This library, HigLabo.Anthropic, facilitates easy integration with Anthropic's Claude AI.It empower developers to efficiently utilize and interact with Anthropic API in C#.
Introduction
For two days, I worked on Anthropic
API and completed creating a C# library, called HigLabo.Anthropic
. You can easily call Claude AI by this library. This article will explain how to use it.
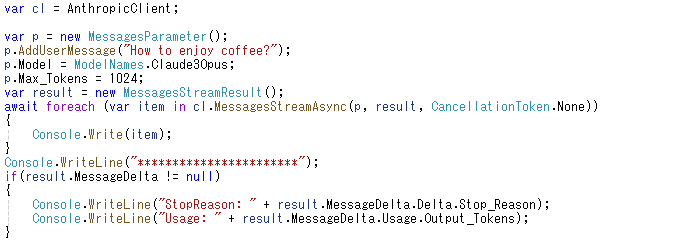
Background
Almost every day, AI provider introduces a new service. It is so fast that there is no library but only REST API endpoint. So, we must develop DIY library to consume API endpoint. I just created a library that may help someone who is planning to develop Claude API. I am sharing it here.
Download via Nuget.
HigLabo.Anthropic
All source code is on https://github.com/higty/higlabo/tree/master/Net8.
HigLabo.Anthropic
is that.
You can see the sample code here.
Using the Code
Consume Messages
endpoint receiving as stream
via server sent event.
var cl = new AnthropicClient("API KEY");
var result = new MessagesStreamResult();
await foreach (string text in cl.MessagesStreamAsync
("How to enjoy coffee?", ModelNames.Claude3Opus, result, CancellationToken.None))
{
Console.Write(text);
}
if (result.MessageDelta != null)
{
Console.WriteLine("StopReason: " + result.MessageDelta.Delta.Stop_Reason);
Console.WriteLine("Usage: " + result.MessageDelta.Usage.Output_Tokens);
}
You can pass all API parameter by using MessagesParameter
class.
var cl = new AnthropicClient("API KEY");
var p = new MessagesParameter();
p.AddUserMessage("How to enjoy coffee?");
p.Model = ModelNames.Claude3Opus;
p.Max_Tokens = 1024;
var result = new MessagesStreamResult();
await foreach (var item in cl.MessagesStreamAsync(p, result, CancellationToken.None))
{
Console.Write(item);
}
if(result.MessageDelta != null)
{
Console.WriteLine("StopReason: " + result.MessageDelta.Delta.Stop_Reason);
Console.WriteLine("Usage: " + result.MessageDelta.Usage.Output_Tokens);
}
You can get all server sent event information by MessagesStreamResult
after completion.
This is the code of function calling.
var cl = new AnthropicClient("API KEY");
var tools = new AnthropicTools();
var tool = new AnthropicTool("GetTickerSymbol",
"Gets the stock ticker symbol for a company searched by name.
Returns str: The ticker symbol for the company stock. Raises
TickerNotFound: if no matching ticker symbol is found.");
tool.Parameters.Add(new AnthropicToolParameter("company_name", "string",
"The name of company"));
tools.Add(tool);
var toolXml = tool.ToString();
var p = new MessagesParameter();
p.Messages.Add(new ChatMessage(ChatMessageRole.User,
$"What is the current stock price of Microsoft?"));
p.SetTools(tools);
p.Model = ModelNames.Claude3Opus;
p.Max_Tokens = 1024;
p.Stream = true;
var result = new MessagesStreamResult();
await foreach (var item in cl.MessagesStreamAsync(p, result, CancellationToken.None))
{
Console.Write(item);
}
var calls = AnthropicFunctionCalls.Parse(result.GetText());
if (calls.InvokeList.Count > 0)
{
Console.WriteLine();
Console.WriteLine("â– Function call list");
Console.WriteLine(calls.ToString());
var invoke = calls.InvokeList.Find(el => el.ToolName == "GetTickerSymbol");
if (invoke != null)
{
var companyName = invoke.GetParameterValue("company_name") ?? "";
var tickerSymbol = GetTickerSymbol(companyName);
}
}
This is the code to send image and get explanation.
var p = new MessagesParameter();
p.Model = "claude-3-opus-20240229";
p.Max_Tokens = 1024;
var msg = new ChatImageMessage(ChatMessageRole.User);
msg.AddTextContent($"What is this image include?");
msg.AddImageFile(Path.Combine(Environment.CurrentDirectory, "Image", "Rock.jpg"));
p.Messages.Add(msg);
p.Stream = true;
var result = new MessagesStreamResult();
await foreach (var item in cl.MessagesStreamAsync(p, result, CancellationToken.None))
{
Console.Write(item);
}
Points of Interest
All class design architecture is the same as HigLabo.OpenAI
. Please see this article.
You can use the same as HigLabo.OpenAI
. HigLabo.Anthropic
has:
AnthropicClient
XXXParameter
XXXAsync
XXXResponse
RestApiResponse
classes and the behavior is the same. This proves that the class architecture design is absolutely well for REST API client library. Actually, I finished 6 hours work to implement the entire code of HigLabo.Anthropic
. You can learn how to design class and library from these two library source code and if you have a plan to implement some REST API client library, it may help you.
History
- 17th March, 2024: Initial release of
HigLabo.Anthropic