Screen Shots
DataGrid
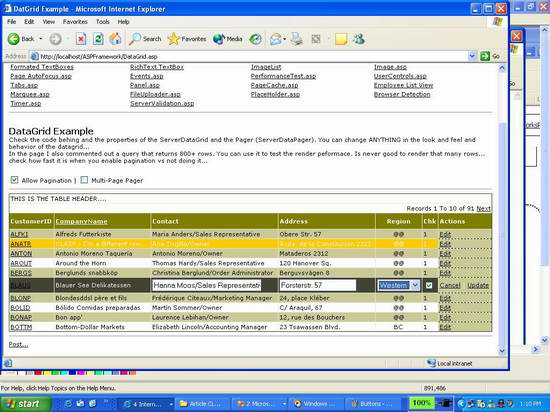
Buttons

Debug/Tracing

DropDown/List

RichTextBox

Tabs

TextBoxes

Introduction
This is CLASP version 2.0 and a follow-up article for
Classic ASP Framework
The Classic ASP Framework allows you to structure your ASP pages in the same
way you would do it in ASP.NET. It is fully event driven and has most of the
controls you will ever need such as TexBoxes, CheckBoxes (and CheckBoxList),
RadioButtons, Labels, DropDowns, Lists, DataGrids, DataRepeaters, DataLists,
RichTextBoxes, Timers, Field Validators, Tabs and many more!
ViewState
support (can be be overridden at the page level):
- Client Side: in a hidden field.
- Server Side: in a Session variable.
- SQL Server: in a SQL Server table.
-
Custom: Write your own Viewstate perisistence handler!
- Different implementations: COM (fastest) and pure ASP (a bit slower). Server
Side and SQL Server don't require COM implementations.
Ability to register client side scripts and to register client side events from
the server code.
For instance. Page.RegisterEventListener cmdSave,"onclick","TestFnc"
. All
events are stacked/linked
and are called in a bubble like fashion, so you can have multiple event
listeners for the same object!!!.
Other nice things are a PageController
concept which allows you to structure
your pages in a template-like fashion that supports role-level authentication
and authorization.
What's new in version 2.0?
Too many things have changed and been improved to
mention in this article. A few things are listed below:
- Event model matches as much as possible to ASP.NET (Page level and Control
life-cycle level)
- Improved Speed - Big time.
- Improvements to all controls. More properties and customization.
- New controls such as RichTextBox, Timer, Panel, Validation Controls (just like
in ASP.NET)
-
More configuration options such as pluggable viewstate persistence and
implementations just to name a few.
- More samples.
- A sample site with help.
- The PageController model to build template pages with support for role-level
authentication/authorization.
- Reusable JavaScript libraries (clasp, clasp.form, clasp.events,
clasp.validation)
- etc etc etc
Do you want to see it in action? visit our
samples site.
Background
CLASP is ideal for developers that need maintain or create new ASP Pages. It
will simplify your coding and make it a breeze to create new and maintain pages
and when it is time to move to ASP.NET, it will take you a fraction of the time
to port since the coding style is almost the same.
The main benefits are:
- Coding takes a lot less
time, code is more readable and easier to maintain.
- Comprenhensive list of controls.
- Flexible event driven model (Similar to AutoEventWireUp in ASP.NET)
- Source code for EVERYTHING.
-
Is Open Source --- FREE ---
- Simplifies the migration to ASP.NET.
Using the code
Using the code couldn't be any easier.
Structure of a Page
CLASP pages are structured in the following way (unless you use the page
controller):
-
Include the WebControl.asp page
-
Include any WebControl file to be used by the page (i.e. Server_TextBox.asp)
-
Include any extra file that your page will make use of.
-
Execute the following ASP Code:
Page.Execute
-
Write your HTML. You can mix html with server controls (i.e.
<TD><%txtFirstName%>
</TD>
) You MUST start the section where you will be
rendering your HTML Form and where WebControls that render HTML input controls
with this ASP Code: Page.OpenForm
and end it with
Page.CloseForm
.
This is critical these functions render the html form and include all the
hidden fields that CLASP make use of.
-
Your Code-Behind or Server Code is next and could be in a separate file or
at the bottom of the page.
-
Include your WebControl variable declarations (i.e.
Dim txtFirstName,
txtLastName
)
-
Include your page-level variables (i.e.
Dim mStatus
)
-
Add
Public Function Page_Init()
and initialize all the WebControls there (I.e.
Set txtFirstName = New_ServerTextBox("txtFirstName")
and module level
variables (i.e. mStatus= 0
)
-
Add any other Page event that you want override such as
Page_Controls_Init
(happens only once and is ideal to initialize controls that have the
viewstate enabled, code in this event will be executed only If Not Page.IsPostBack
) ,
Page_Load
, Page_LoadViewState
, etc. Note: Keep in mind
that viewstate is available only from Page_LoadViewState and any later event.
-
Write your event handlers such as
cmdSave_OnClick
,
txtFirstName_OnTextChange
.
-
Write your supporting functions.
-
That is it!, you are done!.
Sequence of Events in CLASP
Looking at the Page.Execute -"Sub Main()"
- routine in WebControl.asp will
give you a very good idea of how CLASP process each request. A high level
look at the life cycle of a CLASP page looks like this (you can override and
handle the events highlighted in blue):
-
Page_Authenticate_Request
-
Page_Authorize_Request
-
Page_Init
-
If
Not Page.IsPostBack Then Page_Controls_Init
-
If
Page.IsPostBack Then Page_LoadViewState
-
If
Page.IsPostBack Then ProcessPostBackData
-
Page_Load
-
If
Page.IstPostBack Then Page.HandleServerEvent "RaiseChangedEvents"
-
If
Page.IsPostBack Then Page.HandleClientEvent
(Handles the PostBack by executing
the handler of the event)
-
Page_PreRender
-
Page_SaveViewState
-
Page_Terminate
Note : If you use the
PageControler
model you also have the following
events:
Page_Configure
,
Page_RenderHeader
,
Page_RendeForm
,
Page_RenderFooter
and it will handle the
Page_AuthenticateRequest
and
Page_AuthorizeRequest
automatically. The CLASP sample site has an example
of an implementation using the page controller.
The Life Cycle of a Control is:
(this happens automatically and is transparent)
-
Class_Initialize
-
OnInit
- You need to subscribe to receive this
event -
-
ReadProperties(v)
- Only if EnableViewState = True
-
OnLoad
- You need to subscribe to receive this event -
-
ProcessPostBack
- You need to subscribe
to receive this event
-
-
HandleClientEvent(e)
-Only if Target of the postback event
-
WriteProperties(v)
-Only
if EnableViewState = True
-
Render
-
Class_Terminate
Here is a code sample. For those of you that are already programming in ASP.NET
you will find the the code looks very much like an ASP.NET page (with some
obvious differences...).
<!--#Include File = "..\WebControl.asp" -->
<!--#Include File = "..\Server_Button.asp" -->
<!--#Include File = "..\Server_CheckBox.asp" -->
<!--#Include File = "..\Server_DropDown.asp" -->
<!--#Include File = "..\Server_Label.asp" -->
<!--#Include File = "DBWrapper.asp" -->
<HTML>
<HEAD>
<TITLE>Samples</TITLE>
<LINK rel="stylesheet" type="text/css" href="Samples.css">
</HEAD>
<BODY>
<!--#Include File = "Home.asp" -->
<%Page.Execute%>
<Span Class="Caption">DROPDOWN EXAMPLES</Span>
<%Page.OpenForm%>
<%lblMessage%><HR>
<%chkHideShow%> | <%chkAutoPostBack%> | <%chkListBox%><HR>
<%cboDropDown%>
<HR>
<%cmdAdd%> | <%cmdRemove%>
<%Page.CloseForm%>
</BODY>
</HTML>
<%
Dim lblMessage
Dim cmdAdd
Dim cmdRemove
Dim chkHideShow
Dim chkAutoPostBack
Dim chkListBox
Dim cboDropDown
Public Function Page_Init()
Page.DebugEnabled = False
Set lblMessage = New_ServerLabel("lblMessage")
Set cmdAdd = New_ServerLinkButton("cmdAdd")
Set cmdRemove = New_ServerLinkButton("cmdRemove")
Set chkHideShow = New_ServerCheckBox("chkHideShow")
Set chkAutoPostBack = New_ServerCheckBox("chkAutoPostBack")
Set chkListBox= New_ServerCheckBox("chkListBox")
Set cboDropDown = New_ServerDropDown("cboDropDown")
End Function
Public Function Page_Controls_Init()
cmdAdd.Text = "Add"
cmdRemove.Text = "Remove"
lblMessage.Control.Style = "border:1px solid blue;
background-color:#EEEEEE;width:100%;font-size:8pt"
lblMessage.Text = "This is an Example"
chkHideShow.Caption = "Hide/Show List"
chkHideShow.AutoPostBack = True
chkAutoPostBack.Caption = "DropDown AutoPostBack"
chkAutoPostBack.AutoPostBack=True
chkListBox.Caption = "Make it a list box"
chkListBox.AutoPostBack = True
cboDropDown.DataTextField = "TerritoryDescription"
cboDropDown.DataValueField = "TerritoryID"
Set cboDropDown.DataSource = GetRecordset(
"SELECT TerritoryID,TerritoryDescription FROM Territories ORDER BY 2")
cboDropDown.DataBind()
Set cboDropDown.DataSource = Nothing
cboDropDown.Caption = "Territory:"
cboDropDown.CaptionCssClass = "InputCaption"
End Function
Public Function Page_PreRender()
Dim x,mx
Dim msg
Set msg = New StringBuilder
msg.Append "<B>Selected Value=</B>"
& cboDropDown.Items.GetSelectedText & "<BR>"
msg.Append "<B>Selected Text=</B>"
& cboDropDown.Items.GetSelectedValue & "<BR>"
msg.Append "<HR>"
mx = cboDropDown.Items.Count -1
lblMessage.Text = msg.ToString()
End Function
Public Function chkHideShow_Click()
cboDropDown.Control.Visible = Not cboDropDown.Control.Visible
End Function
Public Function chkAutoPostBack_Click()
cboDropDown.AutoPostBack = chkAutoPostBack.Checked
End Function
Public Function cmdAdd_OnClick()
cboDropDown.Items.Add cboDropDown.Items.Count,
cboDropDown.Items.Count,False
End Function
Public Function cmdRemove_OnClick()
cboDropDown.Items.Remove cboDropDown.Items.Count-1
End Function
Public Function chkListBox_Click()
if chkListBox.Checked Then
cboDropDown.Rows = 10
cboDropDown.Multiple = True
Else
cboDropDown.Rows = 1
cboDropDown.Multiple = False
End If
End Function
%>
Points of Interest
History
- The current version is 2.0 (production stable)
Christian is a Microsoft Certified Professional 8+ years of experience in the consulting business. He has designed and developed numerous Web and Windows applications (and many other things he doesn't even remember...) using technologies and tools such as ASP.NET,VB.NET, C#, ASP, COM+/MTS, C++, JavaScript, HTML/DHTML, Visual Basic, SQL Server, Oracle, Microsoft Access, etc.
He is quite "curious" and always wants to know how things work and the "why was it done like this or that, how does this works...", and because of this he is always pursuing to improve his skills so he can know the answers to these questions.
He also likes Martial Arts, finishing and spending time with his wife and daughter. And why not?, playing XBOX from time to time :-P
Christian works as the Microsoft Technologies Manager/Senior Developer for Electronic Knowledge Interchange, a Chicago based Technology Consulting Firm.
http://www.eki-consulting.com