Introduction
This is the third post
of JQuery With SharePoint 2010 series. Please read the first one, how to Integrate/use
JQuery with SP 2010. There are multiple ways to create Organization Chart
in SharePoint including the out of box one. Here I would like to discuss and
share the code, using which you can create simple organization chart web part.
I would like
accomplish following things in the article.
Prerequisites:
Download JQuery from Here Download JQuery plugin jOrgChart from here.
*** Please download the .zip file get full code
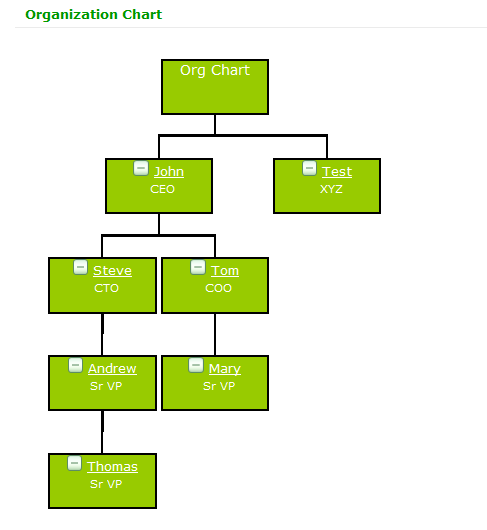
Org Chart Web Part
Create a Visual Web
Part with the following structures.
OrgChartWebPartUserControl.ascx: Fetchs employees from the organization list and formats the content as required by the JQuery Plugin.
Layouts: Contains all the JQuery, JOrgChart Plugin and Images files.
OrgChart List Feature will create a list “OrgChart”, which is pre-populated with some dummy data. Visual web part is going to pick up the employee list from here.

OrgChart List Feature
This
feature will create a list which will store the employee list of an organization.
List has following columns.
Employee
Designation
Manager
You
can add email, phone number columns based on your requirements. For now, I
would like to keep it simple.
Following
code is added in FeatureActivated method.
public override void FeatureActivated(SPFeatureReceiverProperties properties)
{
var web = properties.Feature.Parent as SPWeb;
if (web == null)
return;
var listId = web.Lists.Add("OrgChart", "Organization Structure", SPListTemplateType.GenericList);
var list = web.Lists[listId];
list.OnQuickLaunch = true;
list.Update();
var title = list.Fields["Title"];
title.Hidden = true;
title.Update();
var empName = list.Fields.Add("Employee", SPFieldType.Text, true);
var designation = list.Fields.Add("Designation", SPFieldType.Text, true);
var manager = list.Fields.AddLookup("Manager", list.ID, false);
SPFieldLookup managerField = (SPFieldLookup)list.Fields[manager];
managerField.LookupField = list.Fields["Employee"].InternalName;
managerField.Update();
var view = list.DefaultView;
if (view.ViewFields.Exists("LinkTitle"))
{
view.ViewFields.Delete("LinkTitle");
}
view.ViewFields.Add(empName);
view.ViewFields.Add(designation);
view.ViewFields.Add(manager);
view.Update();
var item1 = list.Items.Add();
item1[empName] = "John";
item1[designation] = "CEO";
item1.Update();
var item2 = list.Items.Add();
item2[empName] = "Steve";
item2[designation] = "CTO";
item2[manager] = new SPFieldLookupValue(item1.ID, item1[empName].ToString());
item2.Update();
var item3 = list.Items.Add();
item3[empName] = "Tom";
item3[designation] = "COO";
item3[manager] = new SPFieldLookupValue(item1.ID, item1[empName].ToString());
item3.Update();
var item4 = list.Items.Add();
item4[empName] = "Andrew";
item4[designation] = "Sr VP";
item4[manager] = new SPFieldLookupValue(item2.ID, item2[empName].ToString());
item4.Update();
var item5 = list.Items.Add();
item5[empName] = "Mary";
item5[designation] = "Sr VP";
item5[manager] = new SPFieldLookupValue(item3.ID, item3[empName].ToString());
item5.Update();
var item6 = list.Items.Add();
item6[empName] = "Thomas";
item6[designation] = "Sr VP";
item6[manager] = new SPFieldLookupValue(item4.ID, item4[empName].ToString());
item6.Update();
var item7 = list.Items.Add();
item7[empName] = "Test";
item7[designation] = "XYZ";
item7.Update();
}
Following code is added in FeatureDeactivating method. This will ensure that the list is deleted when the feature is deactivated.
You can comment out this code, if you want the list to remain after deactivation.
public override void FeatureDeactivating(SPFeatureReceiverProperties properties)
{
var web = properties.Feature.Parent as SPWeb;
if (web == null)
return;
var list = web.Lists.TryGetList("OrgChart");
list.Delete();
}
Visual Web Part: OrgChartWebPartUserControl.ascx
This has the code to retrieve data from list and format as required by the JQuery Plugin.
Code behind:
public partial class OrgChartWebPartUserControl : UserControl
{
string orgDOMstart = "Html Format issue - Downlod code and check";
string orgBody = string.Empty;
string orgDOMEnd = "";
string childRecords = string.Empty;
List<splistitem> listItems = null;
string image = string.Empty;
protected void Page_Load(object sender, EventArgs e)
{
BuildOrgChart();
}
private void BuildOrgChart()
{
string spWebUrl = SPContext.Current.Web.Url;
using (var site = new SPSite(spWebUrl))
{
var web = site.RootWeb;
var list = web.Lists.TryGetList("OrgChart");
listItems = GetListItemsAsList(list.Items);
}
var topLevelNodes = listItems.Where(i => ((i["Manager"] == null) ? "" : i["Manager"].ToString()) == "").ToList<splistitem>();
foreach (var item in topLevelNodes)
{
GenerateChilRecords(item);
orgBody = orgBody + childRecords;
childRecords = string.Empty;
}
lblChartHtml.Text = orgDOMstart + orgBody + orgDOMEnd;
}
private void GenerateChilRecords(SPListItem item)
{
var empReportees = listItems.Where(i => ((i["Manager"] == null) ? "" : i["Manager"].ToString().Split('#')[1]) == item["Employee"].ToString()).ToList<splistitem>();
if (empReportees.Count > 0)
{
image = "Collapse Image - Downlod code and check";
}
childRecords = childRecords + "<li>" + image + " <span style="font-size: 0.9em;">" + item["Employee"] + "</span>
<span style="font-size: 0.8em;"> " + item["Designation"] + "</span>";
if (empReportees.Count > 0)
{
childRecords = childRecords + "<ul>";
foreach (var employee in empReportees)
{
GenerateChilRecords(employee);
}
childRecords = childRecords + "</ul></li>";
}
else
{
childRecords = childRecords + "";
return;
}
}
private List<splistitem> GetListItemsAsList(SPListItemCollection liCol)
{
List<splistitem> toReturn = new List<splistitem>();
foreach (SPListItem li in liCol)
{
toReturn.Add(li);
}
return toReturn;
}
}
</splistitem></splistitem></splistitem></returns></splistitem></splistitem></splistitem>
HTML:
<link href="../../../_layouts/OrgChart/Include/prettify.css" rel="stylesheet" type="text/css" />
<script type="text/javascript">
jQuery(document).ready(function () {
$("#org").jOrgChart({
chartElement: '#chart',
dragAndDrop: true
});
});
var mynode;
function fnExpand(node) {
if (image.attr("src") == '../../../_layouts/OrgChart/Img/Collapse.png') {
image.attr("src", '../../../_layouts/OrgChart/Img/Expand.png')
}
else {
image.attr("src", '../../../_layouts/OrgChart/Img/Collapse.png')
}
}
</script>
<asp:label id="lblChartHtml" runat="server">
Code has been improvised to add expand and collapse images.
There are other ways to create org charts including the COTS products. If you are looking for a simple solution, then you can try this and improvise.