In this post, you will learn how to generate image and add text using Python. You can see the entire source code including the code to install required library and how to use the code.
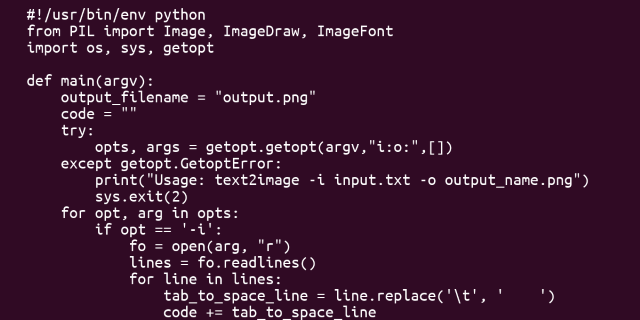
In this post, I will show you how you can generate image and add text using Python. I was working on a project that requires to generate an image for facebook og:image
(featured image) and since this project is source code sharing tool, adding a source code snippet into the featured image make sense.
I used this library on skypaste.com to generate the featured image like shown below:

This project requires Python Imaging Library (PIL). Install it by using the following command.
Installing Required Library
pip install pillow
Source Code
from PIL import Image, ImageDraw, ImageFont
import os, sys, getopt
def main(argv):
output_filename = "output.png"
code = ""
try:
opts, args = getopt.getopt(argv,"i:o:",[])
except getopt.GetoptError:
print("Parameter error")
sys.exit(2)
for opt, arg in opts:
if opt == '-i':
fo = open(arg, "r")
lines = fo.readlines()
for line in lines:
tab_to_space_line = line.replace('\t', ' ')
code += tab_to_space_line
fo.close()
os.remove(arg)
elif opt == '-o':
output_filename = arg
im = Image.new('RGBA', (1200, 600), (48, 10, 36, 255))
draw = ImageDraw.Draw(im)
try:
fontsFolder = '/usr/share/fonts/truetype'
monoFont = ImageFont.truetype(os.path.join(fontsFolder, 'UbuntuMono-R.ttf'), 18)
draw.text((10, 10), code, fill='white', font=monoFont)
except Exception as ex:
draw.text((10, 10), code, fill='white')
im.save(output_filename)
if __name__ == "__main__":
main(sys.argv[1:])
The source code is also available on Github at https://github.com/johnpili/python-text2image.
Usage
text2image -i source.cpp -o output.png
Conclusion
Learning and using Python is fun and easy. No wonder it is one of the recommended programming languages by senior developers. I will use both Python and Golang on my production projects now and in the future. Python comes with a lot of libraries that make software development simple. I recommend that you read Automate the Boring Stuff with Python, 2nd Edition: Practical Programming for Total Beginners. This book will show you a lot of examples that will increase your productivity.