What is enum?
- The enum keyword is used to declare an enumeration
- A distinct type consisting of a set of named constants called the enumerator list
- Every enumeration type has an underlying type
- The default underlying type of the enumeration elements is int
- By default, the first enumerator has the value 0
- And the value of each successive enumerator is increased by 1
- EF 5 enum can have the Types as Byte, Int16, Int32, Int64 or SByte
- You can download the Source code Here from the Original Article
Let's take a simple example
E.g. 1 :
enum Days {Sat, Sun, Mon, Tue, Wed, Thu, Fri};
In this enumeration, Sat is 0, Sun is 1, Mon is 2, and so forth
E.g. 2 : Enumerators can have initializers to override the default values
enum Days {Sat=1, Sun, Mon, Tue, Wed, Thu, Fri};
In this enumeration, the sequence of elements is forced to start from 1 instead of 0
How to Use enum with Entity Framework 5 Code First ?
- This feature is Latest improvement of EF 5
Step 1 : Open Visual Studio 2012
Step 2 : File ==> New Project as EF5EnumSupport
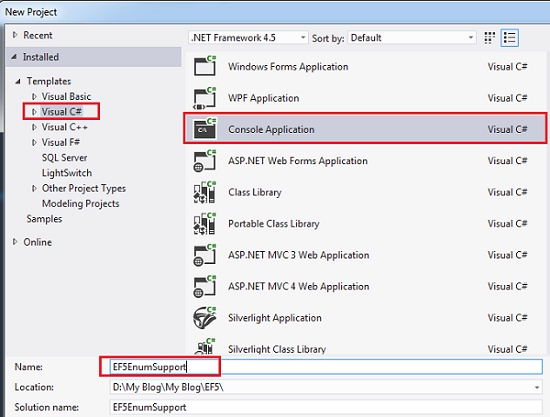
Step 3 : Right Click Your Solution and then Click Manage NuGet Packages...
Step 4 : Click Online Tab and Then Type Entity Framework 5 on Search Box
After that Select EntityFramework and Click Install
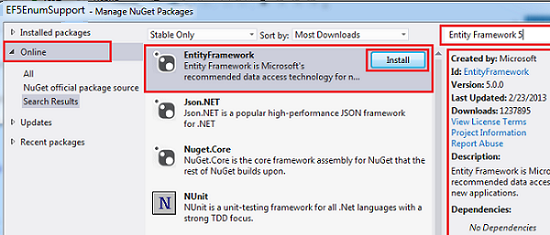
Step 5 : Click I Accept
After the above Step, below mentioned DLLs (Red Color) have added to the Project
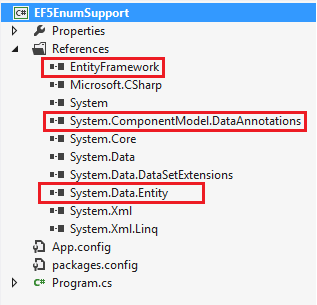
Step 6 : Add ==> New Class and make it as enum Type Course
Solution Explorer
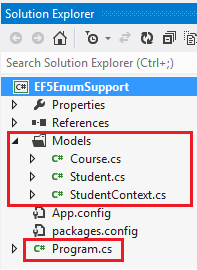
Course.cs
namespace EF5EnumSupport.Models
{
public enum Course
{
Mcsd = 1,
Mcse,
Mcsa,
Mcitp
}
}
Step 7 : Add ==> New Class as Student
Student.cs
namespace EF5EnumSupport.Models {
public class Student
{
public int Id { get; set; }
public string Name { get; set; }
public Course Course { get; set; }
}
}
Step 8 : Create DbContext
derived Class as StudentContext
StudentContext.cs
using System.Data.Entity;
namespace EF5EnumSupport.Models
{
public class StudentContext : DbContext
{
public DbSet<Student> Students { get; set; }
}
}
Step 9 : Console application's Main Method is as below
Program.cs
using System;
using System.Linq;
using EF5EnumSupport.Models;
namespace EF5EnumSupport
{
public class Program
{
static void Main(string[] args)
{
using (var context = new StudentContext())
{
context.Students.Add(new Student { Name = "Sampath Lokuge",
Course = Course.Mcsd });
context.SaveChanges();
var studentQuery = (from d in context.Students
where d.Course == Course.Mcsd
select d).FirstOrDefault();
if (studentQuery != null)
{
Console.WriteLine("Student Id: {0} Name: {1} Course : {2}",
studentQuery.Id, studentQuery.Name,
studentQuery.Course); }
}
}
}
}
The brand new enum
support allows you to have enum
properties in your entity classes
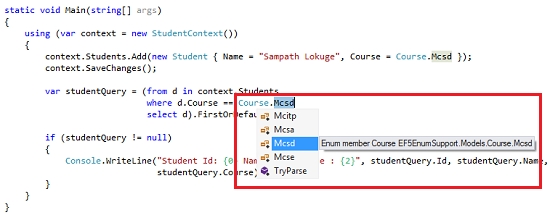
Step 10 : Run the Console Application
DEBUG ==> Start Without Debugging (Ctrl + F5)
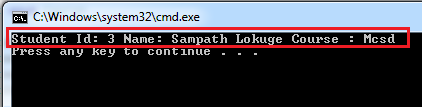
Step 11 : Where's the Database ?
View Data on Students Table
What is LocalDb ?
- Microsoft SQL Server 2012 Express LocalDB is an execution mode of SQL Server Express targeted to program developers
- LocalDB installation copies a minimal set of files necessary to start the SQL Server Database Engine
That's It.You're Done.
Conclusion
- Entity Framework 5 brings a number of improvements and enum Support in Code First is one of them
- You can see that when you use enum type with your model class, Visual Studio 2012 gives intellisense support out of the box
- It's really awesome new feature of the Entity Framework 5
- So Enjoy it
I hope this helps to you. Comments and feedback greatly appreciated.