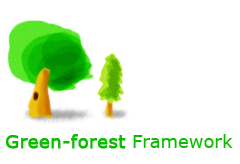
Introduction
Green-forest Framework is a simple IoC Container with Action-Handler architecture.
It's not a competitor for Spring Framework or JEE but it's a powerful addition for these frameworks. You can use Green-forest for a single class implementation
or for complex business logic.
The Action-Handler architecture can be presented by this scheme:

As you see on the scheme we divide our logic into some classes:
Action
The Action class presents an "atomic business method" and contains input and output data:
public class SomeAction extends Action<String, Integer>{
public SomeAction(String input) {
super(input);
}
}
Handler
The Handler class presents an implementation of business logic:
@Mapping(SomeAction.class)
public class SomeHandler extends Handler<SomeAction>{
@Inject
SomeService service;
public void invoke(SomeAction action) throws Exception {
String input = action.getInput();
Integer result = service.doSomeWork(input);
action.setOutput(result);
}
}
Framework
The Framework's Engine ensures functioning of handlers:
Engine engine = new Engine();
engine.putHandler(SomeHandler.class);
Integer result = engine.invoke(new SomeAction("some data"));
Motivation
What is the motivation for using the Green-forest framework? The main task is to simplify code: transfer of one big class (some service implementation) to
a set of small classes (handlers).
See this example: "Classic" implementation presents one big class in 1000 lines of code:
public class SomeBigServiceImpl implements SomeService {
public Doc createDoc(...){...}
public Doc createDocWithAttach(...){...}
public Doc getDocById(...){...}
public List getDocsList(...){...}
}
Action-Handler implementation presents a set of small classes:
public class CreateDocHandler extends Handler<CreateDoc>{
}
public class CreateDocWithAttachHandler extends Handler<CreateDocWithAttach>{
}
public class GetDocByIdHandler extends Handler<GetDoc>{
}
public class GetDocListHandler extends Handler<GetDocList>{
}
Example application
Overview
Let's review a simple example application with Green-forest framework. It presents
a web interface for users and uses a database for storage.
With this application we can create, read, update, and delete simple objects.
Here is a view of the application's web interface:

You can download binaries and sources for this example from the article's download section.
After downloading the "web-app-example.war" file, deploy it into your Web Server. This application was tested on
a Tomcat 7.0.28 web server.
Explanation of example
First we present the model class for CRUD operations - Doc:
package example.common.model;
public class Doc {
public long id;
public String name;
}
After that we create the set of actions - our business API:
src/example/common/action/
CreateDataBase.java
CreateDocs.java
GetDocsCount.java
GetDocsPage.java
RenameDoc.java
Every class of this set is an action class:
package example.common.action;
public class RenameDoc extends Action<Doc, Void> {
public RenameDoc(int id, String newName){
this(new Doc(id, newName));
}
}
So we can use these actions in our web layer:
package example.web;
public class AppServlet extends HttpServlet {
public void doPost(HttpServletRequest req, HttpServletResponse resp) {
int id = Util.tryParse(req.getParameter("id"), -1);
String newName = req.getParameter("name");
application.invoke(new RenameDoc(id, newName));
}
}
And for every action we create its own handler:
package jdbc.storage.handler;
@Mapping(RenameDoc.class)
public class RenameDocHandler extends Handler<RenameDoc>{
@Inject
Connection c;
@Override
public void invoke(RenameDoc action) throws Exception {
Doc input = action.input();
PreparedStatement ps = c.prepareStatement("UPDATE doc SET name=? WHERE id=?");
ps.setInt(2, input.id);
ps.setString(1, input.name);
ps.executeUpdate();
}
}
That's it! We receive a set of compact simple classes for API (Actions) and for implementation (Handlers).
Summary
Green-forest Framework presents
a new perspective on the development of Java applications. It's a free open-source tool which can simplify
the code of your application. This library requires minimum time to learn and is suitable for a wide range of tasks.
Happy coding with it!