In this article, we explore how four leading AI platforms - OpenAI, Groq, Gemini, and Mistral handle JSON formatting. This knowledge is key to getting clean, structured data from as responses from these platforms.
Introduction
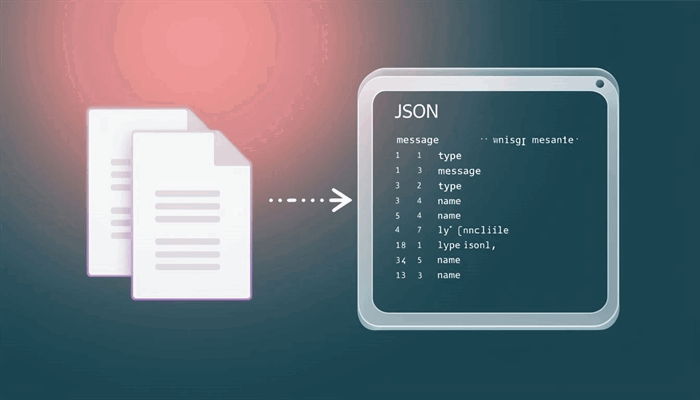
In this article, we'll explore how four leading AI platforms - OpenAI, Groq, Gemini, and Mistral handle response in JSON format. This knowledge is key to getting clean, structured data from AI responses.
Why is this important? JSON is one of the most widely used formats for data exchange between applications. With Structured Outputs, you can ensure AI responses always follow your specified JSON Schema, avoiding issues like missing keys or invalid values.
- Extracting data effortlessly
- Formulating precise queries
- Displaying model outputs with maximum control in your UI
Whether you're a seasoned developer or just starting with AI integration, this guide will help you master JSON in AI platforms, making your applications more reliable and efficient.
JSON and Its Importance in AI APIs
JSON, or JavaScript Object Notation, is like a universal language for data. Imagine it as a way to organize information in a format that both humans and computers can easily read. Here's why it's becoming a big deal in AI:
- Easy to Read: Both humans and machines can understand it quickly.
- Flexible: It can handle complex data structures without breaking a sweat.
- Language-Friendly: Most programming languages can work with JSON out of the box.
- Lightweight: It doesn't add unnecessary bulk to your data.
{
"name": "John Doe",
"age": 30,
"skills": ["Python", "AI", "JSON"]
}
AI Platforms Love JSON
AI platforms are embracing JSON because:
- It makes integrating AI into apps much smoother.
- Can specify exactly what data you want and how you want it.
- It reduces errors and misunderstandings between the AI and your application.
Best Practices for Getting JSON from LLMs
When you're trying to get JSON data from AI APIs, there are some tricks that can make your life a whole lot easier. By following these tips, you'll be a pro at getting clean, useful JSON from AI in no time!

Platform-Specific Guides
OpenAI - Structured Outputs for JSON
OpenAI offers two powerful methods for generating structured JSON responses: JSON mode and the more advanced Structured Outputs feature. While JSON mode ensures responses are in a JSON format, Structured Outputs takes it a step further by guaranteeing adherence to a specific JSON schema. This newer feature, available on the latest models like GPT-4 and its variants, provides developers with precise control over the structure of AI-generated content. By using Structured Outputs, you can define exact schemas for your desired JSON responses, significantly reducing the need for post-processing and validation.
How to use Structured Output
- Define Your Schema: Use Pydantic (Python) or Zod (JavaScript) to define your data structure.
Python pydantic:
class BookReview(BaseModel):
title: str
author: str
rating: int
summary: str
tags: List[str]
class Books(BaseModel):
book_reviews: List[BookReview]
Javascript Zod:
const WeatherForecast = z.object({
location: z.string(),
date: z.string(),
temperature: z.number(),
conditions: z.string(),
precipitation: z.number(),
})
- Install required packages: pydantic, openai
- Make the API Call: Use the parse method in the OpenAI SDK to get structured responses.
- Handle the Response: The API returns parsed data matching your schema.
Example in Python:
from pydantic import BaseModel
from openai import OpenAI
client = OpenAI(
api_key="OPENAI API key"
)
class CalendarEvent(BaseModel):
name: str
date: str
participants: list[str]
completion = client.beta.chat.completions.parse(
model="gpt-4o-2024-08-06",
messages=[
{"role": "system", "content": "Extract the event information."},
{"role": "user", "content": "Alice and Bob are going to a science fair on Friday."},
],
response_format=CalendarEvent,
)
event = completion.choices[0].message.parsed
print(event)
print(event.name)
Example in Javascript:
import OpenAI from "openai";
import { zodResponseFormat } from "openai/helpers/zod";
import { z } from "zod";
const openai = new OpenAI({
apiKey: OPENAI_API_KEY
});
const CalendarEvent = z.object({
name: z.string(),
date: z.string(),
participants: z.array(z.string()),
});
const completion = await openai.beta.chat.completions.parse({
model: "gpt-4o-2024-08-06",
messages: [
{ role: "system", content: "Extract the event information." },
{ role: "user", content: "Alice and Bob are going to a science fair on Friday." },
],
response_format: zodResponseFormat(CalendarEvent, "event"),
});
const event = completion.choices[0].message.parsed;
console.log(event)
Important Notes:
- Available in GPT-4o models (gpt-4o-mini-2024-07-18 and later)
- Use for structuring model responses to users, not for function calling
- All fields in your schema must be required
By using Structured Outputs, you can ensure that your AI responses are always in the format you need, making your applications more robust and easier to develop.
Groq - JSON mode
Groq offers a "JSON mode" that ensures all chat completions are valid JSON. Here's how you can use it effectively:
Key Features:
- Guaranteed Valid JSON: All responses in JSON mode are valid JSON.
- Pretty-Printed JSON: Recommended for best results.
- Model Performance: Mixtral > Gemma > Llama for JSON generation.
How to Use JSON Mode:
- Enable JSON Mode: Set "response_format": {"type": "json_object"} in your chat completion request.
- Describe JSON Structure: Include a description of the desired JSON structure in the system prompt.
- Handle Errors: Groq returns a 400 error with code json_validate_failed if JSON generation fails.
Example in Python:
from typing import List, Optional
import json
from pydantic import BaseModel
from groq import Groq
groq = Groq(
api_key=GROQ_API_KEY
)
class Ingredient(BaseModel):
name: str
quantity: str
quantity_unit: Optional[str]
class Recipe(BaseModel):
recipe_name: str
ingredients: List[Ingredient]
directions: List[str]
chat_completion = groq.chat.completions.create(
messages=[
{
"role": "system",
"content": "You are a recipe database that outputs recipes in JSON.\n The JSON object must use the schema: {json.dumps(Recipe.model_json_schema(), indent=2)}"
},
{
"role": "user",
"content": "Fetch a recipe for apple pie"
}
],
model="llama3-8b-8192",
temperature=0,
stream=False,
response_format={"type": "json_object"}
)
recipe = Recipe.model_validate_json(chat_completion.choices[0].message.content)
print(recipe)
Important Notes:
- JSON mode does not support streaming.
- Keep prompts concise for best results.
- Use Pydantic models to define and validate your JSON schema.
By using JSON mode, you can ensure that your Groq API responses are always in a valid JSON format, making it easier to integrate AI-generated content into your applications.
Gemini - JSON output
Google's Gemini API offers powerful capabilities for generating structured JSON outputs, ideal for applications requiring standardized data formats.
Key Features:
- Configurable Output: Gemini can be set to produce JSON-formatted responses.
- Schema Definition: Supports defining JSON schemas for consistent output structure.
- Flexible Implementation: Works with both Gemini 1.5 Flash and Gemini 1.5 Pro models.
How to Structure Prompts for JSON:
- Specify Format in Prompt: Clearly describe the desired JSON structure in your prompt.
- Use Schema Definition: For Gemini 1.5 Pro, use the response_schema field for more precise control.
Python Code Example:
import google.generativeai as genai
import os
import typing_extensions as typing
# Configure the API
genai.configure(api_key=API_KEY)
# Define the JSON schema
class Recipe(typing.TypedDict):
recipe_name: str
# Initialize the model
model = genai.GenerativeModel('gemini-1.5-pro',
generation_config={
"response_mime_type": "application/json",
"response_schema": list[Recipe]
})
# Generate JSON content
prompt = "List 5 popular cookie recipes"
response = model.generate_content(prompt)
print(response.text)
Best Practices:
- Clear Schema Definition: Always define the expected JSON structure clearly.
- Use Appropriate Model: Choose between Gemini 1.5 Flash and Pro based on your needs.
- Validate Output: Always validate the received JSON to ensure it meets your requirements.
- Error Handling: Implement robust error handling for cases where JSON generation might fail.
- Iterative Refinement: Test and refine your prompts to achieve the desired output consistency.
Mistral - JSON output
Mistral offers a straightforward approach to generating structured JSON outputs, making it ideal for applications requiring standardized data formats.
Mistral's Approach to JSON Formatting:
- JSON Mode: Enable by setting response_format to {"type": "json_object"} in API requests.
- Explicit Instructions: Always include a clear request for JSON output in your prompt.
- Model Compatibility: JSON mode is available for all Mistral models through the API.
Tips for Optimizing JSON Responses:
- Be Specific: Clearly define the desired JSON structure in your prompt.
- Keep It Concise: Request short JSON objects to prevent overly lengthy outputs.
- Validate Output: Always check the returned JSON for correctness and structure.
- Iterative Refinement: Test and adjust your prompts to achieve consistent results.
Step-by-Step Guide with Code Example:
import os
from mistralai import Mistral
# Set up API key and model
api_key = os.environ["MISTRAL_API_KEY"]
model = "mistral-large-latest"
# Initialize Mistral client
client = Mistral(api_key=api_key)
# Define the message requesting JSON output
messages = [
{
"role": "user",
"content": "What is the best French meal? Return the name and ingredients in a short JSON object."
}
]
# Request chat completion with JSON format
chat_response = client.chat.complete(
model=model,
messages=messages,
response_format={"type": "json_object"}
)
# Print the JSON response
print(chat_response.choices[0].message.content)
Expected Output:
{
"name": "Coq au Vin",
"ingredients": ["chicken", "red wine", "bacon", "mushrooms", "onions", "garlic", "chicken broth", "thyme", "bay leaf", "flour", "butter", "olive oil", "salt", "pepper"]
}
Comparing JSON Outputs Across AI Platforms
When it comes to generating structured JSON outputs, each AI platform has its own approach. Here's a comparison of OpenAI, Groq, Gemini, and Mistral:
JSON Structure and Formatting:
- OpenAI: Uses a "Structured Outputs" feature with precise schema adherence.
- Groq: Offers a "JSON mode" that guarantees valid JSON responses.
- Gemini: Provides flexible JSON output with schema definition options.
- Mistral: Implements a straightforward JSON mode for structured outputs.
Pros and Cons:
OpenAI
| Groq
| Gemini
| Mistral
|
- Precise schema control
- Type safety and explicit refusals
- More complex setup
|
- Simple JSON mode activation
- Pretty-printed JSON support
- Limited to specific models (Mixtral > Gemma > Llama)
|
- Flexible schema definition (type hints or protobuf)
- Works with both Flash and Pro models
- May require more prompt engineering for consistency
|
- Straightforward implementation
- Available for all Mistral models
- Requires explicit JSON requests in prompts
|
Choosing the Right Platform:
- For Maximum Control: OpenAI's Structured Outputs offer the most precise schema adherence.
- For Simplicity: Mistral and Groq provide straightforward JSON modes that are easy to implement.
- For Flexibility: Gemini offers a good balance of control and ease of use, with options for both simple and complex schemas.
- For Performance: Consider Groq with the Mixtral model for optimal JSON generation speed.
When selecting a platform, consider your specific needs for schema complexity, ease of implementation, and the level of control required over the JSON output. Always test the outputs across different platforms to ensure they meet your application's requirements for structure, consistency, and accuracy.
Conclusion
As we've explored, AI-powered JSON generation is changing how developers interact with and leverage AI models. From OpenAI's structured outputs to Groq's JSON mode, Gemini's flexible schemas, and Mistral's straightforward approach, each platform offers unique capabilities for creating structured data.
Looking ahead, we can expect even more sophisticated JSON generation techniques, including:
- Enhanced schema validation and error handling
- More intuitive ways to define complex, nested structures
- Improved consistency and reliability in generated outputs
- Integration with data validation and transformation pipelines
The future of AI API responses lies in providing developers with greater control, flexibility, and efficiency in working with structured data. As these technologies evolve, they will enable more seamless integration of AI capabilities into a wide range of applications and services.
We encourage you to experiment with these JSON generation techniques across different AI platforms. By doing so, you'll not only enhance your applications but also contribute to the ongoing evolution of AI-powered data structuring. After obtaining your JSON, you can leverage it for data integrations or create documents by converting JSON to PDF or Word.