This time, I have very interesting stuff for you guys. Few days ago, I got a tricky requirement from one of my customers. It's about populating their organizational structure dynamically on their SharePoint Intranet site without any server side components /Code. This means – there will be a SharePoint list from where data will be pulled and on a Webpart (CEWP or OOB WP) the hierarchy needs to be displayed. Fortunately, within a few hours, I was able to integrate 2-3 things with some JavaScript / jquery code to accomplish this requirement. Things required to cook out are:
- jQuery
- jQuery OrgChart PlugIn – Kindly read the technical artifacts of jQuery OrgChart from this location.
- Content Editor WebPart
- SharePoint Javascript CSOM
- a SharePoint list
- jQuery Balloon Plug In – If need to show any additional data
The scenario that I am going to demonstrate here is to create a corporate organizational structure. For example, CEO on the top then COO, CFO, CTO then Executive VPs, AVPs then comes Directors, Managers after that core execution team.
So, here we start.
<link rel="stylesheet" href="_layouts/jquery.orgchart.css" /></pre>
<link rel="stylesheet" href="_layouts/jquery-ui-1.10.3.custom.css" />
<script src="_layouts/jquery-1.9.1.js"></script>
<script src="_layouts/jquery-ui-1.10.3.custom.js"></script>
<script src="_layouts/jquery.orgchart.js"></script>
<script src="_layouts/jquery.balloon.js"></script>
<!--This div will be populated with the <ul><li> structure from the SCOM returned objects-->
<div id="left">
</div>
<style>
div.node.bladerunner {
background-color: #a4a0d9 !important;
}
div.node.replicant {
background-color: #d9ada0 !important;
}
div.node.deceased {
background-color: #d9d2a0 !important;
text-decoration: line-through;
}
div.node.retired {
background-color: #d9c0a0 !important;
text-decoration: line-through;
}
</style>
<!--Main Org Structure will pupulate under this DIV-->
<div id="content">
<div id="main">
</div>
</div>
<script type="text/javascript">
ExecuteOrDelayUntilScriptLoaded(loadStructure, "sp.js");
function loadStructure() {
var context = new SP.ClientContext.get_current();
var web = context.get_web();
var list = web.get_lists().getByTitle("Employee");
var viewXml = '<View><RowLimit>1200</RowLimit></View>';
var query = new SP.CamlQuery();
query.set_viewXml(viewXml);
this.items = list.getItems(query);
context.load(items, 'Include(Title, Manager,Designation)');
context.add_requestSucceeded(onLoaded);
context.add_requestFailed(onFailure);
context.executeQueryAsync();
function onLoaded() {
var tasksEntries = [];
var itemsCount = items.get_count();
for (i = 0; i < itemsCount; i++) {
var item = items.itemAt(i);
var taskEntry = item.get_fieldValues();
tasksEntries.push(taskEntry);
}
var topHead = GetChildArrayObjects(tasksEntries, null);
var headName = topHead[0].Title;
var title = topHead[0].Designation + ", Manager :" + topHead[0].Manager;
var listString = "<ul id='organisation'><li class='hide'
title='" + headName + "'>" + headName;
var childliststring = getChildNodes(tasksEntries, headName, listString);
listString = childliststring + "</li></ul>";
var divForList = document.getElementById('left');
divForList.innerHTML = listString;
$("#organisation").orgChart({ container: $("#main"), nodeClicked: onChartNodeClicked });
for (var i = 0; i < tasksEntries.length; i++) {
$("div[title=\"" + tasksEntries[i].Title + "\"]").balloon({
contents: '<img src ="/Images1/_t/images_jpg.jpg"/></br><ul><li>
Designation :' + tasksEntries[i].Designation + '</li><li>Phone# : 123-234-1233</li></ul>'
});
}
}
function onChartNodeClicked(node) {
}
function onFailure() {
alert('script failed');
}
}
function getChildNodes(tasksEntries, headName, liststring) {
var childs = GetChildArrayObjects(tasksEntries, headName);
if (childs.length > 0) {
liststring = liststring + "<ul>";
for (var cnt = 0; cnt < childs.length; cnt++) {
var head = childs[cnt].Title;
var title = childs[cnt].Designation + ", Manager :" + childs[cnt].Manager;
liststring = liststring + "<li class='bladerunner' title='" +
head + "'>" + head;
liststring = getChildNodes(tasksEntries, head, liststring);
liststring = liststring + "</li>";
}
liststring = liststring + "</ul>";
}
return liststring;
}
function GetChildArrayObjects(items, manager) {
var newArray = [];
for (var i = 0; i < items.length; i++) {
var item = items[i];
if (item.Manager == manager) {
newArray.push(item);
}
}
return newArray;
}
</script>
JQuery OrgChart basically needs a <ul><li>
(unordered list) format for populating the Org-Chart. So I am querying the SP List using the SCOM (JavaScript) and using a recursive function (getChildNodes
) the <ul><li>
structure is created. After loading this <ul><li>
structure I call the jquery orgchart function to populate the hierarchy on the other <div>
.
The main checkpoints on the above script are:
for (var i = 0; i < tasksEntries.length; i++) {
$("div[title=\"" + tasksEntries[i].Title + "\"]").balloon({
contents: '<img src ="/Images1/_t/images_jpg.jpg"/>
</br><ul><li> Designation :' +
tasksEntries[i].Designation + '</li><li>Phone# : 123-234-1233</li></ul>'
});
Finally, the OrgChart is populated as displayed below. You can see a <ul><li>
list on the top, which can be made to visible=false
to the <DIV>
with ID
‘left’ on the above mentioned script, displayed only for testing purposes.
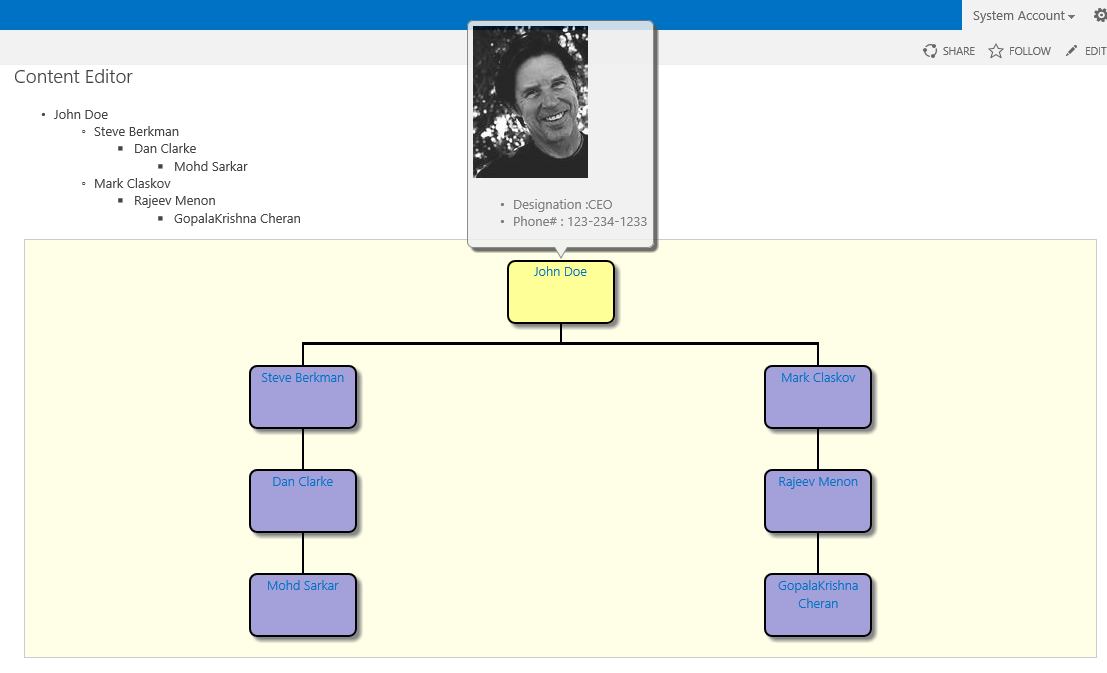
Hope you have enjoyed this article. Kindly let me know if there are any issues .
- Create a custom SharePoint list having columns like
Title
, Manager
, Designation
. Enter some logical details as mentioned below.

- Download and place Jquery & jQuery OrgChart plug-in files into the hive’s _layouts folder (see image below). Note: When we are going through a solution deployment approach, we need to place these files inside SP Project to package it to a WSP file. Here, we are directly copying files to _layouts folder just for the sake of demo. If needed, you can place the jQuery Balloon file too.

- Creating the Content Editor WebPart / Script editor WP – Script source file. Save the below mentioned code to a txt file and upload it to the
SiteAssets
Library. Give the path of the file to CEWP like ”/SiteAssets/ScriptstxtFile.txt” or directly use this script to your client component.
loadStructure()
– JS Function uses SharePoint JS SCOM to Query the list to get the Parent-Child Hierarchy. GetChildArrayObjects()
– it's an alternative to .filter
JS function since this is not supported on IE8. getChildNodes
– Recursive function mentioned above – which basically structures the unordered list (see the <ul>
mentioned on image below) $(“#organisation”).orgChart({ container: $(“#main”), nodeClicked: onChartNodeClicked });
– This line of jQuery code in the above script actually reads the <ul><oli>
and generates the OrgChart. While you skim the above code, you will be able to see a <ul>
with ID as ‘organisation’ created which is read and org chart is populated to a <DIV>
‘main’. - After creating the orgChart, I am iterating through the
<DIV>
of the OrgChart block according to its ID to enable the balloon