This post shows a class that could be universal to any control that has some kind of image. With this class, you can add cropping to any control with image or backgroundimage.
In this post, I will show how to add cropping to any control with image or backgroundimage.
I love programming especially in graphics.
I ran into a situation where I needed to crop a simple picturebox
and it wasn't as easy as anticipated.
Then I wanted to crop a panel background image and started rewriting the code for that.
I brainstormed about how a class could be universal to any control that has some kind of image.
Voila! This class was born.
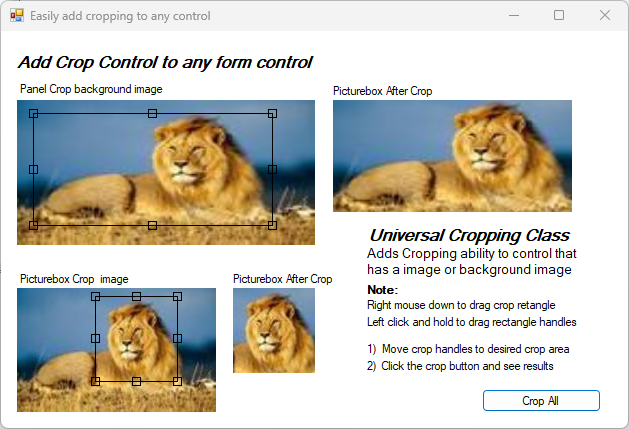
Note: The whole crop rectangle can be dragged using right mouse down and drag crop rectangle to desired are inside the control.
Use the drag by left mouse down on any of the drag handles, drag the crop rectangle in different directions.
Usage is fairly simple:
-
Create an instance of the crop class in form:
ControlCrop ControlrectPanel;
public frmCrop()
{
InitializeComponent();
ControlrectPanel = new ControlCrop(pnlCrop);
ControlrectPanel.SetControl(this.pnlCrop);
ControlrectPicturebox = new ControlCrop(picCrop);
ControlrectPicturebox.SetControl(this.picCrop);
}
-
The crop button code:
private void btnCrop_Click(object sender, EventArgs e)
{
try
{
Rectangle rectPanel = new Rectangle
(ControlrectPanel.rect.X, ControlrectPanel.rect.Y,
ControlrectPanel.rect.Width, ControlrectPanel.rect.Height);
Bitmap _pnlimg = new Bitmap(ControlrectPanel.rect.Width,
ControlrectPanel.rect.Height);
Bitmap OriginalPanelImage =
new Bitmap(pnlCrop.BackgroundImage, pnlCrop.Width, pnlCrop.Height);
using (Graphics g = Graphics.FromImage(_pnlimg))
{
g.InterpolationMode =
System.Drawing.Drawing2D.InterpolationMode.HighQualityBicubic;
g.PixelOffsetMode =
System.Drawing.Drawing2D.PixelOffsetMode.HighQuality;
g.CompositingQuality =
System.Drawing.Drawing2D.CompositingQuality.HighQuality;
g.DrawImage(OriginalPanelImage, 0, 0, rectPanel, GraphicsUnit.Pixel);
picCroppedPanel.Image = _pnlimg;
}
Rectangle rectPicturebox = new Rectangle
(ControlrectPicturebox.rect.X, ControlrectPicturebox.rect.Y,
ControlrectPicturebox.rect.Width,
ControlrectPicturebox.rect.Height);
Bitmap _pboximg = new Bitmap(ControlrectPicturebox.rect.Width,
ControlrectPicturebox.rect.Height);
Bitmap OriginalPictureboxImage = new Bitmap(picCrop.Image,
picCrop.Width, picCrop.Height);
using (Graphics gr = Graphics.FromImage(_pboximg))
{
gr.InterpolationMode =
System.Drawing.Drawing2D.InterpolationMode.HighQualityBicubic;
gr.PixelOffsetMode =
System.Drawing.Drawing2D.PixelOffsetMode.HighQuality;
gr.CompositingQuality =
System.Drawing.Drawing2D.CompositingQuality.HighQuality;
gr.DrawImage(OriginalPictureboxImage, 0, 0,
rectPicturebox, GraphicsUnit.Pixel);
picCropped.Image = _pboximg;
}
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString());
}
}