Shell scripts are awesome. One can do whatever with a shall script. With whatever, I mean whatever deadly or creative.
However in this article, I will try to show how a simple script can be used to kill an application. Whatever application you start from a command prompt. Now the question is what is the difference between an application and a process. Well, a process is a simple entity that runs in the memory with its own stack memory and resources. Advanced processes can have multiple threads running simultaneously. But an application is a combination of processes running together and maintaining inter process communication to handle many tasks at the same time. Typically, an application is a large project under the hood of some group of processes.
Now let's invoke a process for Gnome-Commander with the command:
nohup gnome-commander &
This nohup
is special command to run a process from terminal and isolate its standard I/O from the terminal. The &
after the command will run the command in background so you can use the terminal for other tasks.
Now let's see the process in the process list. Hit the command:
ps
or:
ps -Al
With the -Al
, you will get all the currently running processes in your privilege. But without the -Al
argument, you will get the process-list invoked from current terminal.
Now is the time to kill the processes. To kill a process, simply invoke kill -9 [process_id]
and this [process_id]
you will get from the process-list returned by ps
.
The kill
command basically sends various type of signal to a process. You can see all type of signals with kill -l
and will find SIGKILL
as number 9
. So when you invoke kill [process_id]
, the kill
command actually sends a SIGKILL
signal to that process of id [process_id
]. This is a signal to terminate the process and approximately all the processes handle this signal by terminating itself.
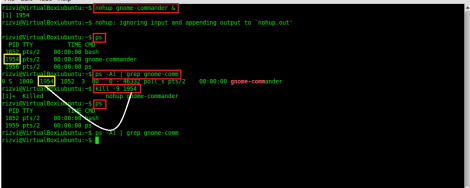
Up to now, this is very simple. Now let's write a script out of this knowledge to kill an application. To find out how an application runs, let's run ‘Chromium-Browser’ application with the command:
nohup chromium-browser &
Now watch how many processes support this application with:
ps -Al | grep chromium
You will find a bundle of processes running behind the application.
Now let's write a script that will close an application (i.e., Chromium-Browser). Create a file and name it ‘killproc
‘ and write #!/bin/bash
at the very first position of the file. Note that if the shell finds ‘#!
’ at the starting of the file, then it will treat this file as a script and will run it in kernel.
So our first step is to take an application name or a name fragment as the command line argument.
if [ $# -lt 1 ]; then
echo "Process name missing !!"
exit 1
...
Here, the $#
indicates the number of command line arguments and $1
or $2
holds the first or second arguments and so on.
The next step is to get the process list with ps
command. Now to filter out our desired process, we need to pipeline the output of ps
to another command awk
which is actually a nice tool to filter in rows and columns.
if [ $# -lt 1 ]; then
echo "Process name missing !!"
exit 1
else
list=($(ps | awk '{if((index($4,"'$1'")>0) && ($1!="PID")) {print $1}}'))
echo "Process count: "${
max=${
if [ ${#list[*]} -eq 0 ]
then
echo "No process found of this name."
exit 0
fi
...
Our filtering command is ps | awk '{if((index($4,"'$1'")>0) && ($1!="PID")) {print $1}}'
. With this command, we are actually getting a list of process ids associated with our application. Then, fill an array with this list of processes by list=($(...))
where … is some command that produces a list.
Next step is to loop through this list of process ids’ and kill them one by one.
if [ $# -lt 1 ]; then
echo "Process name missing !!"
exit 1
else
list=($(ps | awk '{if((index($4,"'$1'")>0)
&& ($1!="PID")) {print $1}}'))
echo "Process count: "${
max=${
if [ ${#list[*]} -eq 0 ]
then
echo "No process found of this name."
exit 0
fi
for ((i=0; i<$max; ++i ));
do
echo "Killing process..."${list[$i]}
kill -9 ${list[$i]}
done
if [ $? -eq 0 ]; then
echo "All process killed sucessfully."
else
echo "Process killed ..."
fi
fi
With a for
loop, we traverse through the process ids and kill them one by one with kill -9 ${list[$i]}
. Finally, we give a friendly message.
Now to kill a ‘Chromium-Browser’ application, we simply invoke:
killproc chromium
This will kill all the associated processes with Chromium-Browser.

Try it yourself and have fun!