Be able to log the duration a snippet of code takes to execute, log it using your existing logger. Not like Benchmarking, which times the whole method, but if you just want to time a snippet of your code within your method. Plus, you can log multiple snippets within the same method.
Download SnippetDurationLogger.zip
Introduction
I'm sure like me, in numerous projects you've had to capture and investigate long running processes. Where you had to find the snippet of code that was causing the issue and then drilling down to see what lines where causing the latency.
You would have numerous logging statements salt and peppered throughout your code (often leaving them in by mistake!!!)
Background
This NuGet Package I created, has been developed for scenarios where a developer wishes to capture the execution time of a snippet of code. Not the execution time for a full method, but a snippet of code in the method and then log it.
Using the code
Add the following NuGet package to your project - SnippetDurationLogger
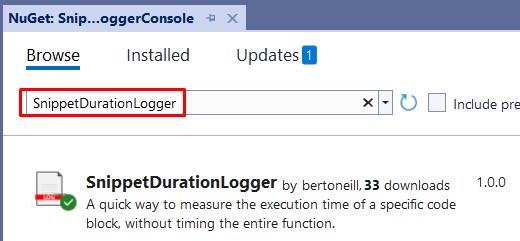
Add this namespace to your class:
using SnippetDuration.Classes;
Then, around the snippet of code you wish to get the execution time against, apply the Logger extension method LogExecutionTime
:
_logger.LogExecutionTime("Add simple comment...", () =>
{
});
In your comment, you can add string interpolation variables:
_logger.LogExecutionTime($"Fnx parameter - {myParam}", () =>
{
});
Then in your log file you will see the comment (and the interpolation values if included) along with the execution time:

Below is a complete example:
using Microsoft.Extensions.Logging;
using SnippetDuration.Classes;
namespace SnippetDurationLoggerConsole
{
internal class ExternalMethods
{
private readonly ILogger<ExternalMethods> _logger;
public ExternalMethods(ILogger<ExternalMethods> logger)
{
_logger = logger;
}
public void LongRunningprocess()
{
_logger.LogExecutionTime("Testing DB Call", () =>
{
System.Threading.Thread.Sleep(3500);
});
}
}
}