If you have a Windows Forms application that involves XML editing or viewing, you can use this control to save yourself the effort of formatting the XML content. For now, only syntax highlighting is implemented. I expect to add more features in the future like spacing, grouping, intellisense, etc…
Usage
Simply add the files (XmlToken.cs, XmlTokenizer.cs, XmlEditor.cs, XmlEditor.designer.cs) to your project, then drag and drop the XmlEditor
control from the Toolbox into your Windows Form.

The XmlEditor
control currently has three public
properties. Use AllowXmlFormatting
to enable or disable formatting on the XML content in the editor. The ReadOnly
property tells whether or not to allow the user to change the text. The Text
property sets or gets the text of the XMLeditor
.
Here is how the control looks like when AllowXmlFormatting = true
and ReadOnly = false
(default values):

Implementation
To color the XML string, we have to split it into multiple tokens, then color each token based on its type. I have identified the following token types (based on syntax highlighting behavior in Visual Studio 2008):
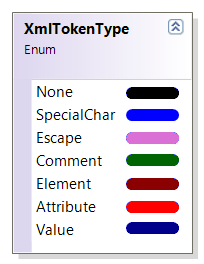
- A “Value” is anything between double quotes
- A “Comment” is anything that starts with <!– and ends with –> (or starts with <!– and is never closed with –>)
- An “Element” is any letter or digit that falls between < and a space or >
- An “Attribute” is any letter or digit that falls after a < followed by space and not closed by >
- An “Escape” is anything that starts with & and ends with ; (For example "e;)
- A “SpecialChar” is any character that is not a letter or a digit
- A “None” is anything else
The Tokenize() public static
method of the XmlTokenizer
class does the job of splitting a string
into XML tokens.

An XmlToken
object is a representation of an XML token with details about the exact text of that token, its location in the string
and its type.

Here is the code in the XmlEditor
control that does the syntax highlighting:
List<XmlToken> tokens = XmlTokenizer.Tokenize(xmlEditor.Text);
foreach (XmlToken token in tokens)
{
xmlEditor.Select(token.Index, token.Text.Length);
switch (token.Type)
{
case XmlTokenType.Attribute:
xmlEditor.SelectionColor = Color.Red;
break;
case XmlTokenType.Comment:
xmlEditor.SelectionColor = Color.DarkGreen;
break;
}
}
You can look at the code on my GitHub page.
Filed under: csharp, WinForms, XML

CodeProject