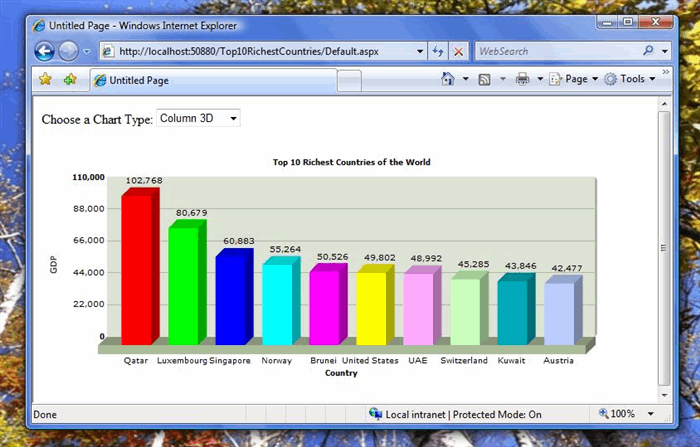

Introduction
FusionCharts Free is an open source free Flash charting component which can be used to display high quality animated charts. FusionCharts can be used with many scripting languages like PHP, ASP.NET, JSP, ColdFusion, JavaScript, etc.
Following are the main advantages of FusionCharts:
- No installation required
FusionCharts works without installation. You just need to copy the swf files on the server. - Ease of use
FusionCharts are easy to use. There is no need to learn flash or any other authoring tool to be able to use FusionCharts. You just have to use XML to define the data. - FusionCharts run on many platforms
Since FusionCharts are based on XML, they can run on a variety of platforms. - FusionCharts are efficient
FusionCharts are rendered on the client-side using Flash. As a result there is no network congestion and load on the server. - Free
FusionCharts Free are completely free.
Background
To demonstrate the working of FusionCharts, I have created a Web Application in ASP.NET with C#. The application shows the top 10 richest countries of the world on the basis of GDP Per Capita. The application is created using Visual Web Developer 2005 Express Edition.
The data is for the period 2011-2012 and taken from the International Monetary Fund, which uses the GDP per capita of each country and is taken from the following site:
Using the code
The user interface of the application consists of a DropDownList control which allows the chart type to be selected and a Literal control which displays the selected chart. The AutoPostBack
property of the DropDownList
control is set to True to automatically cause a PostBack when a different chart type is selected.
The FusionCharts package contains a file called FusionCharts.dll. A reference to this file is added in the solution as follows:

I added a folder called Data in the solution and added a new XML file called Data.xml in the folder with the following contents:
="1.0"="utf-8"
<graph caption='Top 10 Richest Countries of the World' xAxisName='Country'
yAxisName='GDP' showNames='1' decimalPrecision='0' formatNumberScale='0'>
<set name='Qatar' value='102768' color='#FF0000' />
<set name='Luxembourg' value='80679' color='#00FF00' />
<set name='Singapore' value='60883' color='#0000FF' />
<set name='Norway' value='55264' color='#00FFFF' />
<set name='Brunei' value='50526' color='#FF00FF' />
<set name='United States' value='49802' color='#FFFF00' />
<set name='UAE' value='48992' color='#FFAAFF' />
<set name='Switzerland' value='45285' color='#CCFFBB' />
<set name='Kuwait' value='43846' color='#00AABB' />
<set name='Austria' value='42477' color='#BBCCFF' />
</graph>
The I added another folder called FusionCharts and added all the chart swf files from the Charts folder of the FusionCharts package.
Following is the figure of the solution explorer after adding the above mentioned contents:

Following is the code-behind in the Default.aspx.cs file:
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using InfoSoftGlobal;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
DropDownList1_SelectedIndexChanged(sender, e);
}
void ShowChart(string type)
{
Literal1.Text = FusionCharts.RenderChartHTML("FusionCharts/" +
type + ".swf", "Data/Data.xml", "", "countries", "700", "300", false);
}
protected void DropDownList1_SelectedIndexChanged(object sender, EventArgs e)
{
ShowChart(DropDownList1.SelectedValue);
}
}
In the above code, I have imported the InfoSoftGlobal
namespace from the FusionCharts.dll file. This namespace contains the FusionCharts
class. The ShowChart()
user-defined function renders the chart and displays the output on the Literal control using the RenderChartHTML
function of the FusionCharts
class. The SelectedIndexChanged
event of the DropDownList
control passes the chart type selected by the user as a parameter to the ShowChart
function. The Page_Load
event displays the default chart by calling the SelectedIndexChanged
event of the DropDownList
control.
Following are the parameters of the RenderChartHTML
function:
- SWF filename with the path
- XML data filename with the path
- XML data as a string (This parameter can be "" if you are using xml data file)
- ID for the chart
- Chart Width
- Chart Height
- Debug Mode
Points of Interest
FusionCharts provide a quick, easy and efficient method to display good looking charts using any scripting language.