Model binder maps HTML form elements to the model. It acts like a bridge between HTML UI and MVC model.
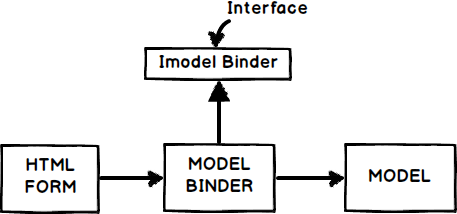
Take the below simple HTML form example:
<formid="frm1" method=post action="/Customer/SubmitCustomer">
Customer code :- <inputname="CCode"type="text"/>
Customer name :- <inputname="CName"type="text"/>
<input type=submit/>
</form>
Now this form needs to fill the below “Customer
” class model. If you see the HTML control name, it is different from the class property name. For example, HTML textbox control name is “CCode
” and the class property name is “CustomerCode
”. This mapping code is written in HTML binder classes.
publicclassCustomer
{
publicstring CustomerCode { get; set; }
publicstring CustomerName { get; set; }
}
To create a model binder, we need to implement “IModelBinder
” interface and mapping code needs to be written in the “BindModel
” method as shown in the below code.
publicclassCustomerBinder : IModelBinder
{
publicobject BindModel(ControllerContext controllerContext, ModelBindingContext bindingContext)
{
HttpRequestBase request = controllerContext.HttpContext.Request;
string strCustomerCode = request.Form.Get("CCode");
string strCustomerName = request.Form.Get("CName");
returnnewCustomer
{
CustomerCode = strCustomerCode,
CustomerName = strCustomerName
};
}
}
Now in the action result method, we need to use the “ModelBinder
” attribute which will attach the binder with the class model.
publicActionResult SubmitCustomer([ModelBinder(typeof(CustomerBinder))]Customer obj)
{
return View("DisplayCustomer");
}
If you are completely new to ASP.NET MVC, you can start with the free youtube video below which teaches MVC 5 from scratch.

For further reading do watch the below interview preparation videos and step by step video series.