Introduction
If you want to make you're own game, then here's a simple one, even though it is advanced for a simple game. Its Tic-Tac-Toe for Visual Studio. Just follow these steps and you will successfully make you're own AMAZING TIC-TAC-TOE game. Just download the link until I say that I am completely done
Background
For this project, you will need to understand the 5 basic data types and the Boolean data type. You will need to get used to most of the basic tools that you can use on your form like the option-box, command button, etc. So let's get started.
Using the code
We will start out by making the player vs player game, since it is easier.
First, open visual basic 6 and open up a form. This will be like a main menu form. Next, change the form's name to "frmStartScreen".
You will need these objects for the starting form:
- 2 TextBoxes
- 2 LabelBox
- 4 Command Buttons
Label one TextBox as "txtPlay1" and the other one as "txtPlay2" label the Command Buttons as "cmdStart", "cmdInstruct", "cmdAIgame", and "cmdQuit"
Here is the code for the "cmdInstruct" button
Private Sub cmdInstruct_Click()
frmInstructions.Show
End Sub
And now for the "cmdStart" button
Private Sub cmdStart_Click()
frmTicTacToe.Show
frmTicTacToe.txtPlayer1.Text = txtPlay1.Text
frmTicTacToe.txtPlayer2.Text = txtPlay2.Text
frmTicTacToe.lblTurn.Caption = frmTicTacToe.txtPlayer1.Text
End Sub
For "cmdAIgame"
Private Sub cmdAIgame_Click()
frmPvAIWelcome.Show
frmPvAI.txtPlayer.Text = txtPlay1.Text
End Sub
And for the "cmdQuit" button.
Private Sub cmdQuit_Click()
End
End Sub
This is what the form should look like:

Now open up a second form, which will be named "frmInstructions".
You will need: 1 labelbox, and 1 command button
Insert all of this as the caption for the labelbox:
The rules are simple. Enter your name(s) in the textbox(es) in the screen. Make sure you have a different name as your opponent or the game won't work properly. Decide who is player 1 and who is player 2. Then play the game. After the round is over. Press Clear to start a new game and press Rematch to play another game. With a rematch, player 2 will go first in the second game, player 1 in the 3rd and so on. If you are playing AI, then press PvAI in the start screen. Be careful, this AI is an expert and will not lose a game if messed with. The same thing will happen with that game. the player goes first and then the AI will go first and so on. To Start Press OK and press the other buttons in the program to begin.
This is what is should look like:
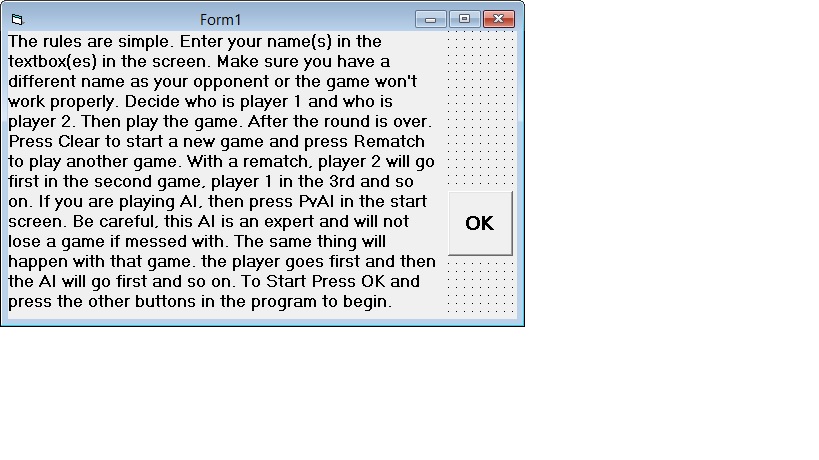
And put this code in for the command button:
Private Sub Command1_Click()
frmInstructions.Hide
End Sub
Now for the big part.
You will need to open up another form and put in:
- 2 textboxes
- 27 labelboxes
- 12 command buttons
- 1 timer
- 12 lines
These will be the names of the text boxes
These will be the names of the labelboxes
- lblX1
- lblX2
- lblX3
- lblX4
- lblX5
- lblX6
- lblX7
- lblX8
- lblX9
- lblO1
- lblO2
- lblO3
- lblO4
- lblO5
- lblO6
- lblO7
- lblO8
- lblO9
- lblTurn
- lblWin1
- lblWin2
- lblTie
These will be the names of the command buttons:
- cmdBox1
- cmdBox2
- cmdBox3
- cmdBox4
- cmdBox5
- cmdBox6
- cmdBox7
- cmdBox8
- cmdBox9
- cmdClear
- cmdRematch
- cmdQuit
The form should look like this:

Here is the entire code for the form:
Sub Xwin()
MsgBox "Congradulations Player 1! You Win!"
lblWin1 = Val(lblWin1.Caption) + 1
cmdRematch.Visible = True
cmdQuit.Visible = True
Call Disable
End Sub
Sub Owin()
MsgBox "Congradulations Player 2! You Win!"
lblWin2 = Val(lblWin2.Caption) + 1
cmdRematch.Visible = True
cmdQuit.Visible = True
Call Disable
End Sub
Sub TieGame()
MsgBox "It's a Tie!"
lblTie = Val(lblTie.Caption) + 1
cmdRematch.Visible = True
cmdQuit.Visible = True
End Sub
Sub endings()
If (lblX1.Visible = True And lblX2.Visible = True And lblX3.Visible = True) Then
Line13.Visible = True
Line13.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblX1.Visible = True And lblX4.Visible = True And lblX7.Visible = True) Then
Line11.Visible = True
Line11.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblX1.Visible = True And lblX5.Visible = True And lblX9.Visible = True) Then
Line12.Visible = True
Line12.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblX2.Visible = True And lblX5.Visible = True And lblX8.Visible = True) Then
Line6.Visible = True
Line6.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblX3.Visible = True And lblX5.Visible = True And lblX7.Visible = True) Then
Line9.Visible = True
Line9.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblX4.Visible = True And lblX5.Visible = True And lblX6.Visible = True) Then
Line10.Visible = True
Line10.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblX3.Visible = True And lblX6.Visible = True And lblX9.Visible = True) Then
Line8.Visible = True
Line8.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblX7.Visible = True And lblX8.Visible = True And lblX9.Visible = True) Then
Line7.Visible = True
Line7.ZOrder (0)
Timer1.Enabled = True
End If
If (lblO1.Visible = True And lblO2.Visible = True And lblO3.Visible = True) Then
Line13.Visible = True
Line13.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblO1.Visible = True And lblO4.Visible = True And lblO7.Visible = True) Then
Line11.Visible = True
Line11.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblO1.Visible = True And lblO5.Visible = True And lblO9.Visible = True) Then
Line12.Visible = True
Line12.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblO2.Visible = True And lblO5.Visible = True And lblO8.Visible = True) Then
Line6.Visible = True
Line6.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblO3.Visible = True And lblO5.Visible = True And lblO7.Visible = True) Then
Line9.Visible = True
Line9.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblO4.Visible = True And lblO5.Visible = True And lblO6.Visible = True) Then
Line10.Visible = True
Line10.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblO3.Visible = True And lblO6.Visible = True And lblO9.Visible = True) Then
Line8.Visible = True
Line8.ZOrder (0)
Timer1.Enabled = True
ElseIf (lblO7.Visible = True And lblO8.Visible = True And lblO9.Visible = True) Then
Line7.Visible = True
Line7.ZOrder (0)
Timer1.Enabled = True
End If
End Sub
Sub reset()
cmdBox1.Caption = ""
cmdBox2.Caption = ""
cmdBox3.Caption = ""
cmdBox4.Caption = ""
cmdBox5.Caption = ""
cmdBox6.Caption = ""
cmdBox7.Caption = ""
cmdBox8.Caption = ""
cmdBox9.Caption = ""
cmdBox1.Enabled = True
cmdBox2.Enabled = True
cmdBox3.Enabled = True
cmdBox4.Enabled = True
cmdBox5.Enabled = True
cmdBox6.Enabled = True
cmdBox7.Enabled = True
cmdBox8.Enabled = True
cmdBox9.Enabled = True
cmdBox1.Visible = True
cmdBox2.Visible = True
cmdBox3.Visible = True
cmdBox4.Visible = True
cmdBox5.Visible = True
cmdBox6.Visible = True
cmdBox7.Visible = True
cmdBox8.Visible = True
cmdBox9.Visible = True
lblX1.Visible = False
lblX2.Visible = False
lblX3.Visible = False
lblX4.Visible = False
lblX5.Visible = False
lblX6.Visible = False
lblX7.Visible = False
lblX8.Visible = False
lblX9.Visible = False
lblO1.Visible = False
lblO2.Visible = False
lblO3.Visible = False
lblO4.Visible = False
lblO5.Visible = False
lblO6.Visible = False
lblO7.Visible = False
lblO8.Visible = False
lblO9.Visible = False
Line6.Visible = False
Line7.Visible = False
Line8.Visible = False
Line9.Visible = False
Line10.Visible = False
Line11.Visible = False
Line12.Visible = False
Line13.Visible = False
Timer1.Enabled = False
End Sub
Sub Disable()
cmdBox1.Enabled = False
cmdBox2.Enabled = False
cmdBox3.Enabled = False
cmdBox4.Enabled = False
cmdBox5.Enabled = False
cmdBox6.Enabled = False
cmdBox7.Enabled = False
cmdBox8.Enabled = False
cmdBox9.Enabled = False
End Sub
Private Sub cmdBox1_Click()
Dim win9
If lblTurn.Caption = txtPlayer1.Text Then
lblTurn.Caption = txtPlayer2.Text
cmdBox1.Enabled = False
cmdBox1.Visible = False
lblX1.Visible = True
ElseIf lblTurn.Caption = txtPlayer2.Text Then
lblTurn.Caption = txtPlayer1.Text
cmdBox1.Enabled = False
cmdBox1.Visible = False
lblO1.Visible = True
End If
If (lblX1.Visible = True And lblX2.Visible = True And lblX3.Visible = True) Or (lblX1.Visible = True And lblX4.Visible = True And lblX7.Visible = True) Or (lblX1.Visible = True And lblX5.Visible = True And lblX9.Visible = True) Then
Call Xwin
Call endings
ElseIf (lblO1.Visible = True And lblO2.Visible = True And lblO3.Visible = True) Or (lblO1.Visible = True And lblO4.Visible = True And lblO7.Visible = True) Or (lblO1.Visible = True And lblO5.Visible = True And lblO9.Visible = True) Then
Call Owin
Call endings
ElseIf tie Then
Call TieGame
End If
End Sub
Private Sub cmdBox2_Click()
If lblTurn.Caption = txtPlayer1.Text Then
lblTurn.Caption = txtPlayer2.Text
cmdBox2.Enabled = False
cmdBox2.Visible = False
lblX2.Visible = True
ElseIf lblTurn.Caption = txtPlayer2.Text Then
lblTurn.Caption = txtPlayer1.Text
cmdBox2.Enabled = False
cmdBox2.Visible = False
lblO2.Visible = True
End If
If (lblX1.Visible = True And lblX2.Visible = True And lblX3.Visible = True) Or (lblX2.Visible = True And lblX5.Visible = True And lblX8.Visible = True) Then
Call Xwin
Call endings
ElseIf (lblO1.Visible = True And lblO2.Visible = True And lblO3.Visible = True) Or (lblO2.Visible = True And lblO5.Visible = True And lblO8.Visible = True) Then
Call Owin
Call endings
ElseIf cmdBox1.Enabled = False And cmdBox2.Enabled = False And cmdBox3.Enabled = False And cmdBox4.Enabled = False And cmdBox5.Enabled = False And cmdBox6.Enabled = False And cmdBox7.Enabled = False And cmdBox8.Enabled = False And cmdBox9.Enabled = False Then
Call TieGame
End If
End Sub
Private Sub cmdBox3_Click()
If lblTurn.Caption = txtPlayer1.Text Then
lblTurn.Caption = txtPlayer2.Text
cmdBox3.Enabled = False
cmdBox3.Visible = False
lblX3.Visible = True
ElseIf lblTurn.Caption = txtPlayer2.Text Then
lblTurn.Caption = txtPlayer1.Text
cmdBox3.Enabled = False
cmdBox3.Visible = False
lblO3.Visible = True
End If
If (lblX1.Visible = True And lblX2.Visible = True And lblX3.Visible = True) Or (lblX3.Visible = True And lblX5.Visible = True And lblX7.Visible = True) Or (lblX4.Visible = True And lblX5.Visible = True And lblX6.Visible = True) Then
Call Xwin
Call endings
ElseIf (lblO1.Visible = True And lblO2.Visible = True And lblO3.Visible = True) Or (lblO3.Visible = True And lblO5.Visible = True And lblO7.Visible = True) Or (lblO4.Visible = True And lblO5.Visible = True And lblO6.Visible = True) Then
Call Owin
Call endings
ElseIf cmdBox1.Enabled = False And cmdBox2.Enabled = False And cmdBox3.Enabled = False And cmdBox4.Enabled = False And cmdBox5.Enabled = False And cmdBox6.Enabled = False And cmdBox7.Enabled = False And cmdBox8.Enabled = False And cmdBox9.Enabled = False Then
Call TieGame
End If
End Sub
Private Sub cmdBox4_Click()
If lblTurn.Caption = txtPlayer1.Text Then
cmdBox4.Caption = "X"
lblTurn.Caption = txtPlayer2.Text
cmdBox4.Enabled = False
cmdBox4.Visible = False
lblX4.Visible = True
ElseIf lblTurn.Caption = txtPlayer2.Text Then
cmdBox4.Caption = "O"
lblTurn.Caption = txtPlayer1.Text
cmdBox4.Enabled = False
cmdBox4.Visible = False
lblO4.Visible = True
End If
If (lblX1.Visible = True And lblX4.Visible = True And lblX7.Visible = True) Or (lblX4.Visible = True And lblX5.Visible = True And lblX6.Visible = True) Then
Call Xwin
Call endings
ElseIf (lblO1.Visible = True And lblO4.Visible = True And lblO7.Visible = True) Or (lblO4.Visible = True And lblO5.Visible = True And lblO6.Visible = True) Then
Call Owin
Call endings
ElseIf cmdBox1.Enabled = False And cmdBox2.Enabled = False And cmdBox3.Enabled = False And cmdBox4.Enabled = False And cmdBox5.Enabled = False And cmdBox6.Enabled = False And cmdBox7.Enabled = False And cmdBox8.Enabled = False And cmdBox9.Enabled = False Then
Call TieGame
End If
End Sub
Private Sub cmdBox5_Click()
If lblTurn.Caption = txtPlayer1.Text Then
cmdBox5.Caption = "X"
lblTurn.Caption = txtPlayer2.Text
cmdBox5.Enabled = False
cmdBox5.Visible = False
lblX5.Visible = True
ElseIf lblTurn.Caption = txtPlayer2.Text Then
cmdBox5.Caption = "O"
lblTurn.Caption = txtPlayer1.Text
cmdBox5.Enabled = False
cmdBox5.Visible = False
lblO5.Visible = True
End If
If (lblX1.Visible = True And lblX5.Visible = True And lblX9.Visible = True) Or (lblX2.Visible = True And lblX5.Visible = True And lblX8.Visible = True) Or (lblX3.Visible = True And lblX5.Visible = True And lblX7.Visible = True) Or (lblX4.Visible = True And lblX5.Visible = True And lblX6.Visible = True) Then
Call Xwin
Call endings
ElseIf (lblO1.Visible = True And lblO5.Visible = True And lblO9.Visible = True) Or (lblO2.Visible = True And lblO5.Visible = True And lblO8.Visible = True) Or (lblO3.Visible = True And lblO5.Visible = True And lblO7.Visible = True) Or (lblO4.Visible = True And lblO5.Visible = True And lblO6.Visible = True) Then
Call Owin
Call endings
ElseIf cmdBox1.Enabled = False And cmdBox2.Enabled = False And cmdBox3.Enabled = False And cmdBox4.Enabled = False And cmdBox5.Enabled = False And cmdBox6.Enabled = False And cmdBox7.Enabled = False And cmdBox8.Enabled = False And cmdBox9.Enabled = False Then
Call TieGame
End If
End Sub
Private Sub cmdBox6_Click()
If lblTurn.Caption = txtPlayer1.Text Then
cmdBox6.Caption = "X"
lblTurn.Caption = txtPlayer2.Text
cmdBox6.Enabled = False
cmdBox6.Visible = False
lblX6.Visible = True
ElseIf lblTurn.Caption = txtPlayer2.Text Then
cmdBox6.Caption = "O"
lblTurn.Caption = txtPlayer1.Text
cmdBox6.Enabled = False
cmdBox6.Visible = False
lblO6.Visible = True
End If
If (lblX4.Visible = True And lblX5.Visible = True And lblX6.Visible = True) Or (lblX4.Visible = True And lblX5.Visible = True And lblX6.Visible = True) Then
Call Xwin
Call endings
ElseIf (lblO4.Visible = True And lblO5.Visible = True And lblO6.Visible = True) Or (lblO3.Visible = True And lblO6.Visible = True And lblO9.Visible = True) Then
Call Owin
Call endings
ElseIf cmdBox1.Enabled = False And cmdBox2.Enabled = False And cmdBox3.Enabled = False And cmdBox4.Enabled = False And cmdBox5.Enabled = False And cmdBox6.Enabled = False And cmdBox7.Enabled = False And cmdBox8.Enabled = False And cmdBox9.Enabled = False Then
Call TieGame
End If
End Sub
Private Sub cmdBox7_Click()
If lblTurn.Caption = txtPlayer1.Text Then
cmdBox7.Caption = "X"
lblTurn.Caption = txtPlayer2.Text
cmdBox7.Enabled = False
cmdBox7.Visible = False
lblX7.Visible = True
ElseIf lblTurn.Caption = txtPlayer2.Text Then
cmdBox7.Caption = "O"
lblTurn.Caption = txtPlayer1.Text
cmdBox7.Enabled = False
cmdBox7.Visible = False
lblO7.Visible = True
End If
If (lblX1.Visible = True And lblX4.Visible = True And lblX7.Visible = True) Or (lblX3.Visible = True And lblX5.Visible = True And lblX7.Visible = True) Or (lblX7.Visible = True And lblX8.Visible = True And lblX9.Visible = True) Then
Call Xwin
Call endings
ElseIf (lblO1.Visible = True And lblO4.Visible = True And lblO7.Visible = True) Or (lblO3.Visible = True And lblO5.Visible = True And lblO7.Visible = True) Or (lblO7.Visible = True And lblO8.Visible = True And lblO9.Visible = True) Then
Call Owin
Call endings
ElseIf cmdBox1.Enabled = False And cmdBox2.Enabled = False And cmdBox3.Enabled = False And cmdBox4.Enabled = False And cmdBox5.Enabled = False And cmdBox6.Enabled = False And cmdBox7.Enabled = False And cmdBox8.Enabled = False And cmdBox9.Enabled = False Then
Call TieGame
End If
End Sub
Private Sub cmdBox8_Click()
If lblTurn.Caption = txtPlayer1.Text Then
cmdBox8.Caption = "X"
lblTurn.Caption = txtPlayer2.Text
cmdBox8.Enabled = False
cmdBox8.Visible = False
lblX8.Visible = True
ElseIf lblTurn.Caption = txtPlayer2.Text Then
cmdBox8.Caption = "O"
lblTurn.Caption = txtPlayer1.Text
cmdBox8.Enabled = False
cmdBox8.Visible = False
lblO8.Visible = True
End If
If (lblX2.Visible = True And lblX5.Visible = True And lblX8.Visible = True) Or (lblX7.Visible = True And lblX8.Visible = True And lblX9.Visible = True) Then
Call Xwin
Call endings
ElseIf (lblO2.Visible = True And lblO5.Visible = True And lblO8.Visible = True) Or (lblO7.Visible = True And lblO8.Visible = True And lblO9.Visible = True) Then
Call Owin
Call endings
ElseIf cmdBox1.Enabled = False And cmdBox2.Enabled = False And cmdBox3.Enabled = False And cmdBox4.Enabled = False And cmdBox5.Enabled = False And cmdBox6.Enabled = False And cmdBox7.Enabled = False And cmdBox8.Enabled = False And cmdBox9.Enabled = False Then
Call TieGame
End If
End Sub
Private Sub cmdBox9_Click()
If lblTurn.Caption = txtPlayer1.Text Then
cmdBox9.Caption = "X"
lblTurn.Caption = txtPlayer2.Text
cmdBox9.Enabled = False
cmdBox9.Visible = False
lblX9.Visible = True
ElseIf lblTurn.Caption = txtPlayer2.Text Then
cmdBox9.Caption = "O"
lblTurn.Caption = txtPlayer1.Text
cmdBox9.Enabled = False
cmdBox9.Visible = False
lblO9.Visible = True
End If
If (lblX1.Visible = True And lblX5.Visible = True And lblX9.Visible = True) Or (lblX3.Visible = True And lblX6.Visible = True And lblX9.Visible = True) Or (lblX7.Visible = True And lblX8.Visible = True And lblX9.Visible = True) Then
Call Xwin
Call endings
ElseIf (lblO1.Visible = True And lblO5.Visible = True And lblO9.Visible = True) Or (lblO3.Visible = True And lblO6.Visible = True And lblO9.Visible = True) Or (lblO7.Visible = True And lblO8.Visible = True And lblO9.Visible = True) Then
Call Owin
Call endings
ElseIf cmdBox1.Enabled = False And cmdBox2.Enabled = False And cmdBox3.Enabled = False And cmdBox4.Enabled = False And cmdBox5.Enabled = False And cmdBox6.Enabled = False And cmdBox7.Enabled = False And cmdBox8.Enabled = False And cmdBox9.Enabled = False Then
Call TieGame
End If
End Sub
Private Sub cmdClear_Click()
Call reset
lblWin1.Caption = 0
lblWin2.Caption = 0
txtPlayer1 = ""
txtPlayer2 = ""
lblTurn.Caption = ""
frmTicTacToe.Hide
frmStartScreen.Show
frmStartScreen.txtPlay1.SetFocus
End Sub
Private Sub cmdPlay_Click()
If txtPlayer1 = "" Or txtPlayer2 = "" Then
MsgBox "Enter your names or start a new game."
End If
If txtPlayer1.Text = txtPlayer2.Text Then
MsgBox "Enter another name Player 1 or Player 2."
Else
lblTurn.Caption = txtPlayer1.Text
End If
End Sub
Private Sub cmdQuit_Click()
End
End Sub
Private Sub cmdRematch_Click()
Call reset
If (Val(lblWin1.Caption) + Val(lblWin2.Caption) + Val(lblTie.Caption)) Mod 2 = 1 Then
lblTurn.Caption = txtPlayer2.Text
ElseIf (Val(lblWin1.Caption) + Val(lblWin2.Caption)) Mod 2 = 0 Then
lblTurn.Caption = txtPlayer1.Text
End If
End Sub
Private Sub Form_Load()
End Sub
Private Sub Timer1_Timer()
If (lblX1.Visible = True And lblX2.Visible = True And lblX3.Visible = True) Then
Me.Line13.Visible = Not Me.Line13.Visible
ElseIf (lblX1.Visible = True And lblX4.Visible = True And lblX7.Visible = True) Then
Me.Line11.Visible = Not Me.Line11.Visible
ElseIf (lblX1.Visible = True And lblX5.Visible = True And lblX9.Visible = True) Then
Me.Line12.Visible = Not Me.Line12.Visible
ElseIf (lblX2.Visible = True And lblX5.Visible = True And lblX8.Visible = True) Then
Me.Line6.Visible = Not Me.Line6.Visible
ElseIf (lblX3.Visible = True And lblX5.Visible = True And lblX7.Visible = True) Then
Me.Line9.Visible = Not Me.Line9.Visible
ElseIf (lblX4.Visible = True And lblX5.Visible = True And lblX6.Visible = True) Then
Me.Line10.Visible = Not Me.Line10.Visible
ElseIf (lblX3.Visible = True And lblX6.Visible = True And lblX9.Visible = True) Then
Me.Line8.Visible = Not Me.Line8.Visible
ElseIf (lblX7.Visible = True And lblX8.Visible = True And lblX9.Visible = True) Then
Me.Line7.Visible = Not Me.Line7.Visible
ElseIf (lblO1.Visible = True And lblO2.Visible = True And lblO3.Visible = True) Then
Me.Line13.Visible = Not Me.Line13.Visible
ElseIf (lblO1.Visible = True And lblO4.Visible = True And lblO7.Visible = True) Then
Me.Line11.Visible = Not Me.Line11.Visible
ElseIf (lblO1.Visible = True And lblO5.Visible = True And lblO9.Visible = True) Then
Me.Line12.Visible = Not Me.Line12.Visible
ElseIf (lblO2.Visible = True And lblO5.Visible = True And lblO8.Visible = True) Then
Me.Line6.Visible = Not Me.Line6.Visible
ElseIf (lblO3.Visible = True And lblO5.Visible = True And lblO7.Visible = True) Then
Me.Line9.Visible = Not Me.Line9.Visible
ElseIf (lblO4.Visible = True And lblO5.Visible = True And lblO6.Visible = True) Then
Me.Line10.Visible = Not Me.Line10.Visible
ElseIf (lblO3.Visible = True And lblO6.Visible = True And lblO9.Visible = True) Then
Me.Line8.Visible = Not Me.Line8.Visible
ElseIf (lblO7.Visible = True And lblO8.Visible = True And lblO9.Visible = True) Then
Me.Line7.Visible = Not Me.Line7.Visible
End If
End Sub
Now you're done with the Player vs. Player part, and now let's get to the Player vs. Computer part.
First, open a form, name it "frmPvAIWelcome", and put these objects in the form:
- 1 frame
- 2 optionboxes
- 1 command button
The name of the two optionboxes are "optXorO(0)" and the "optXorO(1)", the command button should be named "cmdOK"
Change the captions in the form so that it will look like this:

This is the code for this form:
Option Explicit
Private Sub Form_Load()
CenterForm Me
End Sub
Private Sub cmdOK_Click()
gstrPlayerLetter = IIf(optXorO(0).Value, "X", "O")
gstrComputerLetter = IIf(gstrPlayerLetter = "X", "O", "X")
frmPvAI.Show
Unload Me
End Sub
Now, open up a module, name it "modPvAI and type in this code.
Option Explicit
Public gstrPlayerLetter As String * 1
Public gstrComputerLetter As String * 1
Public Sub CenterForm(objForm As Form)
With objForm
.Top = (Screen.Height - .Height) / 2
.Left = (Screen.Width - .Width) / 2
End With
End Sub
Now, open up a form, name it "frmPvAI", and put these objects in it:
- 1 textbox
- 20 labelboxes
- 2 command buttons
- 1 timer
"txtPlayer" should be the name of the textbox.
Here are the names of the labelboxes that need name changes:
- lblBox(0)
- lblBox(1)
- lblBox(2)
- lblBox(3)
- lblBox(4)
- lblBox(5)
- lblBox(6)
- lblBox(7)
- lblBox(8)
- lblWins
- lblGamesPlayed
- lblGamesWon
- lblGamesLost
- lblGamesTied
The names of the command buttons are "cmdPlayAgain" and "cmdQuit".

Here is the code for the form:
Option Explicit
Private mintGamesPlayed As Integer
Private mintGamesWon As Integer
Private mintGamesLost As Integer
Private mintGamesTied As Integer
Private mblnGameOver As Boolean
Private mintGameOutcome As Integer
Private Const mintTIE_GAME As Integer = 0
Private Const mintCOMPUTER_WINS As Integer = 1
Private Const mintPLAYER_WINS As Integer = 2
Private Sub Form_Load()
CenterForm Me
StartNewGame
End Sub
Private Sub Form_Unload(Cancel As Integer)
If MsgBox("Are you sure you want to quit?", _
vbYesNo + vbQuestion, _
"Quit Tic Tac Toe") = vbNo Then
Cancel = 1
End If
End Sub
Private Sub Label4_Click()
End Sub
Private Sub lblBox_Click(Index As Integer)
If mblnGameOver Then Exit Sub
If lblBox(Index).Caption <> "" Then Exit Sub
lblBox(Index).Caption = gstrPlayerLetter
Select Case True
Case PlayerWins(0, 1, 2): GameOver mintPLAYER_WINS, 0
Case PlayerWins(0, 4, 8): GameOver mintPLAYER_WINS, 1
Case PlayerWins(0, 3, 6): GameOver mintPLAYER_WINS, 2
Case PlayerWins(1, 4, 7): GameOver mintPLAYER_WINS, 3
Case PlayerWins(2, 5, 8): GameOver mintPLAYER_WINS, 4
Case PlayerWins(2, 4, 6): GameOver mintPLAYER_WINS, 5
Case PlayerWins(3, 4, 5): GameOver mintPLAYER_WINS, 6
Case PlayerWins(6, 7, 8): GameOver mintPLAYER_WINS, 7
Case Else: If Not TieGame Then TakeComputerTurn
End Select
End Sub
Private Sub cmdPlayAgain_Click()
StartNewGame
End Sub
Private Sub cmdQuit_Click()
Unload Me
End Sub
Private Sub lblGamesWon_Click()
End Sub
Private Sub tmrFlash_Timer()
lblWins.Visible = Not lblWins.Visible
End Sub
Private Sub StartNewGame()
Dim intX As Integer
Dim intGoesFirst As Integer
mblnGameOver = False
For intX = 0 To 8
lblBox(intX).Caption = ""
Next
For intX = 0 To 7
linWin(intX).Visible = False
Next
tmrFlash.Enabled = False
lblWins.Visible = False
cmdPlayAgain.Enabled = False
Randomize
intGoesFirst = Int(2 * Rnd + 1)
If intGoesFirst = 1 Then
MsgBox "I will go first this time.", , "New Game"
TakeComputerTurn
Else
MsgBox "You go first this time.", , "New Game"
End If
End Sub
Private Sub TakeComputerTurn()
Dim intX As Integer
Dim blnMoveMade As Boolean
If ComputerWins(0, 1, 2) Then GameOver mintCOMPUTER_WINS, 0: Exit Sub
If ComputerWins(0, 4, 8) Then GameOver mintCOMPUTER_WINS, 1: Exit Sub
If ComputerWins(0, 3, 6) Then GameOver mintCOMPUTER_WINS, 2: Exit Sub
If ComputerWins(1, 4, 7) Then GameOver mintCOMPUTER_WINS, 3: Exit Sub
If ComputerWins(2, 5, 8) Then GameOver mintCOMPUTER_WINS, 4: Exit Sub
If ComputerWins(2, 4, 6) Then GameOver mintCOMPUTER_WINS, 5: Exit Sub
If ComputerWins(3, 4, 5) Then GameOver mintCOMPUTER_WINS, 6: Exit Sub
If ComputerWins(6, 7, 8) Then GameOver mintCOMPUTER_WINS, 7: Exit Sub
If ComputerBlocks(0, 1, 2) Then TieGame: Exit Sub
If ComputerBlocks(0, 4, 8) Then TieGame: Exit Sub
If ComputerBlocks(0, 3, 6) Then TieGame: Exit Sub
If ComputerBlocks(1, 4, 7) Then TieGame: Exit Sub
If ComputerBlocks(2, 5, 8) Then TieGame: Exit Sub
If ComputerBlocks(2, 4, 6) Then TieGame: Exit Sub
If ComputerBlocks(3, 4, 5) Then TieGame: Exit Sub
If ComputerBlocks(6, 7, 8) Then TieGame: Exit Sub
blnMoveMade = True
If lblBox(4).Caption = "" Then
lblBox(4).Caption = gstrComputerLetter
ElseIf lblBox(0).Caption = "" Then
lblBox(0).Caption = gstrComputerLetter
ElseIf lblBox(2).Caption = "" Then
lblBox(2).Caption = gstrComputerLetter
ElseIf lblBox(6).Caption = "" Then
lblBox(6).Caption = gstrComputerLetter
ElseIf lblBox(8).Caption = "" Then
lblBox(8).Caption = gstrComputerLetter
ElseIf lblBox(4).Caption = gstrComputerLetter Then
If lblBox(3).Caption = "" Then
lblBox(3).Caption = gstrComputerLetter
ElseIf lblBox(5).Caption = "" Then
lblBox(5).Caption = gstrComputerLetter
Else
blnMoveMade = False
End If
Else
blnMoveMade = False
End If
If Not blnMoveMade Then
For intX = 0 To 8
If lblBox(intX).Caption = "" Then
lblBox(intX).Caption = gstrComputerLetter
Exit For
End If
Next
End If
TieGame
End Sub
Private Function PlayerWins(pintPos1 As Integer, _
pintPos2 As Integer, _
pintPos3 As Integer) _
As Boolean
If lblBox(pintPos1).Caption = "" _
Or lblBox(pintPos2).Caption = "" _
Or lblBox(pintPos3).Caption = "" Then
PlayerWins = False
Else
If lblBox(pintPos1).Caption = lblBox(pintPos2).Caption _
And lblBox(pintPos1).Caption = lblBox(pintPos3).Caption Then
PlayerWins = True
Else
PlayerWins = False
End If
End If
End Function
Private Function ComputerWins(pintPos1 As Integer, _
pintPos2 As Integer, _
pintPos3 As Integer) _
As Boolean
If lblBox(pintPos1).Caption = "" _
And lblBox(pintPos2).Caption = gstrComputerLetter _
And lblBox(pintPos3).Caption = gstrComputerLetter Then
lblBox(pintPos1).Caption = gstrComputerLetter
ComputerWins = True
Exit Function
End If
If lblBox(pintPos1).Caption = gstrComputerLetter _
And lblBox(pintPos2).Caption = "" _
And lblBox(pintPos3).Caption = gstrComputerLetter Then
lblBox(pintPos2).Caption = gstrComputerLetter
ComputerWins = True
Exit Function
End If
If lblBox(pintPos1).Caption = gstrComputerLetter _
And lblBox(pintPos2).Caption = gstrComputerLetter _
And lblBox(pintPos3).Caption = "" Then
lblBox(pintPos3).Caption = gstrComputerLetter
ComputerWins = True
Exit Function
End If
ComputerWins = False
End Function
Private Function ComputerBlocks(pintPos1 As Integer, _
pintPos2 As Integer, _
pintPos3 As Integer) _
As Boolean
If lblBox(pintPos1).Caption = "" _
And lblBox(pintPos2).Caption = gstrPlayerLetter _
And lblBox(pintPos3).Caption = gstrPlayerLetter Then
lblBox(pintPos1).Caption = gstrComputerLetter
ComputerBlocks = True
Exit Function
End If
If lblBox(pintPos1).Caption = gstrPlayerLetter _
And lblBox(pintPos2).Caption = "" _
And lblBox(pintPos3).Caption = gstrPlayerLetter Then
lblBox(pintPos2).Caption = gstrComputerLetter
ComputerBlocks = True
Exit Function
End If
If lblBox(pintPos1).Caption = gstrPlayerLetter _
And lblBox(pintPos2).Caption = gstrPlayerLetter _
And lblBox(pintPos3).Caption = "" Then
lblBox(pintPos3).Caption = gstrComputerLetter
ComputerBlocks = True
Exit Function
End If
ComputerBlocks = False
End Function
Private Function TieGame() As Boolean
Dim intX As Integer
For intX = 0 To 8
If lblBox(intX).Caption = "" Then
TieGame = False
Exit Function
End If
Next
TieGame = True
GameOver mintTIE_GAME
End Function
Private Sub GameOver(pintGameOutcome As Integer, _
Optional pintLineIndex As Integer)
Dim strOutcomeMsg As String
If pintGameOutcome = mintTIE_GAME Then
strOutcomeMsg = "IT'S A TIE !!!"
mintGamesTied = mintGamesTied + 1
Else
linWin(pintLineIndex).Visible = True
If pintGameOutcome = mintCOMPUTER_WINS Then
strOutcomeMsg = "YOU LOSE !!!"
mintGamesLost = mintGamesLost + 1
Else
strOutcomeMsg = "YOU WIN !!!"
mintGamesWon = mintGamesWon + 1
End If
End If
lblWins.Caption = strOutcomeMsg
lblWins.Visible = True
tmrFlash.Enabled = True
cmdPlayAgain.Enabled = True
mintGamesPlayed = mintGamesPlayed + 1
lblGamesPlayed.Caption = CStr(mintGamesPlayed)
lblGamesWon.Caption = CStr(mintGamesWon)
lblGamesLost.Caption = CStr(mintGamesLost)
lblGamesTied.Caption = CStr(mintGamesTied)
mblnGameOver = True
End Sub
And Now you're done. So go on and play someone or play the computer if you're good enough.
Points of Interest
The Artificial intelligence was insanely hard and I needed to make it for a school project. My version was the best tic-tac-toe game in my class so far and now you guys can do this as well.
History
Keep a running update of any changes or improvements you've made here.