Introduction
If a database table contains duplicate records, then it is easy to select unique records by using DISTINCT function, but when a .NET DataTable contains duplicate records, then there is no built-in function to remove or select unique records from a DataTable
.
Objective
The main goal of this article is to create a function and remove and select unique records from a DataTable
in C#.NET and return a clean and duplicate free DataTable
.
Explanation
For removing duplicate records from DataTable
, I have created the below function which needs two parameters, DataTable
and Column Name. This function is searching for duplicate records in DataTable
, given column name from second parameter and adding the row (record) in an ArrayList
variable. Then, the record will be deleted from the datatable
by running a loop on founded duplicate records.
DataTable containing duplicate records:
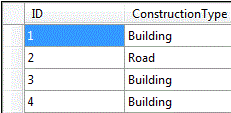
Function for Removing Duplicate Records from DataTable
public DataTable RemoveDuplicateRows(DataTable table, string DistinctColumn)
{
try
{
ArrayList UniqueRecords = new ArrayList();
ArrayList DuplicateRecords = new ArrayList();
foreach(DataRow dRow in table.Rows)
{
if (UniqueRecords.Contains(dRow[DistinctColumn]))
DuplicateRecords.Add(dRow);
else
UniqueRecords.Add(dRow[DistinctColumn]);
}
foreach (DataRow dRow in DuplicateRecords)
{
table.Rows.Remove(dRow);
}
return table;
}
catch (Exception ex)
{
return null;
}
}
Using the Function
It is very easy to use this function, all you need is to pass the datatable
and column name parameters to the function and the function returns the cleaned datatable
.
Example
DataTable DuplicateRecords = objDatabase.getTable("SQL Query");
DataTable UniqueRecords = RemoveDuplicateRows
(DuplicateRecords, "Column Name to check for duplicate records");
Clean and duplicate free DataTable
using the above function:
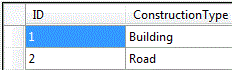
Remarks
The above function selects the first record and deletes others if a duplicate record is found in DataTable
.
Conclusion
By using this function, you will be able to clean your datatable from duplicate records.
Feel free to comment, suggest or give your feedback.