I’ve been using Resharper since I started coding in .NET/C#. It’s a tool with a lot of features which aids you in the average day development. In this blog post, I’m going to show you the features that I use the most.
Enter ALT+Enter
ALT+Enter is the hotkey that you have to start with. It’s a context sensitive beast that will do the most stuff for you.
Working with Classes
Let’s say that I create a new class:
public class UserRepository
{
}
In it, I need to use a DbContext
. So I create a new member field:
public class UserRepository
{
DbContext _dbContext;
}
However, when I type the semicolon, resharper inserts private
for me:
public class UserRepository
{
private DbContext _dbContext;
}
Resharper sees that it has not been initialized and gives a warning (hoover over the field):
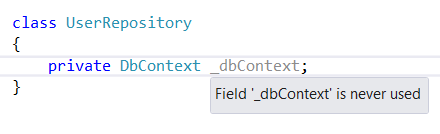
Pressing ALT+ENTER creates a constructor for me:

which results in:
class UserRepository
{
private DbContext _dbContext;
public UserRepository(DbContext dbContext)
{
_dbContext = dbContext;
}
}
Now I want to make sure that null
is not passed in, so I press ALT+ENTER on the argument name:

which adds an argument check:
class UserRepository
{
private DbContext _dbContext;
public UserRepository(DbContext dbContext)
{
if (dbContext == null) throw new ArgumentNullException("dbContext");
_dbContext = dbContext;
}
}
Let’s say that I create a new method in which I decide to change the return type:
class UserRepository
{
private DbContext _dbContext;
public UserRepository(DbContext dbContext)
{
if (dbContext == null) throw new ArgumentNullException("dbContext");
_dbContext = dbContext;
}
public User[] Find()
{
return _dbContext.Users.ToList();
}
}
But since that doesn’t match, the code will not compile. So I press ALT+ENTER on the return
statement:

Now I want to cache the users. So I need to reference the cache which exists in another project. I do that by writing the name and use ALT+ENTER:

That step will add a reference to the other project and import the correct namespace
. After that, I press ALT+ENTER again on the field name and add a constructor.
Renaming Files
Let’s say that I’ve renamed a class by just typing a new name (the file name is still the old one):

I then press ALT+Enter on the class name:

The file is now renamed.
Update namespaces
The file in the previous example should also be moved to a new folder. So I just drag it to the new one folder. However, the namespace
is not correct now, so Resharper gives a warning (by a visual indication = the blue underline):

which means that I can just press ALT+ENTER on it to rename the namespace
(which also updates all usages in the solution).

Summary
ALT+ENTER alone saves you a lot of typing and clicking. But most important: You get a much better flow when coding. No annoying stops for basic bootstrapping.
Unit Tests
Resharper gives you a new unit test browser and context actions for each test.
Let’s say that you create a simple test:

which you run:

and get a result:

The call stack is clickable which makes it easier to browse through the call stack to get an understanding of why the test failed. Anything written to the console (Console.WriteLine()
) also gets included in the output.
Code Navigation
The code completion in Resharper allows you to use abbreviations (the camel humps) to complete text:

If I type one more letter, I only get one match.

When you code against abstractions (interfaces), you’ll probably want to go to the implementation. ReSharper adds a new context menu item which allows you to do so:

If there is only one implementation, it’s opened directly. If there are more than one, you get a list:

CTRL+T opens up a dialog which you can use to navigate to any type. You can either type abbreviations or partial names:

CTRL+SHIFT+T is similar, but is used for files:

Finally, we have “Find usages” which analyses the code and finds where a type/method is used:


Code Cleanup
This well structured file can be only better:

I press ALT+E, C to initiate the cleanup dialog:

The “usevar
” is my own custom rule where I change all possible usages to use var
over explicit declarations.
Result:

Notice that the property got moved up above the method and that unused “using
” directives where removed. You can also automatically sort methods and properties.
Finally, Resharper shows a warning about the property (since it’s not initialized). ALT+Enter and choose to initialize it using a constructor solves that:

Summary
Those are the features that I use the most. What are your favorite features?
The post How Resharper rocks my average work day appeared first on jgauffin's coding den.