This article is a demonstration of generating and reading QR codes. With this article, I wish to demonstrate how a QR code can be generated interactively by taking input from the user, as well as read text from a QR code saved as an image on the disk. The example is implemented as a Java Maven project.
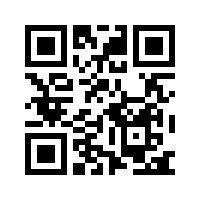
Introduction
The use of QR codes also known as Quick Response codes has become a standard in many areas. The most common use of QR codes currently is in making online shopping easier. The ZXing or "Zebra Crossing" library can be used to process QR codes easily.
Background
I wish to keep the article short and interesting, so the reader remains focused on the required aspects of the application and not divulge into unnecessary details.
I have created a Console Java Maven Project having three options as follows:
- Generate QR Code
- View QR Code
- Extract information from QR Code
Using the code
The following code is used in the pom.xml to add the Maven dependency for the ZXing library.
<dependencies>
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>3.3.0</version>
</dependency>
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>javase</artifactId>
<version>3.3.0</version>
</dependency>
</dependencies>
The Java code to generate a QR code is very short and self-explanatory. It uses stream classes to take input in a string and generate a QR code using the QRCodeWriter, BitMatrix and MatrixToImageWriter classes of the ZXing library.
The following code can be used to generate a QR code from text entered interactively by the user:
try
{
InputStreamReader reader = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(reader);
System.out.println("Enter some text and terminate with blank line...");
String text=new String();
String line = br.readLine();
while(line.trim().length() != 0)
{
text += line + "\n";
line = br.readLine();
}
System.out.println(text);
QRCodeWriter writer = new QRCodeWriter();
BitMatrix matrix = writer.encode(text, BarcodeFormat.QR_CODE, 200, 200);
Path path = Paths.get("5387296/sample.png");
MatrixToImageWriter.writeToPath(matrix, "png", path);
System.out.println("QR Code generated in " + path + ".");
}
catch(IOException ex)
{
System.out.println("I/O Error: " + ex.getMessage());
}
catch(WriterException ex)
{
System.out.println("Writer Error: " + ex.getMessage());
}
The above code generated the following QR code.
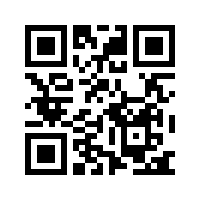
The following code can be used to read and extract text from a QR code saved as a PNG image, using the LuminanceSource, BinaryBitmap and Result classes and the Reader interface of the ZXing library:
try
{
BufferedImage image = ImageIO.read(new File("5387296/sample.png"));
LuminanceSource source = new BufferedImageLuminanceSource(image);
BinaryBitmap bitmap = new BinaryBitmap(new HybridBinarizer(source));
Reader reader = new MultiFormatReader();
Result result = reader.decode(bitmap);
System.out.println("The QR Code contains the following information: ");
System.out.println(result.getText());
}
catch(IOException ex)
{
System.out.println("I/O Error: " + ex.getMessage());
}
catch(NotFoundException ex)
{
System.out.println("Not Found Error: " + ex.getMessage());
}
catch(ChecksumException ex)
{
System.out.println("Checksum Error: " + ex.getMessage());
}
catch(FormatException ex)
{
System.out.println("Format Error: " + ex.getMessage());
}
The above code displays the following text:
Code Project
is awesome.
The generated QR code can be viewed in the default image viewer using the following code:
String commands[] = {"cmd","/c","start","c:/QRApp/sample.png"};
Runtime.getRuntime().exec(commands);
Options can be chosen from the following menu, which appears on running the program:
--------------------------------------------------
Choose an option
--------------------------------------------------
1. Generate QR Code
2. View QR Code
3. Extract information from QR Code
4. Exit
--------------------------------------------------
Enter an option:
--------------------------------------------------
Points of Interest
This article is relatively short, but I hope users find it interesting as well as informative.