This project outlines the logic necessary to write your own dialog message box in Windows. This is not meant to be a step-by-step tutorial on how to use Visual Studio or the C# programming language but an overview of the logic necessary to develop your own message box. The code snippets in the code section have lots of comments to help understand what each piece is doing.
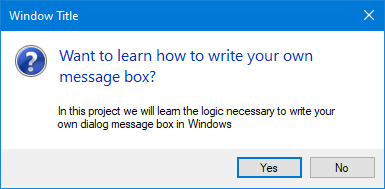
Table of Contents
- Introduction
- Before We Move Ahead
- Implementation
- Form Design
- Code
- Structure
- Events and Methods
- Conclusion
- History
Introduction
Around the time I started programming, I discovered the MessageBox class in the standard .NET Framework library. This was exciting because it allowed me to experiment with different ways of providing myself information about what was happening in my applications. This was well and good until I saw a particular message box while I was using Windows for personal use. I would eventually come to learn that this message box was actually called a TaskDialog, which was introduced in Windows Vista. It had blue text on the top and some smaller black text at the bottom. Understanding how to use a TaskDialog
at the time was beyond my skill set so I decided to try and create a similar looking one using the skills I had at the time.
In this project, we will write our own dialog message box using Visual Studio and the Designer with C#. It will support two styles of text, three button configurations, and six icon configurations. The icons utilized are from the SystemIcons
class.
Before We Move Ahead
This is not meant to be a step-by-step tutorial on how to use Visual Studio or the C# programming language but an overview of the logic necessary to develop your own message box. The code snippets in the code section have lots of comments to help understand what each piece is doing. Below is a list of topics that are assumed you are familiar with:
Implementation
Here is an implementation of the message. This code will show the message as it appears in the image at the beginning of the article.
using DialogMessage;
if (DMessage.ShowMessage(
"Window Title",
"Want to learn how to write your own message box?",
DMessage.MsgButtons.YesNo,
DMessage.MsgIcons.Question,
"In this project we will learn the logic necessary " +
"to write your own dialog message box in Windows")
== DialogResult.Yes)
MessageBox.Show("You clicked Yes!");
else
MessageBox.Show("You clicked No!");
The image below is the Form Designer view of MainForm.Designer.cs with controls and some notable properties. Important properties to notice are Anchor
, MaximumSize
, and FormBorderStyle
.
Anchor
ensures that the controls on the Form
move appropriately as it re-sizes.
MaximumSize
of the Label
s ensures that the text does not spill off the Form
and will wrap to a new line.
FormBorderStyle
set to FixedDialog
ensures that the user cannot re-size the Form
allowing it to re-size based on the amount of text provided.

Code
Structure
The message box is split up into two main files; MainForm.cs and DialogMessage.cs.
MainForm.cs contains the following Form.Load
event:
private void DialogMessage_Load(object sender, EventArgs e)
{
}
DialogMessage.cs contains the following three code blocks below; a public static
method and two enum
's:
public static DialogResult ShowMessage(string _windowTitle,
string _mainInstruction,
MsgButtons _msgButtons,
MsgIcons _msgIcons = MsgIcons.None,
string _content = "")
{
}
public enum MsgButtons
{
OK = 0,
OKCancel = 1,
YesNo = 2
}
public enum MsgIcons
{
None = 0,
Question = 1,
Info = 2,
Warning = 3,
Error = 4,
Shield = 5
}
Events and Methods
Let's go into each code block to see what it does.
Form.Load
event in MainForm.cs:
private void DialogMessage_Load(object sender, EventArgs e)
{
if (msgIcon.Visible == false)
{
mainInstruction.Location = new Point(12, mainInstruction.Location.Y);
mainInstruction.MaximumSize = new Size(353, 0);
content.Location = new Point(12, content.Location.Y);
content.MaximumSize = new Size(353, 0);
}
int mainInstructionBottom = mainInstruction.Location.Y + mainInstruction.Height;
int contentBottom = content.Location.Y + content.Height;
int contentTop = mainInstructionBottom + 18;
content.Location = new Point(content.Location.X, contentTop);
if (content.Text == string.Empty)
Height += (mainInstruction.Location.Y + mainInstruction.Height) - 50;
else
Height += (content.Location.Y + content.Height) - 60;
}
ShowMessage
method in DialogMessage.cs:
public static DialogResult ShowMessage(string _windowTitle,
string _mainInstruction,
MsgButtons _msgButtons,
MsgIcons _msgIcons = MsgIcons.None,
string _content = "")
{
MainForm main = new MainForm();
main.Height = 157;
main.Text = _windowTitle;
main.mainInstruction.Text = _mainInstruction;
main.content.Text = _content;
switch (_msgButtons)
{
case MsgButtons.OK:
main.Button1.Visible = false;
main.Button2.DialogResult = DialogResult.OK;
main.Button2.Text = "OK";
main.AcceptButton = main.Button2;
main.Button2.TabIndex = 0;
main.ActiveControl = main.Button2;
break;
case MsgButtons.OKCancel:
main.Button1.DialogResult = DialogResult.OK;
main.Button2.DialogResult = DialogResult.Cancel;
main.Button1.Text = "OK";
main.Button2.Text = "Cancel";
main.AcceptButton = main.Button2;
main.Button1.TabIndex = 1;
main.Button2.TabIndex = 0;
main.ActiveControl = main.Button2;
break;
case MsgButtons.YesNo:
main.Button1.DialogResult = DialogResult.Yes;
main.Button2.DialogResult = DialogResult.No;
main.Button1.Text = "Yes";
main.Button2.Text = "No";
main.AcceptButton = main.Button2;
main.Button1.TabIndex = 1;
main.Button2.TabIndex = 0;
main.ActiveControl = main.Button2;
break;
default:
break;
}
if (_msgIcons != MsgIcons.None)
{
main.msgIcon.Visible = true;
switch (_msgIcons)
{
case MsgIcons.Question:
main.msgIcon.Image = SystemIcons.Question.ToBitmap();
break;
case MsgIcons.Info:
main.msgIcon.Image = SystemIcons.Information.ToBitmap();
break;
case MsgIcons.Warning:
main.msgIcon.Image = SystemIcons.Warning.ToBitmap();
break;
case MsgIcons.Error:
main.msgIcon.Image = SystemIcons.Error.ToBitmap();
break;
case MsgIcons.Shield:
main.msgIcon.Image = SystemIcons.Shield.ToBitmap();
break;
default:
break;
}
}
else
{
main.msgIcon.Visible = false;
}
return main.ShowDialog();
}
Conclusion
I hope you have found this article useful. I realize that there are already some in-depth articles on CodeProject about message box alternatives and wrappers for the Windows TaskDialog (which inspired this project), however, I wanted this to be a reference for learning how to write your own.
History
- 25th April, 2020
- Added icon functionality. Icons utilized are from the
SystemIcons
class.
- 18th April, 2020
- Re-designed the code to use a
static
class and method. This eliminated the need to create a new instance of DialogMessage
.
- 13th April, 2020