This article has the goal to show a sample to list data, sort data, search data and add pagination.
First, install the nuget package for PagedList.MVC.
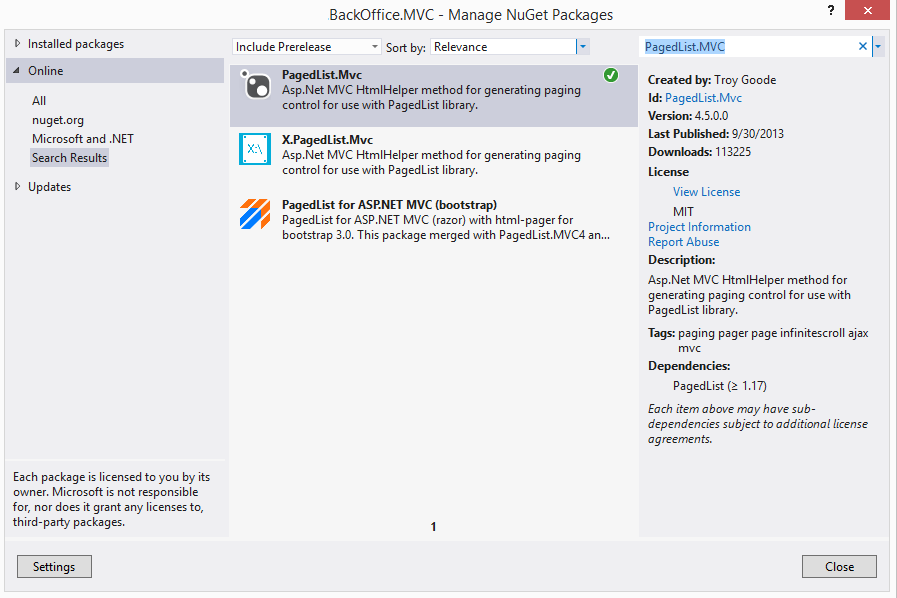
Complete Code
The controller
products
:
public class ProductsController : Controller
{
private readonly ICommandBus _commandBus;
private readonly IBrandRepository _brandRepository;
private readonly ICategoryRepository _categoryRepository;
private readonly IProductRepository _productRepository;
private readonly IProductImageRepository _productImageRepository;
private readonly IUserRepository _userRepository;
private const int PageSize = 10;
public ProductsController(ICommandBus commandBus,
IBrandRepository brandRepository,
ICategoryRepository categoryRepository,
IProductRepository productRepository,
IProductImageRepository productImageRepository,
IUserRepository userRepository)
{
_commandBus = commandBus;
_brandRepository = brandRepository;
_categoryRepository = categoryRepository;
_productRepository = productRepository;
_productImageRepository = productImageRepository;
_userRepository = userRepository;
}
public ActionResult Index(string sortOrder, string currentFilter,
string searchByName,string searchByDescription, string searchByUser,
string searchByBrand, string searchByCategory, int? page)
{
if (searchByName != null)
{
page = 1;
}
else
{
searchByName = currentFilter;
}
var efmvcUser = HttpContext.User.GetEFMVCUser();
var user = _userRepository.GetById(efmvcUser.UserId);
SetCollectionForFilter(user);
ViewBag.CurrentSort = sortOrder;
ViewBag.NameSortParm = String.IsNullOrEmpty(sortOrder) ? "NameDesc" : "";
ViewBag.DescriptionSortParm = sortOrder ==
"Description" ? "DescriptionDesc" : "Description";
ViewBag.UserSortParm = sortOrder == "User" ? "UserDesc" : "User";
ViewBag.PriceSortParm = sortOrder == "Price" ? "PriceDesc" : "Price";
ViewBag.CategorySortParm = sortOrder == "Category" ? "CategoryDesc" : "Category";
ViewBag.BrandSortParm = sortOrder == "Brand" ? "BrandDesc" : "Brand";
ViewBag.CurrentFilter = searchByName;
ViewBag.DescriptionFilter = searchByDescription;
ViewBag.UserParam = searchByUser;
ViewBag.BrandParam = searchByBrand;
ViewBag.CategoryParm = searchByCategory;
int pageIndex = 1;
pageIndex = page.HasValue ? Convert.ToInt32(page) : 1;
IList<Product> products = _productRepository.GetMany
(b => b.CompanyId == user.CompanyId).ToList();
if (!String.IsNullOrEmpty(searchByName))
{
products = products.Where(b => b.Name.ToUpper().Contains
(searchByName.ToUpper())).ToList();
}
if (!String.IsNullOrEmpty(searchByDescription))
{
products = products.Where(b => b.Description.ToUpper().Contains
(searchByDescription.ToUpper())).ToList();
}
if (!string.IsNullOrEmpty(searchByUser))
{
products = products.Where(x => x.User.DisplayName == searchByUser).ToList();
}
if (!string.IsNullOrEmpty(searchByBrand))
{
products = products.Where(x => x.Brand.Name == searchByBrand).ToList();
}
if (!string.IsNullOrEmpty(searchByCategory))
{
products = products.Where(x => x.Category.Name == searchByCategory).ToList();
}
IPagedList<Product> productsToReturn = null;
switch (sortOrder)
{
case "NameDesc":
productsToReturn = products.OrderByDescending
(b => b.Name).ToPagedList(pageIndex, PageSize);
break;
case "User":
productsToReturn = products.OrderBy
(b => b.User.DisplayName).ToPagedList(pageIndex, PageSize);
break;
case "UserDesc":
productsToReturn = products.OrderByDescending
(b => b.User.DisplayName).ToPagedList(pageIndex, PageSize);
break;
case "Description":
productsToReturn = products.OrderBy
(b => b.Description).ToPagedList(pageIndex, PageSize);
break;
case "DescriptionDesc":
productsToReturn = products.OrderByDescending
(b => b.Description).ToPagedList(pageIndex, PageSize);
break;
case "Price":
productsToReturn = products.OrderBy
(b => b.Price).ToPagedList(pageIndex, PageSize);
break;
case "PriceDesc":
productsToReturn = products.OrderByDescending
(b => b.Price).ToPagedList(pageIndex, PageSize);
break;
case "Brand":
productsToReturn = products.OrderBy
(b => b.Brand.Name).ToPagedList(pageIndex, PageSize);
break;
case "BrandDesc":
productsToReturn = products.OrderByDescending
(b => b.Brand.Name).ToPagedList(pageIndex, PageSize);
break;
case "Category":
productsToReturn = products.OrderBy
(c => c.Category.Name).ToPagedList(pageIndex, PageSize);
break;
case "CategoryDesc":
productsToReturn = products.OrderByDescending
(c => c.Category.Name).ToPagedList(pageIndex, PageSize);
break;
default:
productsToReturn = products.OrderBy
(b => b.Name).ToPagedList(pageIndex, PageSize);
break;
}
return View(productsToReturn);
}
private void SetCollectionForFilter(User user)
{
var users = new List<string>();
var usersQuery = from d in _userRepository.GetMany(b => b.CompanyId == user.CompanyId)
orderby d.DisplayName
select d.DisplayName;
users.AddRange(usersQuery.Distinct());
ViewBag.SearchByUser = new SelectList(users);
var brands = new List<string>();
var brandsQuery = from brand in _brandRepository.GetMany
(b => b.CompanyId == user.CompanyId)
orderby brand.Name
select brand.Name;
brands.AddRange(brandsQuery.Distinct());
ViewBag.SearchByBrand = new SelectList(brands);
var categories = new List<string>();
var categoriesQuery = from category in _categoryRepository.GetMany
(b => b.CompanyId == user.CompanyId)
orderby category.Name
select category.Name;
categories.AddRange(categoriesQuery.Distinct());
ViewBag.SearchByCategory = new SelectList(categories);
}
The View – Index:
@model IEnumerable<BackOffice.Model.Entities.Product>
@{
ViewBag.Title = "Products";
}
<h2>Products</h2>
<div id="divProductList">
@Html.Partial("_ProductList",Model)
</div>
The partial view: _ProductsList
:
@using EFMVC.Web.Core.Extensions
@using PagedList.Mvc
@model PagedList.IPagedList<BackOffice.Model.Entities.Product>
<script type="text/javascript">
$(document).ready(function () {
$("tr:even").css("background-color", "#A8A8A8");
$("tr:odd").css("background-color", "#FFFFFF");
});
</script>
@{
var user = HttpContext.Current.User.GetEFMVCUser();
}
<p>
@Html.ActionLink("Create new product","Create")
</p>
<div>
@using (Html.BeginForm("Index", "Products", FormMethod.Get))
{
<p>
<b>Filter by:</b><br/>
<b>Name</b> @Html.TextBox("SearchByName", ViewBag.CurrentFilter as string)
<b>Description</b>
@Html.TextBox("SearchByDescription", ViewBag.DescriptionFilter as string)
<br/>
<b>Category</b>
@Html.DropDownList("SearchByCategory", "All categories")
<b>Brand</b>
@Html.DropDownList("SearchByBrand", "All brands")
@if (user.RoleName == EFMVC.Web.Core.Models.Roles.Admin)
{
<b>User</b>
@Html.DropDownList("SearchByUser", "All users")
}
<input type="submit" value="Search" />
</p>
}
</div>
@if(Model.Count>0)
{
<table>
<tr>
<th style="width:100px">
@Html.ActionLink("Name", "Index", new { sortOrder = ViewBag.NameSortParm,
currentFilter=ViewBag.CurrentFilter,
searchByDescription =
ViewBag.DescriptionFilter,
searchByUser=ViewBag.UserParam,
searchByBrand=ViewBag.BrandParam,
searchByCategory = ViewBag.CategoryParam})
</th>
<th style="width:150px">@Html.ActionLink("Description", "Index", new {
sortOrder = ViewBag.DescriptionSortParm,
currentFilter=ViewBag.CurrentFilter,
searchByDescription =
ViewBag.DescriptionFilter,
searchByUser=ViewBag.UserParam,
searchByBrand=ViewBag.BrandParam,
searchByCategory =
ViewBag.CategoryParam})</th>
<th style="width:100px">@Html.ActionLink("Category", "Index", new {
sortOrder = ViewBag.CategorySortParm,
currentFilter=ViewBag.CurrentFilter,
searchByDescription =
ViewBag.DescriptionFilter,
searchByUser=ViewBag.UserParam,
searchByBrand=ViewBag.BrandParam,
searchByCategory =
ViewBag.CategoryParam})</th>
<th style="width:100px">@Html.ActionLink("Brand", "Index", new {
sortOrder = ViewBag.BrandSortParm,
currentFilter=ViewBag.CurrentFilter,
searchByDescription =
ViewBag.DescriptionFilter,
searchByUser=ViewBag.UserParam,
searchByBrand=ViewBag.BrandParam,
searchByCategory =
ViewBag.CategoryParam})</th>
<th style="width:100px">@Html.ActionLink("Price", "Index", new {
sortOrder = ViewBag.PriceSortParm,
currentFilter=ViewBag.CurrentFilter,
searchByDescription =
ViewBag.DescriptionFilter,
searchByUser=ViewBag.UserParam,
searchByBrand=ViewBag.BrandParam,
searchByCategory =
ViewBag.CategoryParam})</th>
<th>Link</th>
<th>Images</th>
@if (user.RoleName == EFMVC.Web.Core.Models.Roles.Admin)
{
<th style="width:100px">
@Html.ActionLink("User", "Index", new { sortOrder = ViewBag.UserSortParm,
currentFilter=ViewBag.CurrentFilter,
searchByDescription =
ViewBag.DescriptionFilter,
searchByUser=ViewBag.UserParam,
searchByBrand=ViewBag.BrandParam,
searchByCategory = ViewBag.CategoryParam})
</th>
}
<th style="width:120px"></th>
</tr>
@foreach (var item in Model)
{
<tr>
<td>
@item.Name
</td>
<td>
@item.ShortDescription
</td>
<td>
<a href="@Url.Content("/Categories/Details/" + @item.Category.Id)"
target="_blank">@item.Category.Name</a>
</td>
<td>
<a href="@Url.Content("/Brands/Details/" + @item.Brand.Id)"
target="_blank">@item.Brand.Name</a>
</td>
<td>
@item.PriceToString
</td>
<td>
<a href="@item.Link" target="_blank">link</a>
</td>
<td>
<img width="100" src='@Url.Action
("getimage/" + @item.Id+"/0", "Products")' />
<img width="100" src='@Url.Action
("getimage/" + @item.Id+"/1", "Products")' />
<img width="100" src='@Url.Action
("getimage/" + @item.Id+"/2", "Products")' />
</td>
@if (user.RoleName == EFMVC.Web.Core.Models.Roles.Admin)
{
<td>@item.User.DisplayName</td>
}
<td>
@Html.ActionLink("Edit", "Edit", new {id = item.Id})
@Html.ActionLink("Details", "Details", new {id = item.Id})
@Ajax.ActionLink("Delete", "Delete", new {id = item.Id},
new AjaxOptions
{
Confirm = "Would you like to delete the selected product?",
HttpMethod = "Post",
UpdateTargetId = "divProductList"
})
</td>
</tr>
}
</table>
<br />
<div id='Paging' style="text-align: center">
Page @(Model.PageCount < Model.PageNumber ? 0 : Model.PageNumber)
of @Model.PageCount
@Html.PagedListPager(Model, page => Url.Action("Index", new {page,
sortOrder = ViewBag.CurrentSort,
currentFilter = ViewBag.CurrentFilter,
searchByDescription =
ViewBag.DescriptionFilter,
searchByUser=ViewBag.UserParam,
searchByBrand=ViewBag.BrandParam,
searchByCategory =
ViewBag.CategoryParam}))
</div>
}
else
{
if (string.IsNullOrEmpty(ViewBag.CurrentFilter) &&
string.IsNullOrEmpty(ViewBag.BrandParam) &&
string.IsNullOrEmpty(ViewBag.CategoryParam &&
string.IsNullOrEmpty(ViewBag.DescriptionFilter)))
{
<p>There aren´t any product created.</p>
}
else
{
<p>There aren´t any product for the selected search.</p>
}
}
Result
