Introduction
- The ?? operator is called the null-coalescing operator
- It's used to define a default value for nullable value types or reference types
- It returns the left-hand operand if the operand is not null
- Otherwise it returns the right operand
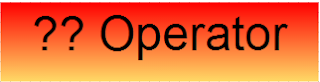
Compile-Time Errors and Exceptions
- A nullable type can contain a value, or it can be undefined
- The ?? operator defines the default value to be returned when a nullable type is assigned to a non-nullable type
- If you try to assign a nullable value type to a non-nullable value type without using the ?? operator, you will generate a compile-time error
It's like this
int? x = null;int z = 0;
z = x;

But if you use ?? operator. You won't have any problem.
z = x ?? 1;
- If you use a cast, and the nullable value type is currently undefined, an
InvalidOperationException
exception will be thrown
It's like this
int? x = null;int z = 0;
z = (int)x;

But If you use ?? operator, you won't have any problem.
z = x ?? 1;
- The result of a ?? operator is not considered to be a constant even if both its arguments are constants
Example
class Program
{
static void Main(string[] args)
{
int? x = null;
int y = x ?? -1;
int i = GetNullableInt() ?? default(int);
string s = GetStringValue();
var z = s ?? "Unspecified";
}
static int? GetNullableInt()
{
return null;
}
static string GetStringValue()
{
return null;
}
}
Output
Example 01

Example 02

Example 03
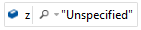
Usage of ?? Operator
- The chaining is a big plus for the ?? operator. It removes a bunch of redundant IFs
e.g.
string finalVal = parm1 ?? localDefault ?? globalDefault;
- You can easily convert nullable value types into non-nullable types
e.g.
int? test = null;
var result = test ?? 0;
- You can use null-coalescing operator in lazy instantiated
private
variables
e.g.
private IList<Car> _car;
public ILis<Car> CarList
{
get { return _car ?? (_car = new List<Car>()); }
}
References
Conclusion
- It can greatly enhance your code, making it concise and pleasant to read.
- Enjoy this ?? operator and let me know if you have any issues.
I hope this helps you. Comments and feedback are greatly appreciated.
You Might Also Like