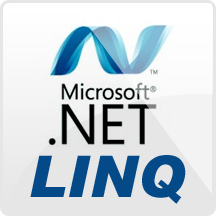
CodeProject
In the last blog post, we discussed about Click Event and Change Event in jQuery. You can read that article here. In this article, we will go over Element Operations in LINQ.
Different Element Operations in LINQ are as follows:
ElementAt
/ElementAtOrDefault
- Returns the element at a specified index
- Look at the example code below. We have a sequence of 2
string
s, Hello
and World
. If we write notEmpty.ElementAt(1)
, we will get World
as output. So this is zero based indexing and World
is the element at position 1
.
First
/FirstOrDefault
- Returns the first element of a collection
- You may use this Element Operation quite frequently with queries, particularly when working with databases. So using this operation, instead of getting back an
IEnumerable
collection, we will just get back one element reference.
Last
/LastOrDefault
– Returns the last element of a collection Single
/SingleOrDefault
- Returns a single element
- This Element Operator returns a single element instead of getting
IEnumerable
collection. So it is almost similar to First
/FirstOrDefault
operator. The primary difference is that, with Single
, if there isn’t just one result in the sequence, it will throw an exception.
Example
sting[] empty={ };
sting[] notEmpty={ "Hello","World"};
var result=empty.FirstOrDefault();
result=notEmpty.Last();
result=notEmpty.ElementAt(1);
result=empty.First();
result=notEmpty.Single();
result=notEmpty.First(s=>s.StartsWith("W"));
Reference
