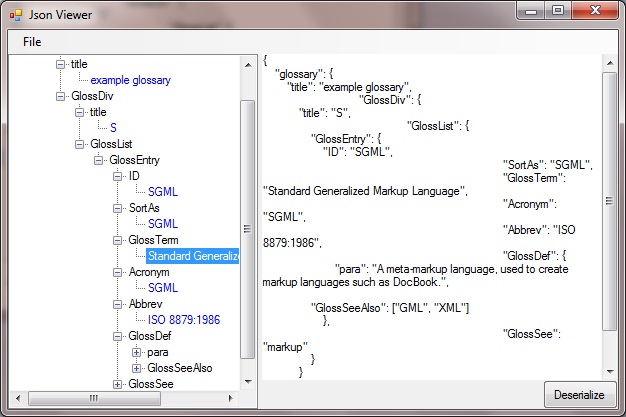
Introduction
As an web developer, I'm almost always working with JSON data. Most of the time, I can look at the data and get a good understanding of what's going on, and if needed, I just run it through a JSON decode script usually in PHP. But recently, I was given a large JSON file which was initially an Ajax response from a website. Normally, I would just write a simple script to make the data more readable but after working with the JavaScriptSerializer
class in a recent .NET web application, I wondered how difficult it would be to develop a Windows application that could decode JSON data and present it to me in a more meaningful way.
It's been a while since I worked on a Windows Forms application and immediately I ran into a problem. For some reason, I was not able to add a reference to System.Web.Extensions
. The Add Reference dialog box didn't list the reference. After some time of head banging and frustration, I realized it was to do with the target framework. My application was targeting the .NET Framework 4 Client Profile. I switched it to use .NET Framework 4 and checked the reference list again. From this moment on, the head banging stopped.
Now that I was able to add the reference, I could use the System.Web.Script.Serialization
namespace. Within this namespace is a class called JavaScriptSerializer
. It has a Deserialize
method, which can deserialize the JSON data to a Type. I used a Dictionary
type since JSON can be considered to be a key/value pair where value can also be an array. Listing 1.1 below shows an excerpt of the deserializing process from the sample application.
Listing 1.1
.......
JavaScriptSerializer js = new JavaScriptSerializer();
try
{
Dictionary<string,> dic = js.Deserialize<dictionary<string,>>(txtInput.Text);
TreeNode rootNode = new TreeNode("Root");
jsonExplorer.Nodes.Add(rootNode);
BuildTree(dic, rootNode);
}
catch (ArgumentException argE)
{
MessageBox.Show("JSON data is not valid");
}
Once I had deserialized the data, I needed a way to show it. For this, I chose to use a TreeView
control where a TreeNode
could represent both a key and a value. Notice in the code above, I have a method BuildTree()
, which accepts a Dictionary
object and a TreeNode
. This method recursively loops through each item and checks if the value is of Type Dictionary
and if it is, it passes the new Dictionary
object to itself (BuildTree
) with a parent TreeNode
.
If the value is not of Type Dictionary
, then I check if it is of Type ArrayList
and if it is, then I loop through the items and add them as the final TreeNode
. Finally, if the value if not of either Dictionary
or ArrayList
Types, then I assume it is a string
and create a final TreeNode
. I gave a blue text color to each final TreeNode
value to indicate it was not a parent TreeNode
.
Listing 1.2 below shows the complete BuildTree
method.
Listing 1.2
public void BuildTree(Dictionary<string,> dictionary, TreeNode node)
{
foreach (KeyValuePair<string,> item in dictionary)
{
TreeNode parentNode = new TreeNode(item.Key);
node.Nodes.Add(parentNode);
try
{
dictionary = (Dictionary<string,>)item.Value;
BuildTree(dictionary, parentNode);
}
catch (InvalidCastException dicE) {
try
{
ArrayList list = (ArrayList)item.Value;
foreach (string value in list)
{
TreeNode finalNode = new TreeNode(value);
finalNode.ForeColor = Color.Blue;
parentNode.Nodes.Add(finalNode);
}
}
catch (InvalidCastException ex)
{
TreeNode finalNode = new TreeNode(item.Value.ToString());
finalNode.ForeColor = Color.Blue;
parentNode.Nodes.Add(finalNode);
}
}
}
}
The rest of the application is made up of reading a file with the help of the OpenFileDialog
class and the System.IO namespace
.
History
- 1st August, 2013: Initial version