Introduction
This is a jQuery based confirm plugin that will enhance built in confirm
-function in JavaScript.
Background
I hate built in confirm
and alert
functions in JavaScript, so I ended up with my own confirm
and alert
function.
The code was not ready for the public, so I decide to convert it to a jQuery plug-in.
Here, I am presenting to you eziConfirm
jQuery plug-in.
Using the Code
Files
The attached zip file contains all you need.
All you need is to include jQuery, eziconfirm.js and eziconfirm.css.
<link rel="stylesheet" type="text/css" href="eziConfirm.css" />
<script type="text/javascript" src="jquery-1.9.0.min.js"></script>
<script type="text/javascript" src="eziConfirm.js"></script>
Simple Confirm Window
The easiest way to use eziConfirm
is by calling the jqConfirm
plugin with only FireFunction
parameter:
$(document.body).eziConfirm(
{
FireFunction: function () {alert('You Click Yes')}
}
)
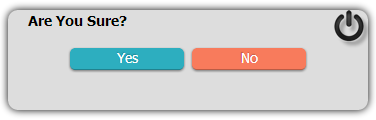
Custom Button(s)
eziConfirm
shows two buttons (Yes and No) as default, but you can add your custom button(s).
$(document.body).eziConfirm(
{
Button:
[
{ Title: "Delete", FireFunction: function (){ alert('You Click Delete') } },
{ Title: "Cancle", ClassName: 'red' }
]
}
);

Changing Title, Description, Width and Height
$(document.body).eziConfirm(
{
Title: 'Are you sure you want to delete this file?',
Desc: 'File01.pdf<br/>Type:PDF<br/>Size:40KB',
Width:320,
Height:200,
Button:
[
{ Title: "Delete", FireFunction: function (){ alert('You Click Delete') } },
{ Title: "Cancle", ClassName: 'red' }
]
}
);

Hide Close Button
$(document.body).eziConfirm({
CloseButton: false,
FireFunction: function () { alert('You Click Yes') }
});

Use As Alert Window
$(document.body).eziConfirm({
Title: 'Alert Window',
Desc: 'Description Description Description Description
Description Description Description Description',
Button: [{ Title: 'OK', ClassName: 'red'}]
});

Use As Message Window
$(document.body).eziConfirm({
Title: 'Message',
Desc: 'Message Message Message Message Message Message Message Message',
Button: []
});

Buttons Position
eziConfirm
supports 4 different buttons positioning:
RenderMode.horizontal
: Render buttons horizontally RenderMode.vertical
: Render buttons vertically RenderMode.auto
: Render buttons in n*m table RenderMode.manual
: Render buttons in given number of columns
...
RenderMode: RenderMode.horizontal,
...

...
RenderMode: RenderMode.vertical,
...

...
RenderMode: RenderMode.auto,
...

...
RenderMode: RenderMode.manual,
Cols:3,
...

Right To Left Support
$(document.body).eziConfirm({
Direction: 'rtl',
FireFunction: function () { alert('You Click Yes') }
});

Advance Sample: Calculator
The below sample shows many eziConfirm
options and abilities.
$(document.body).eziConfirm(
{
Title: 'Calculator',
Desc: '<div id=\'result\'
style=\'font-weight:bold;font-size:16px;height:22px;background-color:#ffffff\'></div>',
Height: 170,
Width: 240,
RenderMode: RenderMode.manual,
Cols: 4,
ButtonDefault: { Width: 33, AutoClose: false },
Button:
[
{ Title: "1", FireFunction: function () { CalcKeyPress('1') } },
{ Title: "2", FireFunction: function () { CalcKeyPress('2') } },
{ Title: "3", FireFunction: function () { CalcKeyPress('3') } },
{ Title: "AC", ClassName: 'red', FireFunction: function () { CalcKeyPress('ac') } },
{ Title: "4", FireFunction: function () { CalcKeyPress('4') } },
{ Title: "5", FireFunction: function () { CalcKeyPress('5') } },
{ Title: "6", FireFunction: function () { CalcKeyPress('6') } },
{ Title: "+", ClassName: 'red', FireFunction: function () { CalcKeyPress('+') } },
{ Title: "7", FireFunction: function () { CalcKeyPress('7') } },
{ Title: "8", FireFunction: function () { CalcKeyPress('8') } },
{ Title: "9", FireFunction: function () { CalcKeyPress('9') } },
{ Title: "-", ClassName: 'red', FireFunction: function () { CalcKeyPress('-') } },
{ Title: "0", FireFunction: function () { CalcKeyPress('0') } },
{ Title: "*", ClassName: 'red', FireFunction: function () { CalcKeyPress('*') } },
{ Title: "/", ClassName: 'red', FireFunction: function () { CalcKeyPress('/') } },
{ Title: "=", ClassName: 'tra', FireFunction: function () { CalcKeyPress('=') } }
]
}
);
function CalcKeyPress(key) {
switch (key) {
case 'ac':
$('#result').html('');
break;
case '=':
$('#result').html(eval($('#result').html()));
break;
default:
$('#result').html($('#result').html() + key);
break;
}
}

Options
This section describes the options available to eziConfirm
. These are set with the argument to the $('#selector').eziConfirm(options)
function.
Options are described with the following convention:
attribute: default,
var defaultParam = {
Title: "Are You Sure?",
ClassName : "",
Desc: "",
Width: 320,
Height: 60,
RenderMode: RenderMode.horizontal,
Cols: 1,
Button: [ Button1, Button2, ...] ,
ButtonDefault : {
Width: 100,
Height: 18,
ClassName: '',
AutoClose:true,
FireGlobalFunction: false,
FireFunction: function () { }
},
FireFunction: function () {} ,
CloseButton: true,
Direction: 'ltr'
};
var Button = {
Title: "Yes",
FireGlobalFunction: true ,
Width: 100,
Height: 18,
ClassName: '',
AutoClose:true,
FireFunction: function () { }},
}
Code
The attached zip file contains all you need.
What's Next?
- Template base buttons position
- Auto Close Timer
History
- 2013/02/16: Version 0.2
- Right to Left Support
- Button Default Options
- Option to Hide/Show Close Button
- New Samples (Alert, Message, ...)
- 2013/02/12: First release (version 0.1)