Recently, we faced a situation in which we need to round up a number to the greatest 10s place.
For example: we needed to round up a number as follows:
- 1023 -> 2000
- 37911 -> 40000
- 912345 -> 1000000
First, we checked Math.Round(). But it didn’t solve our problem. Math.Round()
supports only rounding a value to the nearest integer or to the specified number of fractional digits. On further checking, we come up with a solution by using Math.Ceiling() method.
Math.Ceiling()
returns the smallest integral value that is greater than or equal to the specified double-precision floating-point number.
For example:
Math.Ceiling(7.3)
-> 8 Math.Ceiling(7.64)
-> 8 Math.Ceiling(0.12)
-> 1
Below is the solution we came up with:
decimal[] values = { 1023, 37911, 23000, 1234, 912345 };
foreach (var value in values) {
var length = value.ToString().Length - 1;
var power = Math.Pow(10, (double)length);
var roundadValue = Math.Ceiling(value / (decimal)power) * (decimal)power;
Console.WriteLine("Value:{0} Rounded Value:{1}", value, roundadValue);
}
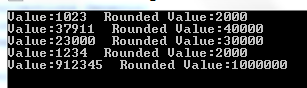
The Logic
Math.Ceiling(value / (decimal)power) * (decimal)power;
var roundadValue = Math.Ceiling(1023 / 1000) * 1000;
So, for any number, first calculate the power of 10s and then use it for the above calculation to get the results.