This article explores how a beginner-friendly console application is developed using C# and the .NET Framework 4.8. We delve into the intricacies of creating a menu-driven interface that offers choices such as Eat Candy, Go Fishing, and Play Basketball, culminating in an option to exit the application gracefully. Through a step-by-step walkthrough, we illustrate the process of writing clean, concise code while emphasizing best practices in user interaction.
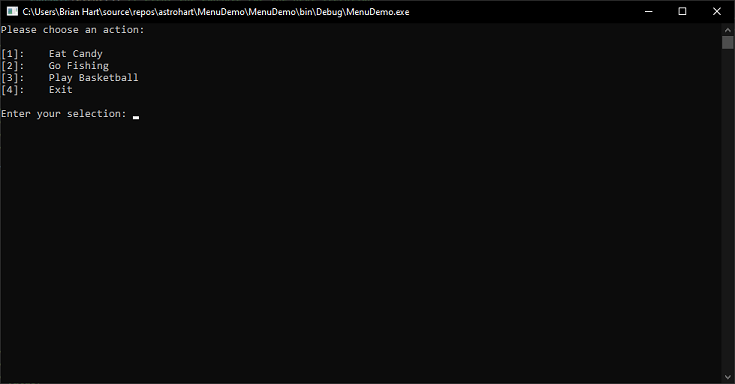
Figure 1. A screenshot of the console application displaying a menu of choices.
Contents
This tutorial will create a simple console application using C# and the .NET Framework 4.8. Our application will display a menu of choices to the user, allowing them to perform various actions. We'll implement features such as displaying the menu, handling user input, and confirming actions like exiting the application.
This tip/trick is aimed at beginners and those just beginning to do C# programming language exercises, such as first-year computer science students. Such a problem is frequently given to those learning to program for the first time.
NOTE: I am aware of the existence of C# 12 and .NET Core 8. However, as a personal preference, I believe that using older frameworks has more instructional value. I am specifically choosing to use .NET Framework 4.8 and C# 7 to show students programming concepts such as namespace
s, etc., that must be more explicitly declared in older versions of C#. Furthermore, as this is not a very advanced tutorial, we can forego using a powerhouse such as .NET Core 8 when .NET Framework on Windows will do just fine, for my purposes.
- Visual Studio 2022 is installed on your machine.
- Basic understanding of C# programming concepts.
- Open Visual Studio 2022.
- Click the File menu, point to New, and click Project/Solution.
- In the Create a new project window, search for
Console App (.NET Framework)
". - Choose the
Console App (.NET Framework)
template and click Next. - Name your project
MenuDemo
and choose a location to save it. - Click Create to create the project.
-
Inside the MenuDemo
project, add a new C# file named Enums.cs
.
-
Define an enum named MenuChoices
to represent the menu options. Include options for EatCandy
, GoFishing
, PlayBasketball
, and Exit
. Use the Description
attribute to provide human-readable descriptions for each option.
using System.ComponentModel;
namespace MenuDemo
{
public enum MenuChoices
{
[Description("Eat Candy")]
EatCandy,
[Description("Go Fishing")]
GoFishing,
[Description("Play Basketball")]
PlayBasketball,
[Description("Exit")]
Exit
}
}
Listing 1. The definition of the MenuChoices
enumeration.
In C#, an enum
(short for "enumeration") is a special data type used to define a set of named integral constants. These constants represent a finite list of possible values that a variable of that enum
type can hold. Each constant in an enum
has an associated integer value, which by default starts from 0 and increments by 1 for each subsequent constant.
In our MenuDemo
application, we're using a enum
called MenuChoices
to represent the different options available in our menu. Instead of using raw integer values to represent each choice (e.g., 0 for "Eat Candy", 1 for "Go Fishing", and so on), we use descriptive names like EatCandy
, GoFishing
, etc., making our code more readable and self-explanatory.
Here are some benefits of using an enum
:
-
Readability: Using descriptive names improves code readability. When someone reads MenuChoices.EatCandy
, they immediately understand the intended meaning.
-
Type Safety: Enums provide type safety, meaning you can't assign arbitrary values to an enum variable. You're restricted to the predefined set of constants.
-
Intellisense Support: IDEs like Visual Studio provide IntelliSense support for enums, making selecting the correct value from a list of options easier.
-
Compile-Time Checks: The compiler performs checks to ensure that enum values are used correctly, reducing the likelihood of errors at runtime.
In summary, enums are a convenient way to define a set of related constants with meaningful names, improving code readability, maintainability, and reliability. They are particularly useful in scenarios where you have a fixed set of options or states, such as menus, application states, days of the week, etc.
-
Open Solution Explorer.
-
Expand all the items in Solution Explorer, find the Program.cs
file, and double-click on it.
-
Somewhere in the Program
class, after the Main
method, add code to define the GetUserChoice
method.
-
Now, add code for the definition of the GetEnumDescription method:
-
Define the DisplayMenu
method:
-
Define the Main
Method:
Here's the entire Program.cs
file with all the methods combined:
using System;
using System.ComponentModel;
namespace MenuDemo
{
public static class Program
{
public static void Main()
{
while (true)
{
DisplayMenu();
var choice = GetUserChoice();
var choiceIndex = (int)choice - 1;
if (choiceIndex >= 0 && choiceIndex < Enum
.GetValues(typeof(MenuChoices))
.Length)
switch (choiceIndex)
{
case (int)MenuChoices.EatCandy:
Console.WriteLine("You chose to Eat Candy.");
break;
case (int)MenuChoices.GoFishing:
Console.WriteLine("You chose to Go Fishing.");
break;
case (int)MenuChoices.PlayBasketball:
Console.WriteLine("You chose to Play Basketball.");
break;
case (int)MenuChoices.Exit:
Console.Write(
"Are you sure you want to exit the application? (Y/N): "
);
var confirmation = Console.ReadLine()
.ToUpper()[0];
Console.WriteLine();
if (confirmation == 'Y')
{
Console.WriteLine("Exiting the application...");
return;
}
Console.Clear();
continue;
default:
Console.WriteLine(
"Invalid choice. Please try again."
);
break;
}
else
Console.WriteLine("Invalid choice. Please try again.");
Console.WriteLine("Press any key to continue...");
Console.ReadKey();
Console.Clear();
}
}
private static void DisplayMenu()
{
Console.WriteLine("Please choose an action:\n");
var menuItemNumber = 1;
foreach (MenuChoices choice in Enum.GetValues(typeof(MenuChoices)))
if (choice != MenuChoices.Unknown)
{
var description = GetEnumDescription(choice);
Console.WriteLine($"[{menuItemNumber}]: {description}");
menuItemNumber++;
}
Console.Write("\nEnter your selection: ");
}
private static string GetEnumDescription(Enum value)
{
var field = value.GetType()
.GetField(value.ToString());
var attribute = (DescriptionAttribute)Attribute.GetCustomAttribute(
field, typeof(DescriptionAttribute)
);
return attribute == null ? value.ToString() : attribute.Description;
}
private static MenuChoices GetUserChoice()
{
var input = Console.ReadLine();
return Enum.TryParse(input, out MenuChoices choice)
? choice
: MenuChoices.Unknown;
}
}
}
Listing 6. The final content of our Program.cs
file.
- Press
F5
on the keyboard, or click the Debug menu on the menu bar, and then click Start Debugging to run the application.
- Visual Studio should automatically build your application into a
.exe
file, and then launch that .exe
file for you. - What you see should resemble -- although it may not look exactly the same -- Figure 1.
- Follow the on-screen instructions to interact with the menu.
- Test each menu option and verify that the application behaves as expected.
Congratulations! You've successfully created a menu-driven console application in C# using .NET Framework 4.8. You've learned to display a menu, handle user input, and implement features like confirming actions before execution. Keep exploring and experimenting with C# to enhance your programming skills further.