Introduction
WCF is built to support interoperability. Being just a .NET developer, it becomes very difficult to prove this concept. I created this POC with the help of one of my friends Jay D Khambholiya who is a Java developer.
This article will talk about how a WCF service can be consumed by a Java client application in following three steps:
-
Create a WCF Service
-
Host WCf Service in IIS
-
Consume WCF Service in Java Client
Step 1: Create WCF Service
- Open Visual Studio 2012 > File Menu > click New Project… > select Class Library – CalculatorService > click OK
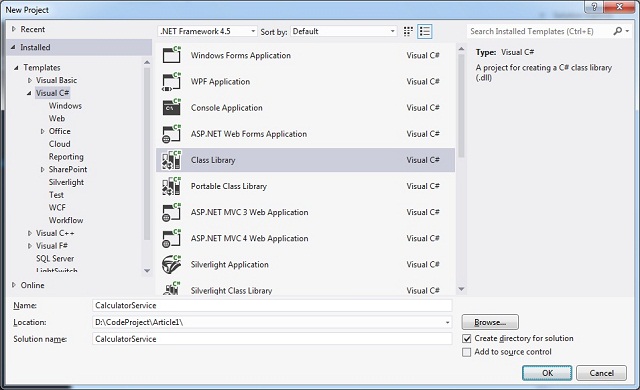
- Delete the class - Class1.cs file that is auto-generated.
- Right-click on CalculatorService project > Add > click New Item > select WCF Service > provide Name as CalculatorService > click Add button:

- This will add the required assembly references (like, System.ServiceModel) along with a Service Contract Interface – ICalculatorService.cs and Service class – CalculatorService.cs
- Modify ICalculatorService.cs as follows:
using System.ServiceModel;
namespace CalculatorService
{
[ServiceContract]
public interface ICalculatorService
{
[OperationContract]
int Add(int num1, int num2);
}
}
- Modify CalculatorService.cs as follows:
namespace CalculatorService
{
public class CalculatorService : ICalculatorService
{
public int Add(int num1, int num2)
{
return num1 + num2;
}
}
}
- Build the solution by pressing [CTRL]+[SHIFT]+b or go to BUILD menu > click Build Solution option.
Step 2: Host WCF Service in IIS
- Add a new web site to host Calculator Service in IIS.
- Go to File Menu > Add > click New Web Site… > select WCF Service > provide a suitable name – CalculatorServiceIISHost > click OK:

- A new web site will be added with a service class - Service.cs and an interface - IService.cs within App_Code folder along with a Service.svc file. Since the service is already created, delete the auto created service class (Service.cs) and the interface (IService.cs) from App_Code folder.
- Right-click on CalculatorServiceIISHost project > click Add Reference > select CalculatorService > click OK:

- Open Service.svc file > Delete CodeBehind property:
<%@ ServiceHost Language="C#" Debug="true" Service="Service" CodeBehind="~/App_Code/Service.cs" %>
- Modify Service property as shown below:
<%@ ServiceHost Language="C#" Debug="true" Service="CalculatorService.CalculatorService" %>
- Modify web.config file to have the following settings:
<system.serviceModel>
<services>
<service name="CalculatorService.CalculatorService"
behaviorConfiguration="mex">
<endpoint address="CalculatorService" binding="basicHttpBinding"
contract="CalculatorService.ICalculatorService"></endpoint>
<host>
<baseAddresses>
<add baseAddress="http://localhost:8080/"/>
</baseAddresses></host>
</service>
</services>
<behaviors>
<serviceBehaviors>
<behavior name="mex">
<serviceMetadata httpGetBinding="true"/>
</behavior>
</serviceBehaviors>
</behaviors>
</system.serviceModel>
- Now the service is ready to be hosted in IIS.
- Open IIS > right-click on Default Web Site > click Add Application… > enter Alias name – calculator > select the Physical path of the service > click OK:

- Enable Anonymous Authentication as shown below:

- Go to Content View > right-click Service.svc > click Browse:

Step 3: Consume WCF Service in Java client
- Tools Used: Eclipse Indigo, Apache Tomcat 6, Apache Axis (comes pre-installed in Eclipse Indigo)
- Create a new Dynamic Web Project in Eclipse:

- To create a WS Client, select New > Web Services > select Web Service Client > click Next button:

- Enter the WSDL path of the WCF Service > click Finish button:

- This should auto-generate the code for the WS proxy:

- Create a simple JSP page that has two text boxes and a servlet to call the “add” method via web service:
- Write the following code in WCFServlet’s doPost() method to call web service’s “add” method:
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException
{
Integer input1=Integer.parseInt(request.getParameter("input1"));
Integer input2=Integer.parseInt(request.getParameter("input2"));
ICalculatorServiceProxy calService=new ICalculatorServiceProxy();
Integer result=calService.add(input1,input2);
request.setAttribute("res", "The result is: "+result);
RequestDispatcher rd=request.getRequestDispatcher("WSDemo.jsp");
rd.forward(request, response);
}
- Display the result on the same page WSDemo.jsp:

Summary
This article can be referred by any project or individual to get a step-by-step guidance on how a WCF service can be created and hosted in IIS and then create a Java client application to consume the service.
History
Keep a running update of any changes or improvements you've made here.
I'm Debabrata Das, also known as DD. I started working as a FoxPro 2.6 developer then there is a list of different technologies/languages C, C++, VB 6.0, Classic ASP, COM, DCOM, ASP.NET, ASP.NET MVC, C#, HTML, CSS, JavaScript, jQuery, SQL Server, Oracle, No-SQL, Node.Js, ReactJS, etc.
I believe in "the best way to learn is to teach". Passionate about finding a more efficient solution of any given problem.