, _
28 ByVal sFTPHost As String, ByVal sFTPUploadPath As String, _
29 ByVal sFTPUserName As String, ByVal sFTPPwd As String, _
30 ByRef oProgressBar As ProgressBar, ByRef oListBox As ListBox)
31 Dim oFileInfo As New System.IO.FileInfo(sFileName)
32
33 Try
34 Dim sUri As String
35
36
37 If sFTPHost.EndsWith("/") = True And _
38 sFTPUploadPath.EndsWith("/") = True Then
39 sUri = sFTPHost & sFTPUploadPath & _
40 System.IO.Path.GetFileName(sFileName.ToString)
41 ElseIf sFTPHost.EndsWith("/") = True And _
42 sFTPUploadPath.EndsWith("/") = False Then
43 sUri = sFTPHost & sFTPUploadPath & "/" & _
44 System.IO.Path.GetFileName(sFileName.ToString)
45 ElseIf sFTPHost.EndsWith("/") = False And _
46 sFTPUploadPath.EndsWith("/") = False Then
47 sUri = sFTPHost & "/" & sFTPUploadPath & "/" & _
48 System.IO.Path.GetFileName(sFileName.ToString)
49 End If
50
51 Dim oFtpWebRequest As System.Net.FtpWebRequest = _
52 CType(System.Net.FtpWebRequest.Create_
53 (New Uri(sUri)), System.Net.FtpWebRequest)
54
55
56 pStatus(oListBox, "Connecting to the server: " & _
57 sFTPHost, My.Resources.connect, Brushes.Blue)
58
59
60 pStatus(oListBox, String.Format("Host: {0} - Username: {1} - Password: {2}", _
61 sFTPHost, sFTPUserName, "******"), My.Resources.connect, Brushes.DarkBlue)
62
63
64 oFtpWebRequest.Credentials = _
65 New System.Net.NetworkCredential(sFTPUserName, sFTPPwd)
66
67
68
69 oFtpWebRequest.KeepAlive = False
70
71
72 pStatus(oListBox, "Starting the connection...", _
73 My.Resources.accept, Brushes.Black)
74
75 Application.DoEvents() : Application.DoEvents()
76
77
78 oFtpWebRequest.Timeout = 30000
79
80
81 pStatus(oListBox, "Connection accepted...", _
82 My.Resources.accept, Brushes.ForestGreen)
83
84 Application.DoEvents() : Application.DoEvents()
85
86
87 pStatus(oListBox, "Sending the file: '" & _
88 System.IO.Path.GetFileName(sFileName.ToString) & "'", _
89 My.Resources.database_lightning, Brushes.Black)
90
91
92 pStatus(oListBox, "Size: '" & _
93 ConvertBytes(oFileInfo.Length) & "'", _
94 My.Resources.database_lightning, Brushes.Black)
95
96 Application.DoEvents() : Application.DoEvents()
97
98
99 oFtpWebRequest.Method = System.Net.WebRequestMethods.Ftp.UploadFile
100
101
102 oFtpWebRequest.UseBinary = True
103
104
105 oFtpWebRequest.UsePassive = True
106
107
108 oFtpWebRequest.ContentLength = oFileInfo.Length
109
110
111
112
113 Dim buffLength As Integer = 2048
114 Dim buff(buffLength - 1) As Byte
115
116 pStatus(oListBox, "Sending the file...", _
117 My.Resources.transmit_blue, Brushes.DeepSkyBlue)
118
119
120
121 Dim oFileStream As System.IO.FileStream = oFileInfo.OpenRead()
122 Application.DoEvents() : Application.DoEvents()
123
124
125 Dim oStream As System.IO.Stream = oFtpWebRequest.GetRequestStream()
126
127
128
129 Dim contentLen As Integer = oFileStream.Read(buff, 0, buffLength)
130 Application.DoEvents() : Application.DoEvents()
131
132 oProgressBar.Maximum = oFileInfo.Length
133 Application.DoEvents() : Application.DoEvents()
134
135 Do While contentLen <> 0
136
137 oStream.Write(buff, 0, contentLen)
138 contentLen = oFileStream.Read(buff, 0, buffLength)
139 oProgressBar.Value += contentLen
140 Application.DoEvents() : Application.DoEvents()
141 Loop
142
143
144 oStream.Close()
145 oStream.Dispose()
146 oFileStream.Close()
147 oFileStream.Dispose()
148
149
150 pStatus(oListBox, "Upload successfully!", _
151 My.Resources.accept, Brushes.DarkGreen)
152 Catch ex As Exception
153 MessageBox.Show(ex.ToString, My.Application.Info.Title, _
154 MessageBoxButtons.OK, MessageBoxIcon.Error)
155
156 pStatus(oListBox, ex.Message, My.Resources.exclamation, Brushes.Red)
157 Finally
158
159 oProgressBar.Value = 0
160 End Try
161 End Sub
162 Public Sub pStatus(ByRef olbox As ListBox, ByVal sText As String, _
176 img As System.Drawing.Bitmap, lColor As System.Drawing.Brush)
177 Dim oXref As Xrefs
178 oXref = New Xrefs(sText, img, lColor)
179 olbox.Items.Add(oXref)
180 End Sub
181
187 Public Function ConvertBytes(ByVal Bytes As Long) As String
188
189 If Bytes >= 1073741824 Then
190
191 Return Format(Bytes / 1024 / 1024 / 1024, "#0.00") _
192 & " GB"
193 ElseIf Bytes >= 1048576 Then
194 Return Format(Bytes / 1024 / 1024, "#0.00") & " MB"
195 ElseIf Bytes >= 1024 Then
196 Return Format(Bytes / 1024, "#0.00") & " KB"
197 ElseIf Bytes > 0 And Bytes < 1024 Then
198
199 Return Fix(Bytes) & " Bytes"
200 Else
201 Return "0 Bytes"
202 End If
203 End Function
204 End Module
Form
The form named frmFTP
has several objects, among them Label
, TextBox
, ProgressBar
, ListBox
.
1 Imports System.IO
2
3 Public Class frmFTP
4
5 Private Sub frmFTP_Load(sender As Object, e As EventArgs) Handles MyBase.Load
6 Text = String.Format("{0} v {1}", _
7 My.Application.Info.Title, My.Application.Info.Version.ToString)
8 End Sub
9
10 Private Sub btnOpenFile_Click(sender As Object, e As EventArgs) _
11 Handles btnOpenFile.Click
12 Dim oDlg As New OpenFileDialog
13 With oDlg
14 .Title = "Open file to Upload"
15 .Filter = "All files (*.*)|*.*"
16 .ShowReadOnly = False
17 If .ShowDialog = Windows.Forms.DialogResult.OK Then
18 txtFileUpload.Text = .FileName
19 End If
20 End With
21 End Sub
22
23 Private Sub btnClose_Click(sender As Object, e As EventArgs) Handles btnClose.Click
24 Application.ExitThread()
25 Application.Exit()
26 End Sub
27
28 Private Sub btnUploadFile_Click(sender As Object, e As EventArgs) _
29 Handles btnUploadFile.Click
30
31 If CheckField(txtFTPHost, "FTP Host") = False Then Exit Sub
32 If CheckField(txtUsername, "FTP Username") = False Then Exit Sub
33 If CheckField(txtPassword, "FTP Password") = False Then Exit Sub
34 If CheckField(txtPathUpload, "FTP Path Upload") = False Then Exit Sub
35 If CheckField(txtFileUpload, "FTP File Upload") = False Then Exit Sub
36 Try
37
38 If File.Exists(txtFileUpload.Text) = False Then
39 MessageBox.Show("The file you are trying to upload via FTP _
40 does not exist or has been moved!", My.Application.Info.Title, _
41 MessageBoxButtons.OK, MessageBoxIcon.Exclamation)
42 Exit Sub
43 End If
44 lstStatus.Items.Clear()
45 UploadFile(txtFileUpload.Text, txtFTPHost.Text, _
46 txtPathUpload.Text, txtUsername.Text, txtPassword.Text, pBar, lstStatus)
47
48 Catch ex As Exception
49 MessageBox.Show(ex.ToString, My.Application.Info.Title, _
50 MessageBoxButtons.OK, MessageBoxIcon.Exclamation)
51 End Try
52 End Sub
53 Private Sub lstStatus_DrawItem(sender As Object, e As DrawItemEventArgs) _
54 Handles lstStatus.DrawItem
55 Try
56 If e.Index > -1 Then
57
58 Dim oXref As Xrefs = lstStatus.Items(e.Index)
59 e.DrawBackground()
60
61 e.Graphics.DrawImage(oXref.Image, e.Bounds.Left, e.Bounds.Top)
62
63 e.Graphics.DrawString(oXref.Text, Me.Font, oXref.Color, _
64 e.Bounds.Left + 20, e.Bounds.Top)
65 End If
66 Catch ex As Exception
67 MessageBox.Show(ex.Message, My.Application.Info.Title, _
68 MessageBoxButtons.OK, MessageBoxIcon.Hand)
69 End Try
70
71 End Sub
72 Public Function CheckField(ByRef ctl As Control, ByVal sCampo As String) As Boolean
73 Dim bResult As Boolean = False
74
75 If TypeOf ctl Is TextBox Then
76 If Trim(ctl.Text) <> String.Empty Then bResult = True
77 End If
78 If TypeOf ctl Is ComboBox Then
79 If Trim(ctl.Text) <> String.Empty Then bResult = True
80 End If
81 If TypeOf ctl Is RichTextBox Then
82 If Trim(ctl.Text) <> String.Empty Then bResult = True
83 End If
84
85
86
87
88 If bResult = False Then
89 MessageBox.Show("The field '" & sCampo & "' _
90 can't be empty!", My.Application.Info.Title, _
91 MessageBoxButtons.OK, MessageBoxIcon.Exclamation)
92 ctl.BackColor = Color.Coral
93 ctl.Focus()
94 Return bResult
95 Else
96 If TypeOf ctl Is Label Then
97 ctl.BackColor = Color.Transparent
98 Else
99 ctl.BackColor = Color.White
100 End If
101 Return bResult
102 End If
103 End Function
104
105 End Class
Screenshot
The application has a form called frmFTP
, whose screen is shown below:
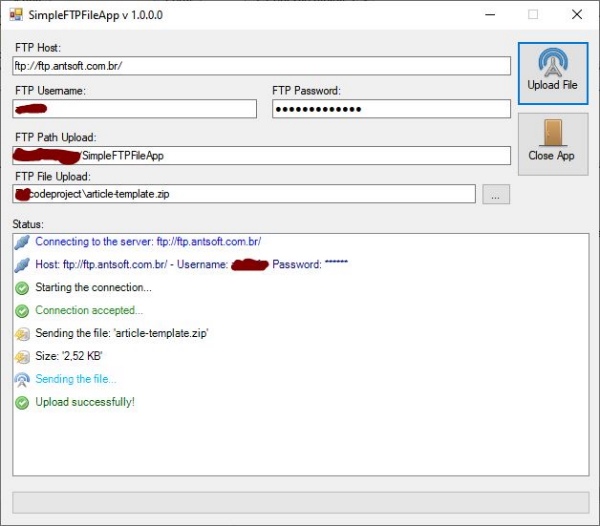
Points of Interest
In this article, you can understand how to send one file at a time to a server via FTP. In this article, it is still possible to understand some properties of the FTPWebRequest
class and the use of local Stream
and its association with WebStream
, to write the file on the server, byte by byte. It is also possible to understand the upload of the file, showing progress and the creation of a Status log, so that the user can follow the upload of the file in a step by step manner.
History
- 2019-09-27: Release version
Reference