A practical guide to building an Asp.Net 8 MVC application that uses jQuery component DataTables.net. This is a continuation of article Part6.
1 ASP.NET8 using jQuery DataTables.net
I was evaluating the jQuery DataTables.net component [1] for usage in ASP.NET8 projects and created several prototype (proof-of-concept) applications that are presented in these articles.
1.1 Articles in this series
Articles in this series are:
- ASP.NET8 using DataTables.net – Part1 – Foundation
- ASP.NET8 using DataTables.net – Part2 – Action buttons
- ASP.NET8 using DataTables.net – Part3 – State saving
- ASP.NET8 using DataTables.net – Part4 – Multilingual
- ASP.NET8 using DataTables.net – Part5 – Passing additional parameters in AJAX
- ASP.NET8 using DataTables.net – Part6 – Returning additional parameters in AJAX
- ASP.NET8 using DataTables.net – Part7 – Buttons regular
- ASP.NET8 using DataTables.net – Part8 – Select rows
- ASP.NET8 using DataTables.net – Part9 – Advanced Filters
2 Final result
The goal of this article is to create a proof-of-concept application that demos DataTables.net component using regular predefined buttons. Let us present the result of this article.
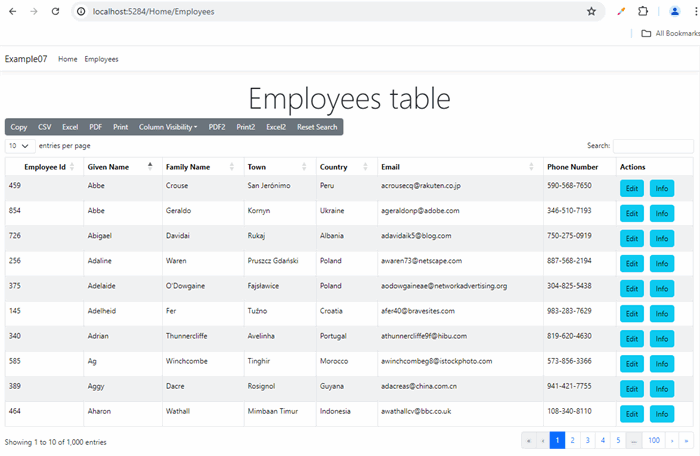

The point is, the component DataTables.net lets you activate some predefined buttons via JavaScript configuration, without the need to code anything.
Typical functionalities are exporting table data to copy-to-clipboard, CSV file, Excel, PDF, and Print.
Then you can choose which table columns should be visible if your screen is not big enough.
Then we show how to control to export only visible table columns to Excel, PDF, and Print.
Then we show how to create the button to reset the search field.
3 Client-side DataTables.net component
Here I will just show what the ASP.NET view using DataTables component looks like.
<!-- Employees.cshtml -->
<partial name="_LoadingDatatablesJsAndCss" />
@{
<div class="text-center">
<h3 class="display-4">Employees table</h3>
</div>
<!-- Here is our table HTML element defined. JavaScript library Datatables
will do all the magic to turn it into interactive component -->
<table id="EmployeesTable01" class="table table-striped table-bordered ">
</table>
}
<script>
document.addEventListener("DOMContentLoaded", InitializeDatatable);
function InitializeDatatable() {
$("#EmployeesTable01").DataTable({
processing: true,
paging: true,
info: true,
ordering: true,
searching: true,
search: {
return: true
},
autoWidth: true,
lengthMenu: [10, 15, 25, 50, 100],
pageLength: 10,
order: [[1, 'asc']],
serverSide: true,
stateSave: true,
stateDuration: -1,
ajax: {
"url": "@Url.Action("EmployeesDT", "Home")",
"type": "POST",
"datatype": "json"
},
columns: [
{
name: 'id',
data: 'id',
title: "Employee Id",
orderable: true,
searchable: false,
type: 'num',
visible: true
},
{
name: 'givenName',
data: "givenName",
title: "Given Name",
orderable: true,
searchable: true,
type: 'string',
visible: true
},
{
name: 'familyName',
data: "familyName",
title: "Family Name",
orderable: true,
searchable: true,
type: 'string',
visible: true
},
{
name: 'town',
data: "town",
title: "Town",
orderable: true,
searchable: true,
type: 'string',
visible: true
},
{
name: 'country',
data: "country",
title: "Country",
orderable: true,
searchable: true,
type: 'string',
visible: true
},
{
name: 'email',
data: "email",
title: "Email",
orderable: true,
searchable: true,
type: 'string',
visible: true
},
{
name: 'phoneNo',
data: "phoneNo",
title: "Phone Number",
orderable: false,
searchable: true,
type: 'string',
visible: true
},
{
name: 'actions',
data: "actions",
title: "Actions",
orderable: false,
searchable: false,
type: 'string',
visible: true,
render: renderActions
},
{
name: 'urlForEdit',
data: "urlForEdit",
title: "urlForEdit",
orderable: false,
searchable: false,
type: 'string',
visible: false,
className: 'FixedVisibility'
}
],
layout: {
top1Start: {
buttons:
['copy', 'csv', 'excel', 'pdf', 'print',
{
extend: 'colvis',
text: 'Column Visibility',
columns: ':not(.FixedVisibility)',
},
{
extend: 'pdfHtml5',
text: 'PDF2',
orientation: 'landscape',
pageSize: 'A4',
exportOptions: {
columns: ':visible'
}
},
{
extend: 'print',
text: 'Print2',
exportOptions: {
columns: ':visible'
}
},
{
extend: 'excel',
text: 'Excel2',
exportOptions: {
columns: ':visible'
}
},
{
text: 'Reset Search',
action: ResetSearch
}
]
}
}
});
function renderActions(data, type, row, meta) {
let html1 =
'<a class="btn btn-info" href="' +
row.urlForEdit + '">Edit</a>';
let infoUrl = "@Url.Action("EmployeeInfo", "Home")" +
"?EmployeeId=" + row.id;
let html2 =
'<a class="btn btn-info" style="margin-left: 10px" href="' +
infoUrl + '">Info</a>';
return html1 + html2;
}
function ResetSearch(e, dt, node, config) {
dt.search('').draw();
};
}
</script>
Note the layout property we used to define all the buttons in this example.
More about these properties can be found in the manual at [1]. The application here is just a proof of concept for ASP.NET environment.
4 Conclusion
The full example code project can be downloaded.
5 References
[1] https://datatables.net/
[21] ASP.NET8 using DataTables.net – Part1 – Foundation
https://www.codeproject.com/Articles/5385033/ASP-NET-8-Using-DataTables-net-Part1-Foundation
[22] ASP.NET8 using DataTables.net – Part2 – Action buttons
https://www.codeproject.com/Articles/5385098/ASP-NET8-using-DataTables-net-Part2-Action-buttons
[23] ASP.NET8 using DataTables.net – Part3 – State saving
https://www.codeproject.com/Articles/5385308/ASP-NET8-using-DataTables-net-Part3-State-saving
[24] ASP.NET8 using DataTables.net – Part4 – Multilingual
https://www.codeproject.com/Articles/5385407/ASP-NET8-using-DataTables-net-Part4-Multilingual
[25] ASP.NET8 using DataTables.net – Part5 – Passing additional parameters in AJAX
https://www.codeproject.com/Articles/5385575/ASP-NET8-using-DataTables-net-Part5-Passing-additi
[26] ASP.NET8 using DataTables.net – Part6 – Returning additional parameters in AJAX
https://www.codeproject.com/Articles/5385692/ASP-NET8-using-DataTables-net-Part6-Returning-addi
[27] ASP.NET8 using DataTables.net – Part7 – Buttons regular
https://www.codeproject.com/Articles/5385828/ASP-NET8-using-DataTables-net-Part7-Buttons-regula
[28] ASP.NET8 using DataTables.net – Part8 – Select rows
https://www.codeproject.com/Articles/5386103/ASP-NET8-using-DataTables-net-Part8-Select-rows
[29] ASP.NET8 using DataTables.net – Part9 – Advanced Filters
https://www.codeproject.com/Articles/5386263/ASP-NET8-using-DataTables-net-Part9-Advanced-Filte
Mark Pelf is the pen name of just another Software Engineer from Belgrade, Serbia.
My Blog https://markpelf.com/