Introduction
In this article I've discussed about WCF REST services and how to consume them in a normal
HTML page. We will see what is required from
a service developer's perspective to create a REST enabled WCF service. We see how we can use and
consume RESTful WCF services.
Background
Overview of REST
REST stands for Representational State Transfer. This is a protocol for exchanging data over a
distributed environment. The main idea behind REST is that we should treat our distributed services as
a resource and we should be able to use simple HTTP protocols to perform various operations on that
resource.
Now the basic CRUD operations are mapped to the HTTP protocols in the following manner:
-
GET: This maps to the
R(Retrieve)
part of the CRUD operation. This will be used to retrieve the required
data (representation of data) from the remote resource.
-
POST: This maps to the
U(Update)
part of the CRUD operation. This protocol will update the current representation
of the data on the remote server.
-
PUT: This maps to the
C(Create)
part of the CRUD operation. This will create a new entry for the current data that
is being sent to the server.
-
DELETE: This maps to the
D(Delete)
part of the CRUD operation. This will delete the specified data from the remote server.
Walk through application
When you create a WCF service application project in
Visual Studio you will get the IService1.cs and Service1.svc files along with
a few other project related stuff. First we will discuss a few definitions which will be used in the article.
A Service Contract can be defined using the [ServiceContract]
and
[OperationContract]
attribute. The [ServiceContract]
attribute is similar to the
[WebServcie]
attribute
in the WebService and [OpeartionContract]
is similar to the [WebMethod]
in
a WebService.
Now we will see how to create two web service methods:
- Method that returns the employee class instance as XML output.
- Method that returns the employee class instance as JSON output.
In the code, I've created two entities:
EmployeeXML
class
using System.Runtime.Serialization;
namespace WcfServiceXmlAndJsonDemo
{
[DataContract]
public class EmployeeXML
{
#region Properties
[DataMember]
public string Name { get; set; }
[DataMember]
public int Id { get; set; }
[DataMember]
public double Salary { get; set; }
#endregion
}
}
EmployeeJSON
class:
namespace WcfServiceXmlAndJsonDemo
{
[DataContract]
public class EmployeeJSON
{
#region Properties
[DataMember]
public string Name { get; set; }
[DataMember]
public int Id { get; set; }
[DataMember]
public double Salary { get; set; }
#endregion
}
}
The first method's return type is an instance of the EmployeeXML
class and the other for the second method. Now I will show you how to expose our service methods to the clients.
Declare the two methods in the IService1
interface as follows:
[ServiceContract]
public interface IService1
{
#region OperationContracts
[OperationContract]
[WebInvoke(Method="GET",UriTemplate="GetXml",
ResponseFormat=WebMessageFormat.Xml,RequestFormat=WebMessageFormat.Xml,
BodyStyle=WebMessageBodyStyle.Bare)]
EmployeeXML GetEmployeeXML();
[OperationContract]
[WebInvoke(Method = "GET", UriTemplate = "GetJson",
ResponseFormat = WebMessageFormat.Json,
RequestFormat = WebMessageFormat.Json,
BodyStyle = WebMessageBodyStyle.Wrapped)]
EmployeeJSON GetEmployeeJSON();
#endregion
}
Method="GET"
specifies that it is the HTTP GET verb which performs the retrieval operation. UriTemplate="GetXml"
is the path through which the method is accessed (http://localhost/Service1.svc/GetXml).
ResponseFormat
specifies the response format of the method and
RequestFormat
is the way you should send data if any parameters are present for the method to request any data.
Now implement the methods in the Service1.svc file as follows:
public class Service1 : IService1
{
#region ImplementedMethods
public EmployeeXML GetEmployeeXML()
{
EmployeeXML xml = new EmployeeXML() {Name="Sudheer",Id=100,Salary=4000.00 };
return xml; ;
}
public EmployeeJSON GetEmployeeJSON()
{
EmployeeJSON json = new EmployeeJSON() {Name="Sumanth",Id=101,Salary=5000.00 };
return json;
}
#endregion
}
The next important thing is the Web.config file which is the key part of the service. In
web.config you should specify the service metadata, that is, the details of binding
and the endpoint through which the clients can access your service. The important things to consider in
web.config file are:
- Service behavior.
- End point behavior.
<system.servicemodel>
<behaviors>
<servicebehaviors>
<behavior>
<servicemetadata httpsgetenabled="true" httpgetenabled="true">
<servicedebug includeexceptiondetailinfaults="false">
</servicedebug> </servicemetadata></behavior>
</servicebehaviors>
<endpointbehaviors>
<behavior name="web">
<webhttp>
</webhttp></behavior>
</endpointbehaviors>
</behaviors>
<protocolmapping>
<add scheme="https" binding="basicHttpsBinding">
</add></protocolmapping>
<servicehostingenvironment multiplesitebindingsenabled="true" aspnetcompatibilityenabled="true">
<services>
<service name="WcfServiceXmlAndJsonDemo.Service1">
<endpoint binding="webHttpBinding"
contract="WcfServiceXmlAndJsonDemo.IService1" behaviorconfiguration="web">
</endpoint> </service>
</services>
</servicehostingenvironment>
</system.servicemodel>
Testing the Service
Now right click on the Service1.svc file and select the View in browser option as follows:
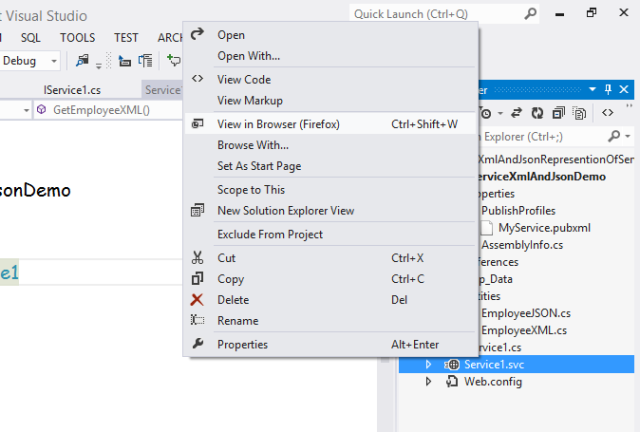
Now your browser looks something like this:

Now add the uritemplate
of the method to see the output. Ex: localhost:1249/Service1.svc/GetXml gives you the following output:

The same way you can also see the JSON output by adding the uritemplate
specific to the JSON method (GetJson
). Following is the JSON
output:

Client application to consume the WCF service
Now go ahead and create an empty web application and add an HTML page with the following code. Note: Don't forget to add the jQuery library to the application
as we are going to consume the WCF service using a jQuery AJAX call. The HTML code for the page is as follows (part of
the code, for full code please go through the code I've attached):
<script>
$(document).ready(function () {
});
var GetJson = function () {
$.ajax({
type: "GET",
url: "http://localhost:4545/Service/Service1.svc/GetJson",
contentType: "application/json;charset=utf-8",
dataType: "json",
success: function (data) {
var result = data.GetEmployeeJSONResult;
var id = result.Id;
var name = result.Name;
var salary = result.Salary;
$('#jsonData').html('');
$('#jsonData').append('<table border="1"><tbody><tr><th>" +
"Employee Id</th><th>Name</th><th>Salary</th>" +
"</tr><tr><td>' + id + '</td><td>' + name +
'</td><td>' + salary + '</td></tr></tbody></table>');
},
error: function (xhr) {
alert(xhr.responseText);
}
});
}
var GetXml = function () {
$.ajax({
type: "GET",
url: "http://localhost:4545/Service/Service1.svc/GetXml",
dataType: "xml",
success: function (xml) {
$(xml).find('EmployeeXML').each(function () {
var id = $(this).find('Id').text();
var name = $(this).find('Name').text();
var salary = $(this).find('Salary').text();
$('#xmlData').html('');
$('#xmlData').append('<table border="1"><tbody><tr><th>Employee" +
" Id</th><th>Name</th><th>Salary</th></tr><tr><td>' +
id + '</td><td>' + name + '</td><td>' + salary +
'</td></tr></tbody></table>');
});
},
error: function (xhr) {
alert(xhr.responseText);
}
});
}
</script>
The URL I've provided above is already published and hosted in IIS. It may vary from yours. So please look through the below steps for how to host the application under IIS.
Now we are ready with the code side. The next big part is hosting your service and client application in IIS in
the same port. Why I've have specified the same port is because of
the WCF service account's cross domain issues i.e., the client application should run under
the same port that the service runs.
Hosting the application in IIS
Step 1: Publish your application by right clicking the project and selecting the
Publish option as follows:
Publishing the Service





Publishing the Client




Note: Publish both the client and service application in the same parent folder.

Step 2: Add a new website under IIS and map that physically to the application parent folder as follows:

Be cautious while adding the dite name because while typing the name it will also
be reflected in the application pool as follows:






Now you can run your client application and you will see the following output:
Caution: You will get the following error:

Now go back to IIS and select Client and choose the following:






Hope you will find this helpful.