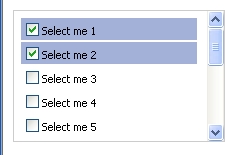
Introduction
In this article, I will show you a different way of doing a multi-select in an ASP.nET page. I will keep this article short and sweet so you can just use the code in your applications.
Using the code
We are going to put our CheckBoxList
ASP.NET control inside an HTML div
object. I have also added code to support changing the background color of the selected row.
<%@ Page Language="C#" AutoEventWireup="true"
CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<style type="text/css">
.scroll_checkboxes
{
height: 120px;
width: 200px;
padding: 5px;
overflow: auto;
border: 1px solid #ccc;
}
.FormText
{
FONT-SIZE: 11px;
FONT-FAMILY: tahoma,sans-serif
}
</style>
<script language="javascript">
var color = 'White';
function changeColor(obj)
{
var rowObject = getParentRow(obj);
var parentTable =
document.getElementById("<%=chkList.ClientID%>");
if(color == '')
{
color = getRowColor();
}
if(obj.checked)
{
rowObject.style.backgroundColor = '#A3B1D8';
}
else
{
rowObject.style.backgroundColor = color;
color = 'White';
}
function getRowColor()
{
if(rowObject.style.backgroundColor == 'White')
return parentTable.style.backgroundColor;
else return rowObject.style.backgroundColor;
}
}
function getParentRow(obj)
{
do
{
obj = obj.parentElement;
}
while(obj.tagName != "TR")
return obj;
}
function TurnCheckBoixGridView(id)
{
var frm = document.forms[0];
for (i=0;i<frm.elements.length;i++)
{
if (frm.elements[i].type == "checkbox" &&
frm.elements[i].id.indexOf("<%= chkList.ClientID %>") == 0)
{
frm.elements[i].checked =
document.getElementById(id).checked;
}
}
}
function SelectAll(id)
{
var parentTable = document.getElementById("<%=chkList.ClientID%>");
var color
if (document.getElementById(id).checked)
{
color = '#A3B1D8'
}
else
{
color = 'White'
}
for (i=0;i<parentTable.rows.length;i++)
{
parentTable.rows[i].style.backgroundColor = color;
}
TurnCheckBoixGridView(id);
}
</script>
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<div class="scroll_checkboxes">
<asp:CheckBoxList Width="180px" ID="chkList"
runat="server" CssClass="FormText"
RepeatDirection="Vertical" RepeatColumns="1"
BorderWidth="0" Datafield="description"
DataValueField="value">
<asp:ListItem id="text1" Value="Select me 1"
onclick="changeColor(this);"></asp:ListItem>
<asp:ListItem id="text2" Value="Select me 2"
onclick="changeColor(this);"></asp:ListItem>
<asp:ListItem id="text3" Value="Select me 3"
onclick="changeColor(this);"></asp:ListItem>
<asp:ListItem id="text4" Value="Select me 4"
onclick="changeColor(this);"></asp:ListItem>
<asp:ListItem id="text5" Value="Select me 5"
onclick="changeColor(this);"></asp:ListItem>
<asp:ListItem id="text6" Value="Select me 6"
onclick="changeColor(this);"></asp:ListItem>
<asp:ListItem id="text7" Value="Select me 7"
onclick="changeColor(this);"></asp:ListItem>
<asp:ListItem id="text8" Value="Select me 8"
onclick="changeColor(this);"></asp:ListItem>
<asp:ListItem id="text9" Value="Select me 9"
onclick="changeColor(this);"></asp:ListItem>
<asp:ListItem id="text10" Value="Select me 10"
onclick="changeColor(this);"></asp:ListItem>
<asp:ListItem id="text11" Value="Select me 11"
onclick="changeColor(this);"></asp:ListItem>
<asp:ListItem id="text12" Value="Select me 12"
onclick="changeColor(this);"></asp:ListItem>
<asp:ListItem id="text13" Value="Select me 13"
onclick="changeColor(this);"></asp:ListItem>
</asp:CheckBoxList>
</div>
</div>
</form>
</body>
</html>
If you would need to load this list from a database, then you can easily bind the CheckBoxList
to a DataSource
object.
To add the JavaScript call to change the color, just use text1.Attributes.Add("onclick", "changeColor(this)");
in the code-behind for each ListItem
control (you would need to use a loop).
Highlight the row in a GridView using the same Javascript code
You can use the same JavaScript for highlighting a row in a GridView
; for example, let's say, you have a GridView
with an ItemTemplate
that has a CheckBox
.
Adding onclick="changeColor(this)"
to the checkbox will do the trick.
<ItemTemplate >
<asp:CheckBox ID="chkSelect" runat="server"
Width = "24px" Visible = "true"
onclick="changeColor(this)" EnableViewState="True" />
<asp:Label ID="HiddenShipmentID" runat="server"
visible = "false"
Text = '<%# Eval("shipment_id") %>'></asp:Label>
</ItemTemplate>.....
All you have to do is change the JavaScript to use the name of the GridView
:
var parentTable = document.getElementById("<%=chkList.ClientID%>");
Change that to:
var parentTable = document.getElementById("<%=GridViewName.ClientID%>");
And that's all.
I hope you enjoyed this little trick. If you liked this idea, feel free to vote.